Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial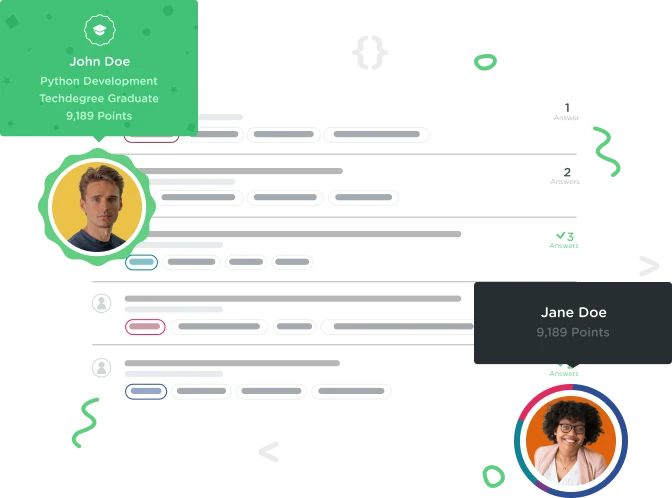
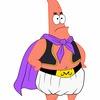
<noob />
17,062 Pointshelp with adding a local storage to my practice project
Hi!.
I'm currently in a really advaned step in the proccess of building the app.
This is the current snapshot: https://w.trhou.se/oon5ak53to
As u see, I have 2 tables. The first table is for the tools that are being in use currently. The second table is for history, when u clikc on the "X" button the current tool will be added to this table with the time u removed it.
so far i implemented local storage on the first table but i wont do be able to save the history data in the second table as well.
I'm having really hard time to figure how to do it. I can add a second key called historoyTools for instance to the localStorage and build the same addTool, removToolm fucntion to him as well? I have tried to do, ull see it on the files but i failed.
I will really appreciate ur help.
4 Answers
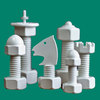
Steven Parker
240,995 PointsDid your original local storage question get resolved yet?
And here, you have created some functions but just have not used them. In particular, there is no call to "addToolToHistoryLog" anywhere, or anyplace where "getHistoryTools" is used to reload the history list.
You already have working examples using "getTools" and "addTool" for the other list, you can refer to them as you finish the implementation for the history list.
It's also a bit difficult to test code that responds in a different language!
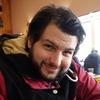
Eric M
11,546 PointsHi bot,
localStorage
can be thought of as a set of key-value pairs (in javascript this is often just called an "object") that persists through sessions.
Adding and removing from it should work the same as with any Object, but there's a catch, you can only store a single string against each key, so you need to serialise and deseralise JSON strings for nested data. Thanks to the JSON library in JavaScript this is easier than it sounds!
Here's some example code I've thrown together that adds data to localStorage, changes it, then displays it. I hope it helps you figure out your issues.
// add, remove, and read items from local storage
/*
Define some example data to work with
*/
// a string
exampleMessage = "This is some text that might appear in a message"
// a key-value object with multiple levels, you can visualise localStorage like this
exampleData = {
'firstKey' : {
'childKey' : "exampleString",
'childKeyTwo' : "another exampleString"
},
'secondKey' : "just a string"
}
// a key-value object where the values are integers
otherExampleData = {
'someData' : 3296871,
'otherData' : 6848949
}
/*
Now let's add our data to local storage. Each piece of data needs its own key
We will use these keys to retrieve or remove the data later on
*/
// add our message to local storage, we'll give it a key of "message"
localStorage.setItem('message', exampleMessage)
// add our example nested hashobject to local storage
// we can't store a object directly, but we can store a JSON string, so we need to
// convert our hashobject to a string before it can be stored in localStorage eaisly
// when we go to access it, we'll have to convert it from the JSON string
// back into a hashobject that we can access the keys of
localStorage.setItem('nested-string-object', JSON.stringify(exampleData))
// add our example integer object to local storage
// as this is another object, we have to do the same conversion to a structured string
localStorage.setItem('numbers', JSON.stringify(otherExampleData))
/*
Now let's change some of the data in local storage
*/
// overwrite our message to say something else
localStorage.setItem('message', "This is a new message")
// remove a specific key from our numbers stored in local storage
// note that we're not removing the entire dataset from numbers,
// just one value in the object stored in numbers
// first lets get the data out of local storage and into a variable we can work with
// we have to convert it back to a object remember! We do this with the parse method of JSON
rawWorkingNumbers = localStorage.getItem('numbers')
workingNumbers = JSON.parse(rawWorkingNumbers)
// now lets remove the otherData key
delete workingNumbers['otherData']
// alright, now lets overwrite the numbers item in localStorage with our updated numbers
// don't forget to translate it back to a string!
localStorage.setItem('numbers', JSON.stringify(workingNumbers))
/*
Now let's read the data we have stored
*/
// the message is a string stored directly, so we don't have to do anything special to access it
displayMessage = localStorage.getItem('message')
console.log(displayMessage)
// now let's show a specific key from our object of strings, we have to convert it from its
// representation as a JSON string back into a JavaScript object, this is easy thanks to the
// builtin JSON library
displayStrings = JSON.parse(localStorage.getItem('nested-string-object'))
displayString = displayStrings['firstKey']['childKey']
console.log(displayString)
// there should only be one int in the numbers in local storage, let's loop through this object
// and display every item, there should only be one though!
