Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial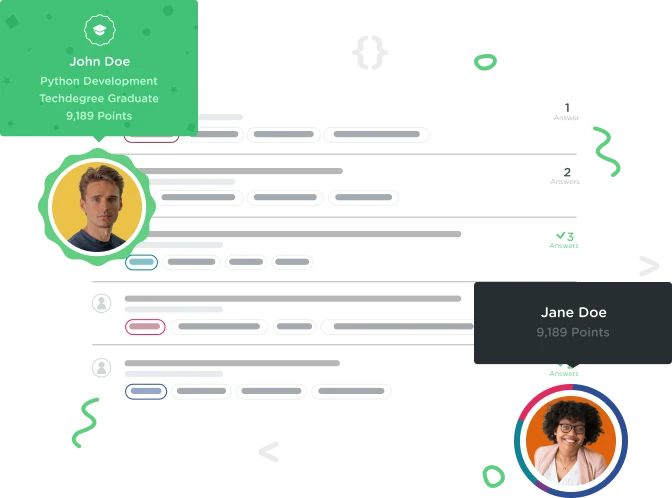
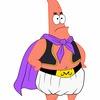
<noob />
17,062 PointsHelp with a practice project on JS DOM
Hi. Im doing a practice project which is basically a dynamic tool manager with the DOM. Im following the toturial from: https://www.youtube.com/watch?v=JaMCxVWtW58&t=416s
which teached me few things.
this is the current snapshot: https://w.trhou.se/fema7xdyoj
basically the adding a tool and remove a tool functionallity is working.
Im trying to add a Edit and Save functionallity to the app but i dont know how to do it when the table row, we didn't done it with tables in treehouse..
i tried few things already:
list.addEventListener("click", (e) => {
if(e.target.tagName === "A") {
const button = e.target;
if(button.textContent === "Edit") {
const list = document.querySelector("#tool-list");
const tr = list.firstElementChild;
const td = tr.firstElementChild;
const input = document.createElement("input");
input.type = "text";
input.value = td.innerHTML;
tr.insertBefore(input,td);
tr.removeChild(td);
button.textContent = "save";
}
}
});
this code only works on the first element in the first row because i select the "firstElementChild", i know that is the problem because i dont know how to select all the "td" tags in each row. The goal is when ill click the "Edit" button i want to have an input in all of my "td" tags inside the specified row.
another thing i'm thinking to do is to somehow loop through all the td tags but i dont have much success in it:
//Event - edit a tool
list.addEventListener("click", (e) => {
if(e.target.tagName === "A") {
const button = e.target;
if(button.textContent === "Edit") {
const input = document.createElement("input");
input.type = "text";
const list = document.querySelector("#tool-list");
const tr = list.querySelectorAll("tr");
for(let i = 0; i < tr.length; i++) {
let td = tr[i].querySelectorAll("tr")[0];
tr.insertBefore(input, td);
tr.removeChild(td);
}
I will really appreciate any help... Steven Parker
3 Answers
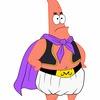
<noob />
17,062 PointsHi! first of all thank you for the reply ! I 90% understood what u did and it’s basically genious XD.
i have few questions about the code : however i don’t understand how the “save” functionality work since i don’t see any code for saving the updated input.
EDIT >> I forked the workspace and tried to use the edit button and i still dont understand how exactly when i press save the updated input get saved, the only thing that change is from this line:
<input value="tool one" disabled="">
to this line :
<input value="tool one" >
what is happeniong here is that we simply disable the option to edit which lead me to a conclusion that the updated input get saved automatically when we set deactivate the disable property?
when we press on the edit button, for example creating a td and set the textContent if the input tag to it. i’m currently on the phone so i cant fork it.
another thing i have to ask is why in order to get the parentRow of the specified input u did this :
letx=this.document.activeElement.parentElement;
var parentRow = x.parentElement
u couldn’t done this?:
parentRow = button.parentNode.parentNode;
the input is the child of the “td” tag and the “td” tag is a child of the row.
another question i have is about this line:
input.disabled = !input.disabled;
what is the purpose of this line? u set the property disabled and nigate it and in this current state u basically setting the input.disabled to “false”,
u could just do the if check like that and delete this line?
// if the current state of the input is set to disabled
if(input.disabled) {
// the button textcontent is edit
button.textContent = “Edit”
} else {
if the disable is activated then the textcontent of the button change to Save.
button.textContent = “Save”
}
i will really appreciate any insights!
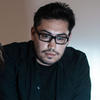
Fernando Boza
25,384 Pointshaha i forgot to post it as answer , instead of a comment, but here is the workspace
your first question is you're correct, the input value is automatically stored because again, we're just toggling the disabled attribute.
- the disabled attribute is a stand alone attribute, meaning you don't need to add = " " after it, just add disabled if you want that element to be disabled or not. What i did was toggle it on the click of the edit button.
your second question is a yes / maybe
i could and should have just done written parentElement twice like so
let parentRow = this.document.activeElement.parentElement.parentElement;
this line
input.disabled = !input.disabled;
input.disabled is a boolean value , true or false. so when i assign it to the opposite here is where i am toggling between true or false
if true turn false and vice versa, if false turn true.
then this part just checks for the value of the input.disabled ( true or false ) and sets the button text accordingly
if(input.disabled){
button.textContent = "Edit"
} else {
button.textContent = "Save"
}
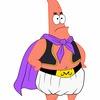
<noob />
17,062 PointsThanks for the reply! i almost understand it all but i still not quite sure about this line:
input.disabled = !input.disabled;
what do u mean by "toogling"?, I dont understand the logic behind it. why i can't just do this check it like that:
if(input.disabled){
button.textContent = "Edit"
} else {
button.textContent = "Save"
}
I will really appreciate a further explantion about this topic or a simpler way to do it.
I have come with a not very DRY solution but it make more sense:
if(button.textContent === "Edit") {
for(let input of inputsArray) {
input.disabled = false;
button.textContent = "Save";
}
} else if(button.textContent === "Save") {
for(let input of inputsArray) {
input.disabled = true;
button.textContent = "Edit";
}
}
I know it's a shitty way...