Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial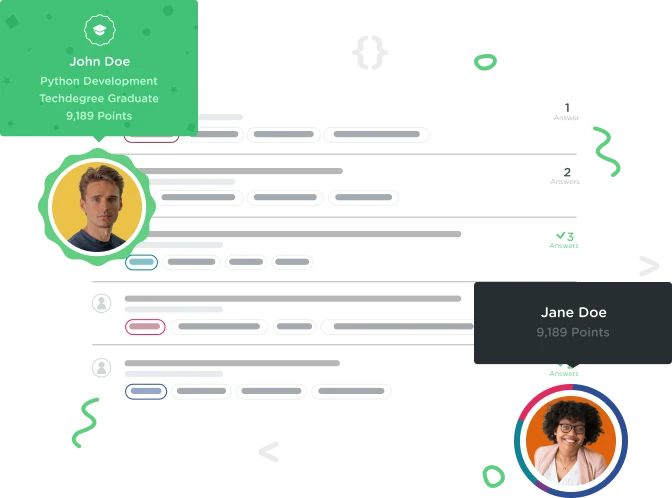
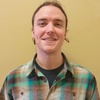
Nathan Pickard
10,950 PointsHelp with code
Help with code
I am working on the "Building a self-destructing message android app" project with Android Studio and I'm stuck trying to figure out how to translate the code from Eclipse (which Ben is using in the video).
After doing some searching on these forums, this is what I have come up with. However I am getting an error in my mRecipientsListView.setOnClickListener(new AdapterView.OnItemClickListener() method below. Anyone know of a solution to get through this project using Android Studio?
Here is my code for RecipientsActivity.java
package apps.nathanpickard.ribbit;
import android.app.AlertDialog;
import android.os.Build;
import android.support.v4.app.NavUtils;
import android.support.v7.app.ActionBarActivity;
import android.os.Bundle;
import android.util.Log;
import android.util.SparseBooleanArray;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.widget.AdapterView;
import android.widget.ArrayAdapter;
import android.widget.ListView;
import android.widget.TextView;
import com.parse.FindCallback;
import com.parse.ParseException;
import com.parse.ParseQuery;
import com.parse.ParseRelation;
import com.parse.ParseUser;
import org.w3c.dom.Text;
import java.util.List;
import butterknife.ButterKnife;
import butterknife.InjectView;
public class RecipientsActivity extends ActionBarActivity {
public static final String TAG = RecipientsActivity.class.getSimpleName();
protected List<ParseUser> mFriends;
protected ParseRelation<ParseUser> mFriendsRelation;
protected ParseUser mCurrentUser;
protected MenuItem mSendMenuItem;
@InjectView(R.id.RecipientsList) ListView mRecipientListView;
@InjectView(R.id.empty) TextView mEmptyFriendList;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_recipients);
ButterKnife.inject(this);
mRecipientListView.setOnClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> adapterView, View view, int i, long l) {
if (getCheckedItemCount() > 0) {
mSendMenuItem.setVisible(true);
} else {
mSendMenuItem.setVisible(false);
}
}
});
mRecipientListView.setChoiceMode(ListView.CHOICE_MODE_MULTIPLE);
}
@Override
public void onResume() {
super.onResume();
mCurrentUser = ParseUser.getCurrentUser();
mFriendsRelation = mCurrentUser.getRelation(ParseConstants.KEY_FRIENDS_RELATION);
ParseQuery<ParseUser> query = mFriendsRelation.getQuery();
query.addAscendingOrder(ParseConstants.KEY_USERNAME);
query.findInBackground(new FindCallback<ParseUser>() {
@Override
public void done(List<ParseUser> friends, ParseException e) {
if (e == null) {
mFriends = friends;
String[] usernames = new String[mFriends.size()];
int i = 0;
for (ParseUser user : mFriends) {
usernames[i] = user.getUsername();
i++;
}
ArrayAdapter<String> adapter = new ArrayAdapter<String>(
mRecipientListView.getContext(),
android.R.layout.simple_list_item_checked,
usernames);
mRecipientListView.setAdapter(adapter);
mRecipientListView.setEmptyView(mEmptyFriendList);
} else {
Log.e(TAG, e.getMessage());
AlertDialog.Builder builder = new AlertDialog.Builder(RecipientsActivity.this);
builder.setMessage(e.getMessage())
.setTitle(R.string.error_title)
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
}
});
}
public int getCheckedItemCount() {
ListView listView = mRecipientListView;
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.HONEYCOMB) {
return listView.getCheckedItemCount();
}
SparseBooleanArray checkedItems = listView.getCheckedItemPositions();
int count = 0;
for (int i = 0, size = checkedItems.size(); i < size; ++i) {
if (checkedItems.valueAt(i)) {
count++;
}
}
return count;
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.menu_recipients, menu);
mSendMenuItem = menu.getItem(0);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
switch(item.getItemId()) {
case android.R.id.home:
NavUtils.navigateUpFromSameTask(this);
return true;
case R.id.action_send:
return true;
}
return super.onOptionsItemSelected(item);
}
}
activity_recipients.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".RecipientsActivity">
<ListView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:id="@+id/RecipientsList"
android:layout_alignParentLeft="true"
android:layout_alignParentTop="true"
android:layout_alignParentStart="true"/>
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/empty"
android:text="@string/empty_recipients_list_message" />
