Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial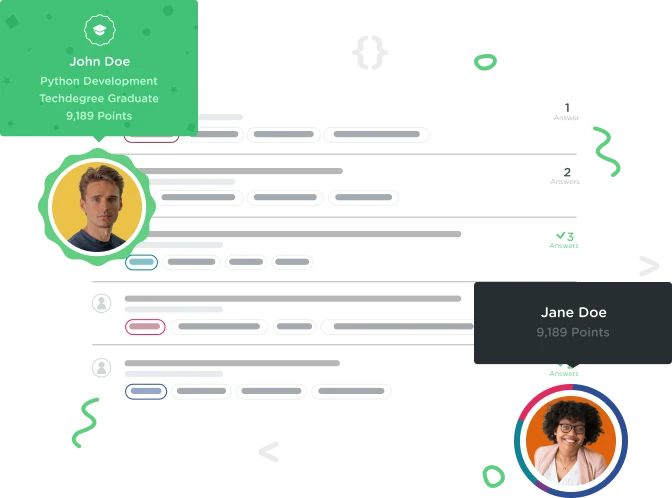

Nina Kozlova
11,602 PointsHelp understanding the code, please
var $password = $("#password"); var $confirmPassword = $("#confirm_password");
$("form span").hide();
THESE FUNCTIONS... we are telling them to return something without checking whether the condition is true. How does this work???
+++++++++++++++++++++++++++++++++++++++++++++ function isPasswordValid() { return $password.val().length > 8; }
function arePasswordsMatching() { return $password.val() === $confirmPassword.val(); }
function canSubmit() { return isPasswordValid() && arePasswordsMatching(); } +++++++++++++++++++++++++++++++++++++++++++++
Here we're declaring a function, which checks something. It appears that it checks wither the functions declared earlier return true. But how can those function return false if we told them to return the specific statement???
+++++++++++++++++++++++++++++++++++++++++++++ function passwordEvent(){ if(isPasswordValid()) { $password.next().hide(); } else { $password.next().show(); } } function confirmPasswordEvent() { if(arePasswordsMatching()) { $confirmPassword.next().hide(); } else { $confirmPassword.next().show(); } } +++++++++++++++++++++++++++++++++++++++++++++
IN SETTING THE PROPERTY OF THE ELEMENT WITH THE ID "SUBMIT" TO "DISABLED" WE ADD AN AFORE-DECLARED FUNCTION WITH AN EXCLAMATION MARK BEFORE IT. ARE WE TELLING THE FUNCTION NOT TO RUN? OR ARE WE SAYING THAT THE FUNCTION SHOULD RETURN FALSE?
++++++++++++++++++++++++++++++++++++++++++++++ function enableSubmitEvent() { $("#submit").prop("disabled", !canSubmit()); } ++++++++++++++++++++++++++++++++++++++++++++++ $password.focus(passwordEvent).keyup(passwordEvent).keyup(confirmPasswordEvent).keyup(enableSubmitEvent); $confirmPassword.focus(enableSubmitEvent).keyup(enableSubmitEvent);
enableSubmitEvent();
//Problem: Hints are shown even when form is valid
//Solution: Hide and show them at appropriate times
var $password = $("#password");
var $confirmPassword = $("#confirm_password");
//Hide hints
$("form span").hide();
function isPasswordValid() {
return $password.val().length > 8;
}
function arePasswordsMatching() {
return $password.val() === $confirmPassword.val();
}
function canSubmit() {
return isPasswordValid() && arePasswordsMatching();
}
function passwordEvent(){
//Find out if password is valid
if(isPasswordValid()) {
//Hide hint if valid
$password.next().hide();
} else {
//else show hint
$password.next().show();
}
}
function confirmPasswordEvent() {
//Find out if password and confirmation match
if(arePasswordsMatching()) {
//Hide hint if match
$confirmPassword.next().hide();
} else {
//else show hint
$confirmPassword.next().show();
}
}
function enableSubmitEvent() {
$("#submit").prop("disabled", !canSubmit());
}
//When event happens on password input
$password.focus(passwordEvent).keyup(passwordEvent).keyup(confirmPasswordEvent).keyup(enableSubmitEvent);
//When event happens on confirmation input
$confirmPassword.focus(enableSubmitEvent).keyup(enableSubmitEvent);
enableSubmitEvent();
<!DOCTYPE html>
<html>
<head>
<title>Sign Up Form</title>
<link rel="stylesheet" href="css/style.css" type="text/css" media="screen" title="no title" charset="utf-8">
</head>
<body>
<form action="#" method="post">
<p>
<label for="username">Username</label>
<input id="username" name="username" type="text">
</p>
<p>
<label for="password">Password</label>
<input id="password" name="password" type="password">
<span>Enter a password longer than 8 characters</span>
</p>
<p>
<label for="confirm_password">Confirm Password</label>
<input id="confirm_password" name="confirm_password" type="password">
<span>Please confirm your password</span>
</p>
<p>
<input type="submit" value="SUBMIT" id="submit">
</p>
</form>
<script src="//code.jquery.com/jquery-1.11.0.min.js" type="text/javascript" charset="utf-8"></script>
<script src="js/app.js" type="text/javascript" charset="utf-8"></script>
</body>
</html>
3 Answers
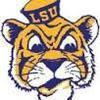
Cena Mayo
55,236 PointsHi Nina,
These functions:
function isPasswordValid() {
return $password.val().length > 8;
}
function arePasswordsMatching() {
return $password.val() === $confirmPassword.val();
}
function canSubmit() {
return isPasswordValid() && arePasswordsMatching();
}
can return true (or false) because they're using conditional operators; that is, the greater than, true equality, and AND operators ('>', '===', '&&', respectively). These always return a value of true or false. See conditional operators and boolean values for more information about this.
So, the first function, for example, will return TRUE anytime the length of the $password is greater than 8, and FALSE if less than 8. In the second function, it will return TRUE if both the $password and $confirmPassword fields are exactly the same in both value and type (meaning - they're both the same integers in the same order, or the same strings. The 3rd function returns true if the return values of the first two functions are both true, and false otherwise.
The above paragraphs should also explain your second question about how they can ever return false. If they don't meet the condition in the function, they return false. If they do, they return true.
In your 3rd question, you ask in regards to the exclamation point: "ARE WE TELLING THE FUNCTION NOT TO RUN? OR ARE WE SAYING THAT THE FUNCTION SHOULD RETURN FALSE?"
The exclamation is the NOT operator. Let's say that canSubmit() returns TRUE. Then !canSubmit() means NOT TRUE, or FALSE. If canSubmit() returns FALSE, then it means NOT FALSE, or TRUE.
Hope that helps clarify things!

