Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial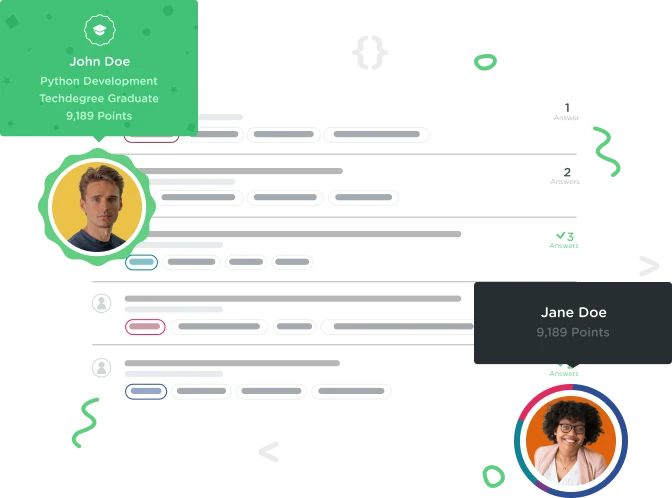
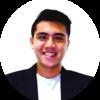
Sheng Wei
4,382 Points[Help!] What is wrong with my super.init?
Im quite sure Im on the right track, but Im not sure about the correct syntax for calling the super class and the instance. Would appreciate any help!
class Vehicle {
var numberOfDoors: Int
var numberOfWheels: Int
init(withDoors doors: Int, andWheels wheels: Int) {
self.numberOfDoors = doors
self.numberOfWheels = wheels
}
}
// Enter your code below
class car: Vehicle {
let numberOfSeats: Bool = true
override init(withDoors doors: Int, andWheels wheels: Int){
super.init(withDoors doors: doors, andWheels wheels: wheels)
self.numberOfSeats = 4
}
let someCar = someCar(doors: 2, wheels: 4)
1 Answer

Chase Marchione
155,055 PointsHi Sheng,
It's partially a syntactical issue concerning your super.init statement (in your example, you want to supply withDoors with doors, and andWheels with wheels, instead of stating doors and wheels twice on both sides of the colon.) Try replacing:
super.init(withDoors doors: doors, andWheels wheels: wheels)
with:
super.init(withDoors: doors, andWheels: wheels)
You also want to capitalize the name of your Car class (case sensitivity)...
class Car: Vehicle {
...and appropriately reference it later with your constant (while referencing withDoors and andWheels, rather than doors and wheels):
let someCar = Car(withDoors: 2, andWheels: 4)
Since you have numberOfSeats declared as a bool, it cannot be set to the value of 4. Let's try making it of type Int so that we can do that:
var numberOfSeats: Int
Your self.NumberOfSeats statement is currently after the super.init statement. Because of this, we get the error 'property 'self.numberOfSeats' not initialized at super.init call'. So, let's move it up:
self.numberOfSeats = 4
super.init(withDoors: doors, andWheels: wheels)
Finally, we're missing a second closing brace, since we need to enclose the Car class (to properly contain it.)
Your final code might look something like this:
class Vehicle {
var numberOfDoors: Int
var numberOfWheels: Int
init(withDoors doors: Int, andWheels wheels: Int) {
self.numberOfDoors = doors
self.numberOfWheels = wheels
}
}
// Enter your code below
class Car: Vehicle {
var numberOfSeats: Int
override init(withDoors doors: Int, andWheels wheels: Int){
self.numberOfSeats = 4
super.init(withDoors: doors, andWheels: wheels)
}
}
let someCar = Car(withDoors: 2, andWheels: 4)
Hope this helps!
Sheng Wei
4,382 PointsSheng Wei
4,382 PointsThanks CJ! Looks like Im still not too clear with the syntax, but this clears up a lot for me!