Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial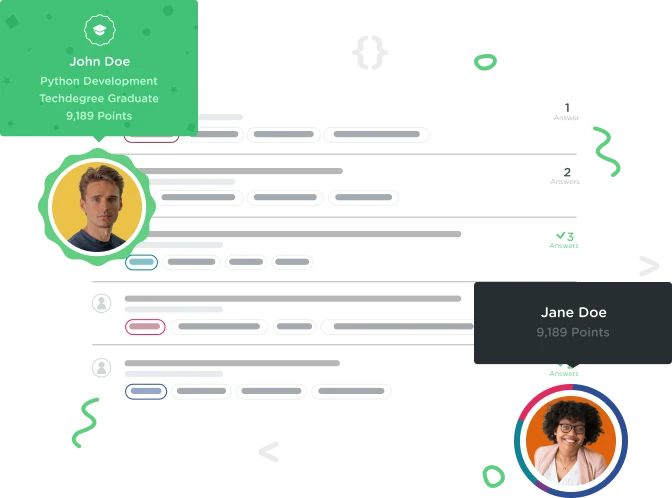

Sam Simion
847 PointsHelp to solve unit test task 1
Hello i have been looking on this task for a while now, and have no clue on how to solve it is there any solution for this task?
thanks in advance
namespace TreehouseDefense
{
public class Map
{
public readonly int Width;
public readonly int Height;
public Map(int width, int height)
{
if(width < 1 || height < 1)
{
throw new System.ArgumentOutOfRangeException(
"Map must be at least 1x1");
}
Width = width;
Height = height;
}
public bool OnMap(Point point)
{
return point.X >= 0 && point.X < Width &&
point.Y >= 0 && point.Y < Height;
}
}
}
using Xunit;
namespace TreehouseDefense.Tests
{
public class MapTests
{
[Fact]
public void OnMapTest()
{
Assert.True(false, "This test needs an implementation");
}
}
}
using System;
namespace TreehouseDefense
{
public class Point
{
public readonly int X;
public readonly int Y;
public Point(int x, int y)
{
X = x;
Y = y;
}
public double DistanceTo(Point point)
{
return Math.Sqrt(Math.Pow(X - point.X, 2.0) + Math.Pow(Y - point.Y, 2.0));
}
}
}
2 Answers

James Churchill
Treehouse TeacherSam,
Just for reference, here are the instructions for the code challenge:
"Fill out MapTests.OnMapTest to test that Map.OnMap returns true when passed a point that is on the map."
To start, think about what you need to do in order to successfully call the Map.OnMap
method. Do you need to instantiate an instance of the Map class? What do you need to pass to the OnMap
method?
Once you have the code written to call the Map.OnMap
method, then think about the property values that the Map and Point objects need to have in order for the OnMap
method to return a value of true
. Then, once you have the OnMap
method returning a value of true
, you can use the Assert.True
method to validate that the OnMap
method's return value is in fact the expected value of true
.
I hope this helps :)
Thanks ~James
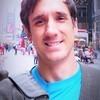
johnamann
14,064 PointsIt helped me to think about it from Assert to Arrange. Assert-
Assert.True(result);
The result parameter of the assertion (Assert.True) comes from the method tested in Act-
var result = mapInstance.OnMap(parameter);
The mapInstance is instantiated in Arrange. The parameter of the OnMap method is initialized in Arrange-
//1) use the new keyword to instantiate Map
Map yourMap = new Map(yourMapWidth, yourMapHeight);
//2) initialize x and y for yourPoint
//3) instantiate yourPoint using x and y just like you instantiated yourMap
Of course, your code must follow the order Arrange, Act, then Assert. Ask yourself what the method's signature is (it's name and what type of parameter or parameters it takes) and what it returns. That is why the Map and Point class are so helpful.
Steven Barkley
8,924 PointsSteven Barkley
8,924 PointsI have compile errors with my submission even with the downloaded solution, So I'm not even going to move forward with this track.