Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial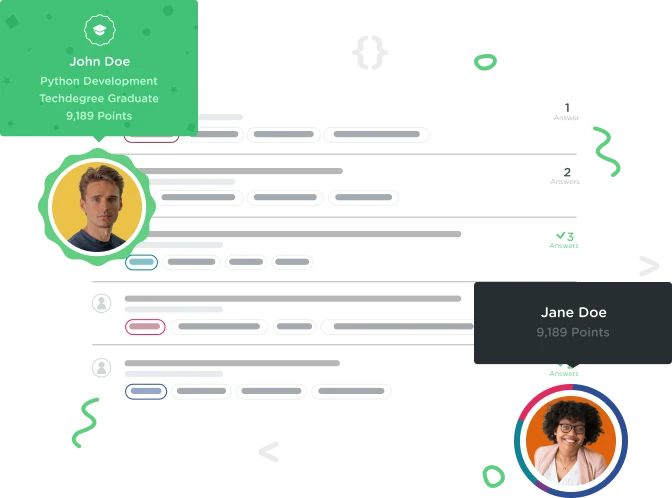

Brett Carter
Full Stack JavaScript Techdegree Graduate 17,766 PointsHelp setting variable to dynamic input field.
I'm working on the Full Stack Javascript Project 2 - Pagination Filter and have run into a problem.
In attempting to build the search function, I've run into a problem. If the search input is built directly into the index.html doc, my "Search" function runs great. For some reason when I add the search input to the html dynamically with javascript, my variable for the value of the search field throws an error of "undefined".
Here's how I create the search input on the page:
$(document).ready(function(){
$('.page-header').append('<div class ="studentSearch"><input type="text" id="searchField" placeholder="Search for a student"><button id="searchButton">SEARCH</button></div>')
});
Here's the search method which sets the variable 'studentSearch' to the value of '.studentSearch' when the appropriate button is clicked.
PLEASE NOTE that the searchField variable is declared globally at the beginning of the script with 'let searchField;'
// search function
var search = function(){
let filter, ul, li, a, i;
searchField = $('#searchField').value;
filter = searchField.toUpperCase(); /* the error is thrown on this line and reads 'cannot read property "toUpperCase" of undefined' */
ul = $('.student-list');
li = $(ul).find('li');
results = [];
// Loop through all list items, and hide those who don't match the search query
for (i = 0; i < li.length; i++) {
//variables to get student name text
a = $(li[i]).find('h3')[0];
b = $(a).text();
c = b.toUpperCase().indexOf(filter);
//variables to get student email text
d = $(li[i]).find('span')[0];
e = $(d).text();
f = e.toUpperCase().indexOf(filter);
//variable s set as student object being checked for search match
s = li[i];
//if s is a match and less
if (c > -1 || f > -1) {
$(results).push(s);
}
}
$(students).hide();
showTen(results,0,9);
if (results.length <= 0){
$('.student-list').append('<h3 class="no-results">No results have been found. Please try a new search.</h3>');
} else {
$('.no-results').remove(); //clears no results text in case it was there
}
};
//call search function on 'search' button click
$('div.page-header').on('click','#searchButton',search);
As previously mentioned, I've had this search function working properly with the same code when the search field is added as an element directly to index.html.
I can bind events to the input, but setting a darn variable to the value of the input has me stumped!

Brett Carter
Full Stack JavaScript Techdegree Graduate 17,766 PointsI was able to fix the by setting the input value to the variable to the button click event instead of in the search function (which was called with the same button click). In other words, I changed this:
//call search function on 'search' button click
$('div.page-header').on('click','#searchButton',search);
to this
//call search function on 'search' button click
$('div.page-header').on('click','#searchButton',function(){
searchField = $('#searchField').val();
search();
});
Still curious why this fixes the issue.
2 Answers
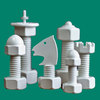
Steven Parker
231,271 PointsIt's not where you set the value of "searchField", but how. The original function had this line:
searchField = $('#searchField').value;
But I don't think jQuery objects have a "value" property. So this would explain why on the next line "searchField" had an undefined. When you moved the line, you also modified it to call the "val()" method of the jQuery object, which fixed it.

Brett Carter
Full Stack JavaScript Techdegree Graduate 17,766 PointsSteven Parker . Yes, defining the variable in 'search' function works with .val().
I had tried .val() with no success in the past, but I believe I was using .toUpperCase on the variable the line BEFORE the variable was declared.
Also, I was not aware that jquery objects could not access the .value property. Does this mean that all jquery objects don't have any default javascript properties? .length works fine on a jquery object. Curious what the difference is.
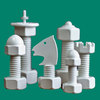
Steven Parker
231,271 PointsDespite having a large number of methods, jQuery objects have very few properties other than length.
On the other hand, input elements have several properties (including value) plus they inherit even more from ancestors such as Element and Node.
Happy coding!

Brett Carter
Full Stack JavaScript Techdegree Graduate 17,766 PointsGot it. Thanks for the info Steven Parker
Greg Kaleka
39,021 PointsGreg Kaleka
39,021 PointsWould be great if you could add your whole project to a workspace and take a snapshot. Or put the necessary code into a jsfiddle or codepen page. It's pretty hard to debug without being able to play around with stuff.