// even though there's only one object, it's still stored as a key value pair in a JSON string
// this means we have to parse it before we can iterate over it with a for loop
// we don't care about the keys, we just want to see every value, so we'll use Object.values to access these
displayNumbers = JSON.parse(localStorage.getItem('numbers'))
for (let num of Object.values(displayNumbers))
{
console.log(num)
}
The code in the snapshot you've listed is extensive and you have things like app.js
and app2.js
that make it difficult to tell exactly what code you're referencing.
When you're getting help with bigger things like this it's a good idea to either reference files and line numbers or add the relevant snippets directly into your question.
This will help other programmers quickly understand what you're trying to do and which parts of the code you need help with.
For instance, in your question above it would help if you directly referenced the code that works to add your tools to localStorage (I suspect this is the Store
class on line 142 of showtoc.js
), and a snippet of the code you've attempted that is not working as you expect.
Best of luck,
Eric
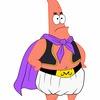
<noob />
17,062 PointsI'm referencing to maincode.js, ignore all the other js files, this is final version
this is the current snapshot translated to english: https://w.trhou.se/a5nu40es2s
I created the same functions i created for the history log table the same functions i created for the first table. I'm having troubles to add the data in the second table to the localStorage.
Ifu guys didnt understand yet, wheh i press x i delete the specifed row from the UI and from the localStorage, this row is cloned to the historylog table and contains a return time parameter in addition.
class Store {
//this fucntions for the first table
static getTools() {
let tools;
//checking if there is a tool in the localStorage
if(localStorage.getItem("tools") === null) {
tools = [];
} else {
tools = JSON.parse(localStorage.getItem("tools"));
}
return tools;
}
static addTool(tool) {
//first we get the tools from the localStorage by using the getTools function
const tools = Store.getTools();
//t add the tool to the tools array
tools.push(tool);
//setting the tool to be in the localstorage
localStorage.setItem("tools", JSON.stringify(tools));
}
static removeTool(toolNumber) {
const tools = Store.getTools();
tools.forEach((tool,index) => {
if(tool.number === toolNumber) {
tools.splice(index, 1);
}
});
//update the localStorage
localStorage.setItem("tools",JSON.stringify(tools));
}
///this fucntiopns are for the second table
static getHistoryTools() {
let historyTools;
if(localStorage.getItem("historyTools") === null) {
historyTools = [];
} else {
historyTools = JSON.parse(localStorage.getItem("historyTools"));
}
return historyTools
}
static addToolToHistoryLog(tool) {
const historyTools = Store.getHistoryTools();
historyTools.push(tool);
localStorage.setItem("historyTools",JSON.stringify(historyTools));
}
}
Now to use it like i used it the first table, i tried to call the addToolHistoryLog here:
static addToolToHistoryList(historyTool,takeTimeToolTd) {
//i get a ref to the history-list which will store the history
const historyList = document.querySelector("#history-list");
//then i create a tr(row) which will get inserted the specified cells from the "delete event" fucntion that we passing to this function
let historyRowTest = document.createElement("tr");
//getting the return date when we press on the x button
const returnDate = new Date().toLocaleString();
//then i insert to the row the values that we got from the "delete event" function
historyRowTest.innerHTML = `
<td>${historyTool.name}</td>
<td>${historyTool.toolName}</td>
<td>${historyTool.number}</td>
<td>${takeTimeToolTd}</td>
<td>${returnDate}</td>
`;
//and finally i append the row to the history table
historyList.appendChild(historyRowTest);
//saving the row in the log table
Store.addToolToHistoryLog(historyRowTest);
}
Im referencing line 77 in maincode.js
I really appreciate if u can check the current snap shot now
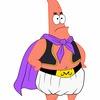
<noob />
17,062 Points****UPDATE****
this the current snapshot: https://w.trhou.se/c6qcduckes
The historyTool key get added to the localStorage after i delete it but it still dont save it on the page How is it possible that i see it got saved in the localstorage and i dont see it on the app?
picture: https://gyazo.com/6ae59adaf7d9b959e5e665b24be184c0
i tried to place the addToolHistoryLog fucntion here as well
static addToolToHistoryList(historyTool,takeTimeToolTd) {
//i get a ref to the history-list which will store the history
const historyList = document.querySelector("#history-list");
//then i create a tr(row) which will get inserted the specified cells from the "delete event" fucntion that we passing to this function
let historyRowTest = document.createElement("tr");
//getting the return date when we press on the x button
const returnDate = new Date().toLocaleString();
//then i insert to the row the values that we got from the "delete event" function
historyRowTest.innerHTML = `
<td>${historyTool.name}</td>
<td>${historyTool.toolName}</td>
<td>${historyTool.number}</td>
<td>${takeTimeToolTd}</td>
<td>${returnDate}</td>
`;
//and finally i append the row to the history table
historyList.appendChild(historyRowTest);
Store.addToolToHistoryLog(historyTool);
}
i saw the row from the "tools" key now has an empty array([]) and the "historyTools" has the row now but it still dont work
<noob />
17,062 Points<noob />
17,062 PointsI will upload a test code in english sry for that.