Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial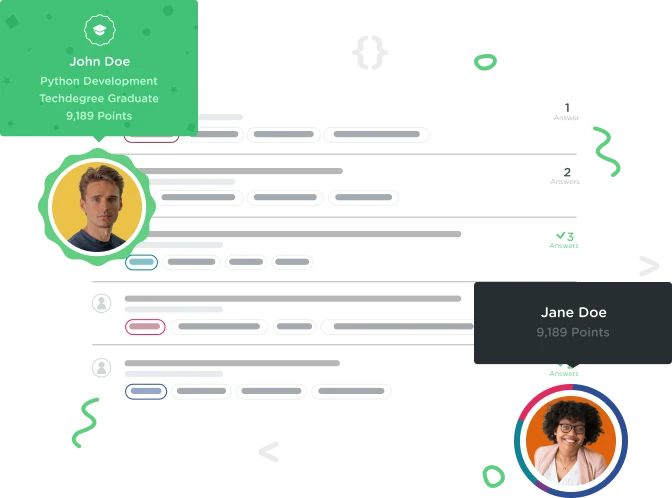

shawn khah
15,082 Pointshelp plz
Challenge Task 1 of 2
Code the AddWord method such that, WordCount will contain the number of times the AddWord method has been called with a given word. For example, if AddWord is called twice with the word "rock" then WordCount["rock"] will be 2. Bummer! An item with the same key has already been added. Restart Preview Get Help Recheck work LexicalAnalysis.cs
1 1 using System.Collections.Generic; 2 3 namespace Treehouse.CodeChallenges 4 { 5 public class LexicalAnalysis 6 { 7 public Dictionary<string, int> WordCount = new Dictionary<string, int>(); 8 9 public void AddWord(string word) 10 { 11 WordCount.Add(word, (WordCount.Count +1)); 12 } 13 } 14 } 15
using System.Collections.Generic;
namespace Treehouse.CodeChallenges
{
public class LexicalAnalysis
{
public Dictionary<string, int> WordCount = new Dictionary<string, int>();
public void AddWord(string word)
{
WordCount.Add(word, (WordCount.Count +1));
}
}
}
6 Answers

Kevin Hatch
12,487 PointsHi Shawn,
So this one was quite confusing based on the actual question itself:
"Code the AddWord method such that, WordCount will contain the number of times the AddWord method has been called with a given word."
What it is actually requires is what Steven posted.
- you'll need to test if the word is already in the dictionary
- if it is, you will add 1 to the count that it already has
- if it is not, you will add it with a count of 1
So the first step is to go through the methods listed in the Dictionary Class to find one that will check IF the "Key" is already in the dictionary; in this case the "Key" is the variable "word" that is being passed. A method that will accomplish this nicely is the "ContainsKey" method. Since this method returns a true or false, a simple if else statement can be used to accomplish the next to two steps.
Remember that as in Arrays, when you need to get a value from the array you can type, sampleVar = sampleArray[samplePosition]
Or to set it, sampleArray[samplePosition] = sampleVar
So to increase the "Value" by 1, the code would be or with some syntactic sugar....
exampleDictionary[examplePosition] = exampleDictionary[examplePosition] + 1;
exampleDictionary[examplePosition] += 1;
exampleDictionary[examplePosition] ++;
A simple ++ will work just fine.
As for the ELSE part of the condition, search the Dictionary Class methods again to find the "Add" method that will, as it implies, add to the current Dictionary. You will need to pass the "word" variable as the "Key" and the integer "1" for the "Value"; (Key, Value) = (word, 1).
Code Sample:
public void AddWord(string word)
{
if (WordCount.ContainsKey(word))
{
WordCount[word]++;
}
else
{
WordCount.Add(word, 1);
}
}
I hope this helps.
Resources:

Kevin Gates
15,053 PointsThis also works:
using System.Collections.Generic;
using Newtonsoft.Json.Linq;
namespace Treehouse.CodeChallenges
{
public class LexicalAnalysis
{
public Dictionary<string, int> WordCount = new Dictionary<string, int>();
public void AddWord(string word)
{
try
{
WordCount.Add(word, 1);
}
catch
{
WordCount[word] +=1;
}
}
}
}

Erin Claudio
Courses Plus Student 8,403 PointsNice solution
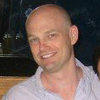
Noah Yasskin
23,947 PointsThe brevity of this question was confusing. Here's Katrin's clever code solution formatted pretty.
using System.Collections.Generic;
namespace Treehouse.CodeChallenges
{
public class LexicalAnalysis
{
public Dictionary<string, int> WordCount = new Dictionary<string, int>();
public void AddWord(string word)
{
bool check = false;
foreach (string entry in WordCount.Keys)
{
if (entry == word)
{
check = true;
break;
}
}
if (check) {
WordCount[word] += 1;
} else {
WordCount.Add(word, 1);
}
}
}
}
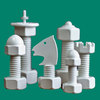
Steven Parker
231,269 PointsHere are a few hints:
- you'll need to test if the word is already in the dictionary
- if it is not, you will add it with a count of 1
- if it is, you will add 1 to the count that it already has
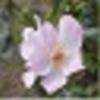
Katrin Nitsche
23,462 PointsI tried to solve in one line which didn't work. So I added all the checks and solved it with this code:
public void AddWord(string word) { bool check = false; foreach (string entry in WordCount.Keys) { if (entry == word) { check = true; break; } }
if (check) { WordCount[word] += 1; } else { WordCount.Add(word, 1); } }

Anne Yeiser
19,291 PointsI am sorry, I have again worked on this a long time, but still need your help. Thanks!!!
Anne Yeiser
19,291 PointsAnne Yeiser
19,291 Pointsthank you!