Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial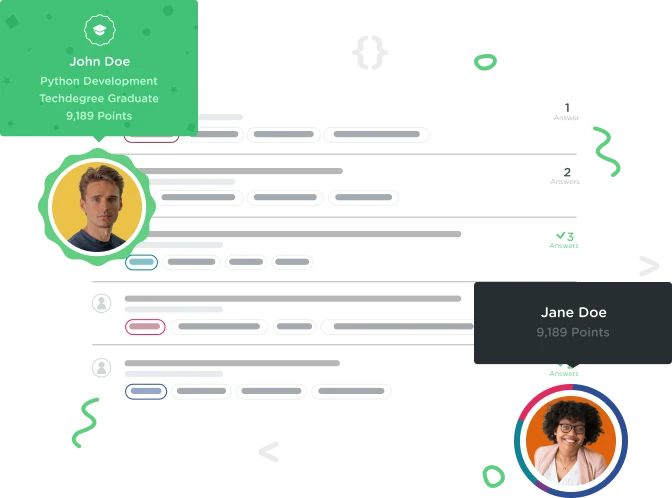

Stefan Vaziri
17,453 PointsHelp using filter() and map()
I don't think I'm using the filter() and map() right. Can someone help?
import datetime
birthdays = [
datetime.datetime(2012, 4, 29),
datetime.datetime(2006, 8, 9),
datetime.datetime(1978, 5, 16),
datetime.datetime(1981, 8, 15),
datetime.datetime(2001, 7, 4),
datetime.datetime(1999, 12, 30)
]
today = datetime.datetime.today()
def is_over_13(new_dates):
return (today - new_dates).days >= 4745
def date_string(dt_new):
fmt = '%B %d'
return dt_new.strftime(fmt)
birth_dates = filter(birthdays, map(is_over_13, date_string))
1 Answer
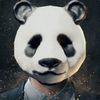
Haider Ali
Python Development Techdegree Graduate 24,728 PointsHi once again Stefan! Both map()
and filter()
are functions which take 2 arguments; a function and an iterable. When calling either of these functions, the first argument passed in should be the function you wish to call on the iterable and the second argument should be the iterable. For this challenge, we need to use filter()
to find all the birthdays which are greater than 13 years old and then we use map to apply the date_string
function to all of them. Here is how your code should look:
import datetime
birthdays = [
datetime.datetime(2012, 4, 29),
datetime.datetime(2006, 8, 9),
datetime.datetime(1978, 5, 16),
datetime.datetime(1981, 8, 15),
datetime.datetime(2001, 7, 4),
datetime.datetime(1999, 12, 30)
]
today = datetime.datetime.today()
def is_over_13(new_dates):
return (today - new_dates).days >= 4745
def date_string(dt_new):
fmt = '%B %d'
return dt_new.strftime(fmt)
birth_dates = map(date_string, filter(is_over_13, birthdays))
Notice that filter()
is nested inside map()
because we are only bothered about the birthdays which are over 13. We get all of these birthdays using filter. Once we have all of them, we create a date string by calling the date_string
function on them using map. I know this can seem kind of confusing at first, but please rewatch the video and I am sure you will understand :).
Edit:
The difference between map()
and filter()
is that map loops through the iterable and executes the specified function on each item in the iterable. However, filter()
does the same but only returns 'truthy' values. Hope this helps!
Thanks, Haider
Stefan Vaziri
17,453 PointsStefan Vaziri
17,453 PointsGot it. Thanks so much Haider!