Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial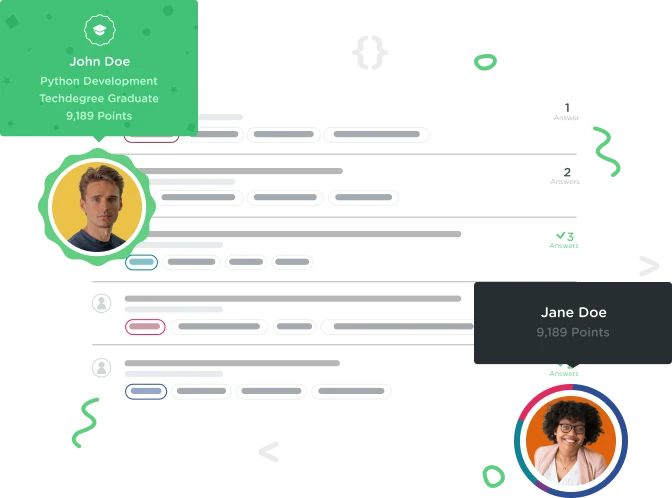
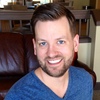
Kris Byrum
32,636 PointsHelp with Array in an Array please
I'm attempting to put my resume together for employers and I'm having a little trouble with the array in an array.
I started with a foreach loop then changed it because it didn't work. I tried a few other things, but I eventually settled on this current version.
I want to make a list in HTML for each of my duties for each of my employers, but I can't get the list for the duties to work.
Could you help please?
Here is my mark up (I shortened it to keep it clean here)
<?php
function employer_list_view($job) {
$output = "";
$output = $output . "<h3>" . $job["employer"] . "</h3>";
$output = $output . "<h3>" . $job["title"] . "</h3>";
$output = $output . "<h3>" . $job["city"] . "</h3>";
$output = $output . "<ul>";
$output = $output . "<li>" . $job["first"] . "</li>";
$output = $output . "<li>" . $job["second"] . "</li>";
$output = $output . "<li>" . $job["third"] . "</li>";
$output = $output . "</ul>";
};
$jobs = array();
$jobs[102] = array(
"employer" => "Better Business Bureau",
"title" => "Investigator",
"city" => "Seattle, WA",
"first" => "Regularly conducted analysis...",
"second" => "Drafted public alerts...",
"third" => "Handled incoming...."
);
?>
2 Answers
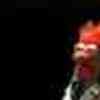
James Andrews
7,245 PointsThe foreach loop is the correct way to handle a multidimensional array. You should however not make a habit of concatinating strings of HTML in your php code, it makes trouble shooting much more difficult. Build your data, and then build your HTML then where you need to loop through data in the HTML or do conditionals in the HTML break in and out of the php code like this.
<?php
$jobs = array();
$jobs[102] = array(
"employer" => "Better Business Bureau",
"title" => "Investigator",
"city" => "Seattle, WA",
"first" => "Regularly conducted analysis...",
"second" => "Drafted public alerts...",
"third" => "Handled incoming...."
);
?>
<html>
<body>
<?php foreach($jobs as $job): ?>
<h3><?php echo $job["employer"]; ?></h3>
<h3><?php echo $job["title"]; ?></h3>
<h3><?php echo $job["city"]; ?></h3>
<ul>
<li><?php echo $job["first"]; ?></li>
<li><?php echo $job["second"]; ?></li>
<li><?php echo $job["third"]; ?></li>
</ul>
<?php endforeach; ?>
</body>
</html>
This is much easier to read debug and update as necessary.

Jason Cullins
Courses Plus Student 4,893 PointsAlso, just FYI, you can take:
$output = $output . "<h3>" . $job["employer"] . "</h3>";
and use it cleaner like this:
$output .= "<h3>" . $job['employer'] . "</h3>";
just use a dot equal, because
$output = 'Hello '; $output .= 'World';
echo($output);
Echo Reads:
Hello World
Hope this helps too! :)