Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial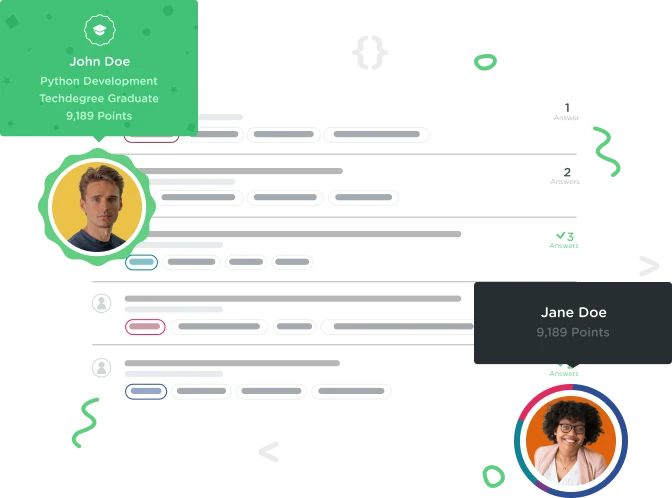
Jessica Stout
4,828 PointsHELP! This for loop is confusing me!
I am lost as to how to list the random number from 4-156 using a for loop. What am I missing? Should I add another variable? I'm so stuck! Any help would be GREATLY appreciated! Thank you!!!!
for (var i=1; i<157; i+=1) {
}
console.log(i);
4 Answers
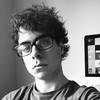
Zach Allan
19,452 PointsFirstly, you need to put console.log(i) inside your for loop. Like this:
for (var i=1; i<157; i+=1) {
console.log(i);
}
var i=1
is your initializer. It sets i
(the index) to be 1.
i<157
is your condition. The loop keeps going until that condition is no longer true.
i+=1
is your increment. It keeps adding 1 to the previous number.
The for loop will break once it has reached 157. As soon as i
gets to 157 then the condition is no longer true and the console.log will no longer get executed. So basically your loop will print out the numbers 1-156.
Hope that helps! :)
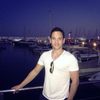
Jayden Spring
8,625 PointsThis look is not generating a random number simply iterating through I until its value is less than 157. Also to see an output you need the console log inside the for statement.
To generate random number between 0-157 use something like this; Math.floor(Math.Random() * 157)
Jayden
Jessica Stout
4,828 PointsOH! I stated the task incorrectly- I'm sorry. I meant I need to log all the numbers from 4 to 156 in the console. Using the for loop.
What component am I missing?
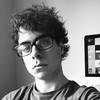
Zach Allan
19,452 PointsJust change i=1
to i=4
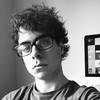
Zach Allan
19,452 PointsAnd don't forget to put your console.log(i)
inside the curly braces of the for
loop.
Answer:
for (var i=4; i<157; i+=1) {
console.log(i);
}
Jessica Stout
4,828 PointsFantastic!!!! Thank you so very much!!!!! That helps tremendously!
I appreciate your quick reply! This was driving me insane!
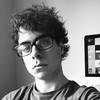
Zach Allan
19,452 PointsNo problem! Haha it's great when you finally figure something out! Glad I could help :)
Rich Donnellan
Treehouse Moderator 27,671 PointsRich Donnellan
Treehouse Moderator 27,671 PointsGood explanation – however, in this case, it should be
i = 4;
.