Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial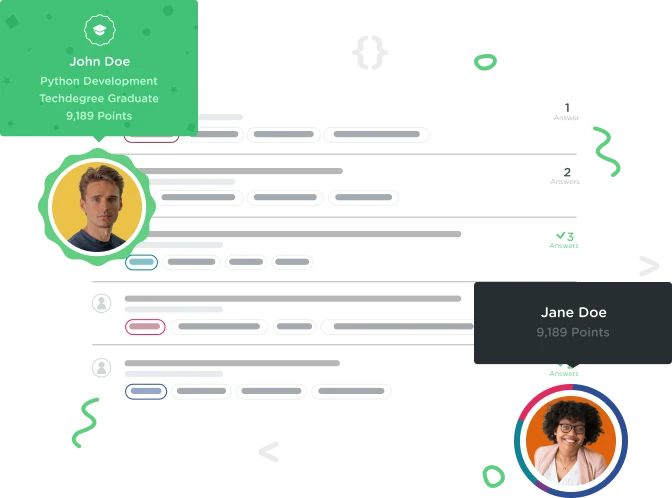

Colby Wise
3,165 PointsHelp with Challenge error - "for-each not applicable to expression type | require Array or List"
I think i'm close to the solution but I'm having trouble with my nested for loop. In the second for loop im trying to iterate from category to getCategory but i'm not passing an array or list
if I'm thinking about the solution incorrectly please also let me know!
package com.example;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.TreeSet;
public class Blog {
List<BlogPost> mPosts;
public Blog(List<BlogPost> posts) {
mPosts = posts;
}
public List<BlogPost> getPosts() {
return mPosts;
}
public Set<String> getAllAuthors() {
Set<String> authors = new TreeSet<>();
for (BlogPost post: mPosts) {
authors.add(post.getAuthor());
}
return authors;
}
public Map<String,Integer> getCategoryCounts() {
Map<String,Integer> categoryCounts = new HashMap<String,Integer>();
//categoriedPost = new ArrayList<BlogPost>();
for (BlogPost post : mPosts) {
for (String category : post.getCategory()) { //How do I iterate over categories?
Integer count = categoryCounts.get(category);
if (count == null) {
count = 0;
}
count++;
categoryCounts.put(category,count);
}
}
}
1 Answer

Simon Coates
28,694 PointsEach post has a single category if you look at the BlogPost source code. So you've trying to loop on something that can't be looped on.
public Map<String,Integer> getCategoryCounts() {
Map<String,Integer> categoryCounts = new HashMap<String,Integer>();
//categoriedPost = new ArrayList<BlogPost>();
for (BlogPost post : mPosts) {
String category = post.getCategory();
Integer count = categoryCounts.get(category);
if (count == null) {
count = 0;
}
count++;
categoryCounts.put(category,count);
}
return categoryCounts ;
}
this is a very common mistake that people seem to make. not sure if it's because people assume there should be multiple categories or if its a function of confusion over the for loop. If you read the colon in the for loop as 'in', then it should be clear that the syntax is only for collections. Apart from this, your code was missing a return statement.