Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial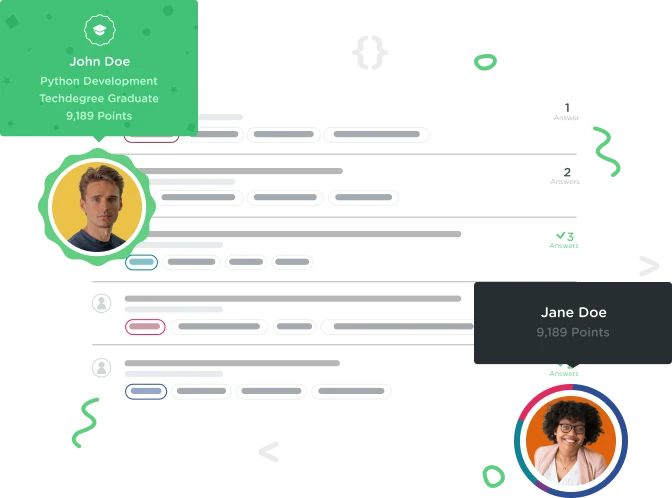
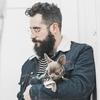
Alexander D
8,685 PointsHelp to understand Setter
CodePen: https://codepen.io/DeMichieli/pen/jgYqzN
Hello there,
I am trying to understand the Setter method. I understand they are used to change the properties of an object in a dynamic way. However, In all examples we focus on changing one property per time.
Also, I am not sure why we are not using functions to change properties? Example below:
class Math {
constructor (width, heigth) {
this.width = width
this.heigth = heigth
}
get multiply () {
return this.width * this.heigth
}
}
const result1 = new Math (2, 2)
const result2 = new Math (4, 4)
Let's say I want to change width and height to another value dynamically. For instance 9 and 7. Why can't I use the following:
newValues (width, heigth) {
this.width = width
this.heigth = heigth
}
}
when I call " result1.newValues(9, 7)" in the console this method updates dynamically width and height.
1) Is this considered a Setter method? 2) If not, since a Setter uses only one parameter, how can I update width and height at the same time?
Thanks!
1 Answer
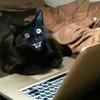
Owen Bell
8,060 Points1) A setter method uses special keyword set
, so the example you have provided is just an ordinary method. Setter methods allow you more control over how your object properties are assigned: for example, you can use conditional statements to limit the values your setter can accept, such as restricting it to certain data types or upper and lower bounds, whereas setting a property using this.prop
will just assign the newly provided value. Setters and getters also communicate to other developers and yourself which of your object's properties are designed to be stored and retrieved, and give you the option of performing actions on the data you're storing/accessing before your program exits the method.
2) The recommendation here is simply to use a simple method that calls on the setter methods for your values independently. It would look exactly like what you have already provided, but the statements assigning values to this.width
and this.height
would instead be method calls to setters with the same name, which take your method's arguments and assign them to this._width
and this._height
:
constructor (width, height) {
this._width = width;
this._height = height;
}
set width (width) {
this._width = width;
}
set height (height) {
this._height = height;
}
newValues (width, height) {
this.width = width;
this.height = height;
}
This example makes it seem like getters and setters only serve to complicate the code or oppose the DRY principle, but they help future-proof your code and make it more robust, aiding its extensibility.
Alexander D
8,685 PointsAlexander D
8,685 PointsOwen Bell I really appreciate you taking the time to respond thoroughly to my question!
Owen Bell
8,060 PointsOwen Bell
8,060 PointsAlexander D my pleasure! Glad it was useful to you :)