Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial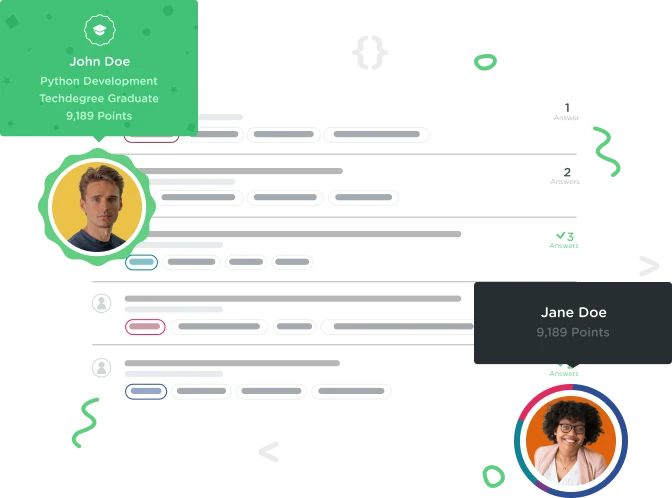

Kirome Thompson
5,350 PointsHelp to write a function covers that returns a list of courses from a dict of courses?
Let's write some functions to explore set math a bit more. We're going to be using this COURSES dict in all of the examples. Don't change it, though! So, first, write a function named covers that accepts a single parameter, a set of topics. Have the function return a list of courses from COURSES where the supplied set and the course's value (also a set) overlap. For example, covers({"Python"}) would return ["Python Basics"].
COURSES = {
"Python Basics": {"Python", "functions", "variables",
"booleans", "integers", "floats",
"arrays", "strings", "exceptions",
"conditions", "input", "loops"},
"Java Basics": {"Java", "strings", "variables",
"input", "exceptions", "integers",
"booleans", "loops"},
"PHP Basics": {"PHP", "variables", "conditions",
"integers", "floats", "strings",
"booleans", "HTML"},
"Ruby Basics": {"Ruby", "strings", "floats",
"integers", "conditions",
"functions", "input"}
}
def covers(topics):
course_list = []
for key in COURSES.keys():
if topics == COURSES.values():
course_list.extend(key)
return course_list
2 Answers
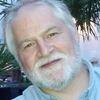
Jeff Muday
Treehouse Moderator 28,726 PointsYou seem to be on the right track. Though you cannot really use the COURSES.values() in an equivalence '==' expression. We will want to, instead, use the "in" operator. This will allow us to see if the topic from the required_topics is contained in one of the key/value pairs of courses.
Note there is no single "right way" to do this challenge, but many different right ways to pass the challenge.
I find it is difficult to do this type of challenge without breaking down the COURSES into its component key/value pairs in a loop. You should get into the habit of using the pattern below:
# this method gets all our key/value pairs into key and value variables from "my_dictionary"
for key, value in my_dictionary.items():
print(key, value)
Finally, lets incorporate this idea into our function...
def covers(required_topics):
matched_courses = set() # the set of our matched courses
# make sure we check every topic in the required_topics list
for topic in required_topics:
# here is where we break down the course/topics key/value pairs from COURSES
for course, topics in COURSES.items():
# if the topic is in the course topics, add it to the set
if topic in topics:
matched_courses.add(course)
return list(matched_courses)
Good luck with Python. It is one of the best languages to learn!
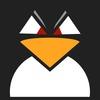
mhjp
20,372 Points2 things of note for this challenge.
-
The idea is to use Set Math to come up with the solution. i.e from the teachers notes
| or .union(*others) - all of the items from all of the sets. & or .intersection(*others) - all of the common items between all of the sets. - or .difference(*others) - all of the items in the first set that are not in the other sets. ^ or .symmetric_difference(other) - all of the items that are not shared by the two sets.
course_list.extend() will iterate over the string and add each letter to the list i.e. course_list.extend() will output something like ['P', 'y', 't', 'h', 'o', 'n', ' ', 'B', 'a', 's', 'i', 'c', 's'] instead use course_list.append().
def covers(topic):
course_list = []
for key in COURSES.keys():
if COURSES[key] & topic:
course_list.append(key)
return course_list