Nina Kozlova
11,602 PointsSo it is not imperative to spell it out like: function isPasswordValid(){ if ($password.val().length > 8){ return true; } else { return false;}
Writing it as
function isPasswordValid() { return $password.val().length > 8;}
already implies that the function will return false if the condition (which is not written out as an if clause) is true?
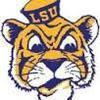
Cena Mayo
55,236 PointsThat's exactly right, Nina.
ARG! I misread your comment. It's correct to say, rather, that 'the function will return true if the condition is true'. It will return false if the condition is not true. Conditional operators always return a Boolean value (that is, true or false), so it's not necessary to include an explicit if/else loop to determine which to return.

Nina Kozlova
11,602 PointsThe thing is, Melisa, this is not my code - I copied the code in the challenge. And that is what I am trying to understand - the logic behind that code. Unfortunately, your answer didn't shed any light on my puzzle, but I appreciate it none the less.

Nina Kozlova
11,602 PointsCena, thank you ever so much!!!! Have a great day.
melisa pettaway
9,556 Pointsmelisa pettaway
9,556 Pointsyour conditional statements are in the wrong place. for example your first part try this. $(span).show(); function isPasswordValid() {
if($password.val().length > 8 && $password.val() === $confirmPassword.val()){ $(span).hide(); } }
function canSubmit() { if(isPasswordValid){
$(span).append("<p>Ok to Submit</p>");
} }
so you see you can nest control flow statements inside of a method and return what ever info you need to. just note that 1) you are returning so it wont show anything in the doc. 2) the conditionals are returning boolean values 3)your spans are the messages so you need to point to them when trying to show and hide the messages. you can give them individual ids and point to the id names. in my example i just pointed to all spans. also you can do whatever you wants with canSubmit i just put that there to show you that you can see where the conditional and block statements go.