Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial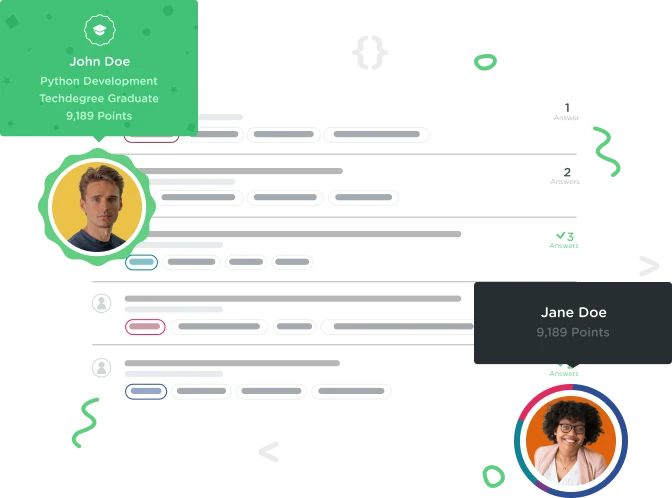

Benjamin Hedgepeth
5,672 PointsHelp understanding callback functions
Steven Parker, or anyone for that matter
I'm really struggling with callback functions in JavaScript. If you could breakdown step by step what is happening in this code it would be extremely helpful.
1) function greeting(name) {
2) alert('Hello ' + name);
3) }
4)
5) function processUserInput(callback) {
6) var name = prompt('Please enter your name.');
7) callback(name);
8) }
9)
10) processUserInput(greeting);
How is it possible for a function (Line 1), that is declared with a parameter, be treated as a callback which does not have its parameter (Line 10)? How does the omission of the parameter affect the function body of greeting
? What is being referenced if we don't include the parameter as apart of the callback?
There is no explicit definition of reference in JavaScript, so how do we know that a function without parentheses is a reference?
Finally, please simply code in JavaScript. By that please don't use Jquery because I'm just not there yet.
4 Answers

Thomas Nilsen
14,957 PointsWhat is being referenced if we don't include the parameter as apart of the callback
There is a difference between passing in a callback, and actually calling it.
instead of
processUserInput(greeting);
you could just as easily write this, if this is more understandable for you:
processUserInput(function greeting(name) {
document.write('Hello ' + name);
});
And inside processUserInput we call our callback by saying callback(name)
Meaning we're actually calling greeting(name) (the function we passed in)
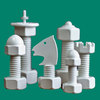
Steven Parker
229,771 Points
I got your tag notice.
You asked, "How is it possible for a function that is declared with a parameter, be treated as a callback which does not have its parameter?" As Thomas pointed out, when the function is passed as a callback, it is not being invoked ("called"). Only the name of the function is being passed.
But then on line 7 the function (which is now named "callback") does get invoked. And here the argument it needs is being passed to it.
Whenever you call a function, you must have parentheses after the name even if no argument is being passed. This is how JavaScript knows if you are calling the function or just referencing it. Here's a simple example of invoking vs. referencing:
function hey() { return "Hey there!"; }
var reference = hey; // "reference" now contains a reference to the function
var message = hey(); // "message" now contains "Hey there!"
var message2 = reference(); // "message2" now also contains "Hey there!"
I hope that clears things up. Happy coding!

Benjamin Hedgepeth
5,672 PointsSo treating greeting
as a callback it would look like this right? ...
5) function processUserInput(greeting) {
6) var name = prompt('Please enter your name.');
7) greeting(name);
}
Which would look like...
5) function processUserInput(greeting) {
6) var name = prompt('Please enter your name.');
7) alert("Hello " + name)(name);
}
This is what I don't get. I refer to the greeting
function in the processUserInput
argument. Referring to the function body of greeting
I would insert
alert("Hello " + name)
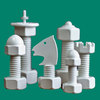
Steven Parker
229,771 PointsGiven the current code, the equivalent of: "greeting(name)
" (or the original "callback(name)
") would be: "alert('Hello ' + name)
". The latter replaces the entire call, not just the name of the function. This replacement would only be valid if processUserInput were called with function "greeting" as its argument. The whole point of a callback is that it might be called with some other function, in which case it would do something entirely different.
alert("Hello " + name)(name);
but this is not valid syntax.

Benjamin Hedgepeth
5,672 PointsWith the function greeting
being invoked on it's own. It would generate an alert dialog box with "Hello name"; name
being whatever argument that is passed.
In the context of processUserInput
, the function body of greeting
is stored for the callback. For which a response is retrieved from a user and stored in the variable name
. Then it is decided that particular variable will be used as an argument for the callback. Am I understanding this right?
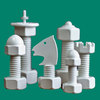
Steven Parker
229,771 PointsClose. The callback stores a reference to the function, not the actual function body. Otherwise, I think you have the basic idea.