Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial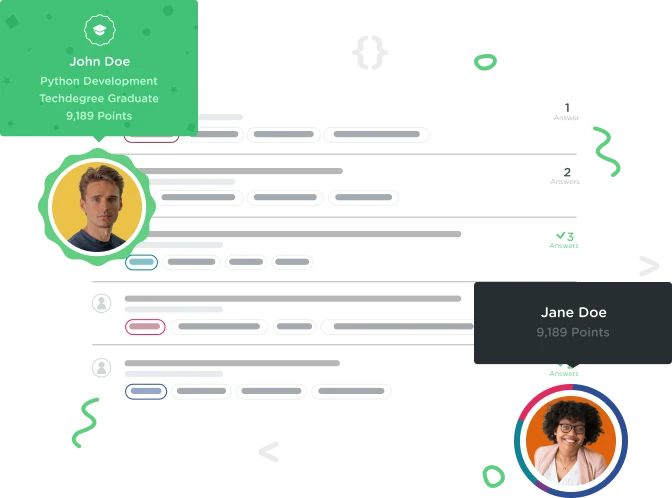

izqcabvvli
4,111 PointsHelp to solve code challenge!! Also please explain the logic and thanks in advance.
So back to that ScrabblePlayer. I found that it's not enough to know if they just have a tile of a specific character. We need to know how many they actually have. Can you please add a method called getTileCount that uses the for each loop you just learned to loop through the characters and increment a counter if it matches? Return the count, please, thanks!
public class ScrabblePlayer {
private String mHand;
public ScrabblePlayer() {
mHand = "";
}
public String getHand() {
return mHand;
}
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
}
public boolean hasTile(char tile) {
return mHand.indexOf(tile) > -1;
}
}
5 Answers
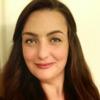
Jennifer Nordell
Treehouse TeacherGratz on getting to the trickier stuff!
Let's say we want to know how many "R"s you have in your scrabble hand.
First, we're going to initialize a counter variable of type int to 0. Because we don't know yet how many "R"s you have in your hand we're going to say 0 to start with.
Second, we have a sequence of letters in your scrabble hand. However, that sequence is represented as a string. To compare a character against it we have to convert it to an array of individual letters. Right now the program sees that entire thing as one single word and can't really look at the individual characters. Now we're going to use our for each to start at the beginning of that array and go down the entire thing. Every time we hit an R we're going to increment our counter.
Third, when we get to the end we'll know how many "R"s we have. And that's the number we'll send back... or "return". Take a look at my solution:
public int getTileCount(char tile) { //declare method and take a tile... return an int
int tileCount = 0; //initialize the counter
for(char myTile: getHand().toCharArray()) { //convert the hand to a character array and start searching for a "R"
if(myTile == tile) { //If it's an "R"
tileCount++; //increment counter
}
}
return tileCount; //return final number of "R"s
}

izqcabvvli
4,111 PointsThanks a lot Jennifer:)!
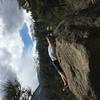
Nicholas Olsen
1,698 PointsYou are a life saver! Thank you so much for the clear explanation!! I had wondered if we were supposed to declare the method as an "int" since it would be returning one. I hadn't seen it in the videos, unless I am totally blind and missed it. Again, thanks Jennifer!
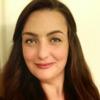
Jennifer Nordell
Treehouse TeacherNicholas Olsen Glad it was useful!
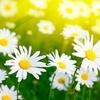
soo ao long tian
1,111 Pointsthanks! love it!

Sami Väisänen
2,398 PointsThanks a lot!
Grigorij Schleifer
10,365 PointsGrigorij Schleifer
10,365 PointsSuperb explanation Jennifer !!!!!
Michael VanKlompenberg
2,292 PointsMichael VanKlompenberg
2,292 PointsExcellent explanation much appreciated