</RelativeLayout>
5 Answers

miguelcastro2
Courses Plus Student 6,573 PointsNathan,
This issue has nothing to do with Android Studio - it is just basically a text editor. The error in your code shows the problem. The method: mRecipientListView.setOnClickListener() expects a View object, whereas your code passed an AdapterView. I believe the method you are looking for is not for when the ListView is clicked, but rather when a ListView item is clicked. Use the method setOnItemClickListener() to set the listener for when a ListView's item is clicked. Moreover, this method receives an AdapterView object as your code is currently passing:
mRecipientListView.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> adapterView, View view, int i, long l) {
if (getCheckedItemCount() > 0) {
mSendMenuItem.setVisible(true);
} else {
mSendMenuItem.setVisible(false);
}
}
});
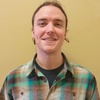
Nathan Pickard
10,950 PointsI am getting and error in this block of code in RecipientsActivity.java:
mRecipientListView.setOnClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> adapterView, View view, int i, long l) {
if (getCheckedItemCount() > 0) {
mSendMenuItem.setVisible(true);
} else {
mSendMenuItem.setVisible(false);
}
}
});
It is all underlined in read and it's saying:
"setOnClickListener (android.view.View.OnClickListener) in AdapterView cannot be applied to (anonymous android.widget.AdapterView.OnItemClickListener)
What exactly should I correct here? I'm sure other Android Studio users are getting stuck like me.
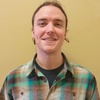
Nathan Pickard
10,950 PointsThanks miguelcastro2, simply changing "setOnClickListener" to "setOnItemClickListener" did the job. It appears I need to pay more attention to the methods I'm using and what's being pasted through. Cheers!

abdul rehman
Python Web Development Techdegree Student 1,025 PointsHy athan Pickard! i'm also using Android Studio and follow you suggestion to remove the error, but when i run my app and try to create a new message click on choose Photo and select a photo from gallery then my app has crashed it' says Ribbit has stopped , help me plz!
RecipientsActivity.java
package browsetolearn.com.ribbit;
import android.app.AlertDialog;
import android.os.Build;
import android.support.v4.app.NavUtils;
import android.support.v7.app.ActionBarActivity;
import android.os.Bundle;
import android.util.Log;
import android.util.SparseBooleanArray;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.widget.AdapterView;
import android.widget.ArrayAdapter;
import android.widget.ListView;
import android.widget.TextView;
import com.parse.FindCallback;
import com.parse.ParseException;
import com.parse.ParseQuery;
import com.parse.ParseRelation;
import com.parse.ParseUser;
import org.w3c.dom.Text;
import java.util.List;
public class RecipientsActivity extends ActionBarActivity {
public static final String TAG = RecipientsActivity.class.getSimpleName();
protected List<ParseUser> mFriends;
protected ParseRelation<ParseUser> mFriendsRelation;
protected ParseUser mCurrentUser;
protected MenuItem mSendMenuItem;
ListView mRecipientListView = (ListView) findViewById(android.R.id.list);
TextView mEmptyFriendList = (TextView) findViewById(android.R.id.empty);
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_recipients);
mRecipientListView.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> adapterView, View view, int i, long l) {
if (getCheckedItemCount() > 0) {
mSendMenuItem.setVisible(true);
} else {
mSendMenuItem.setVisible(false);
}
}
});
mRecipientListView.setChoiceMode(ListView.CHOICE_MODE_MULTIPLE);
}
@Override
public void onResume() {
super.onResume();
mCurrentUser = ParseUser.getCurrentUser();
mFriendsRelation = mCurrentUser.getRelation(ParseConstants.KEY_FRIENDS_RELATION);
ParseQuery<ParseUser> query = mFriendsRelation.getQuery();
query.addAscendingOrder(ParseConstants.KEY_USERNAME);
query.findInBackground(new FindCallback<ParseUser>() {
@Override
public void done(List<ParseUser> friends, ParseException e) {
if (e == null) {
mFriends = friends;
String[] usernames = new String[mFriends.size()];
int i = 0;
for (ParseUser user : mFriends) {
usernames[i] = user.getUsername();
i++;
}
ArrayAdapter<String> adapter = new ArrayAdapter<String>(
mRecipientListView.getContext(),
android.R.layout.simple_list_item_checked,
usernames);
mRecipientListView.setAdapter(adapter);
mRecipientListView.setEmptyView(mEmptyFriendList);
} else {
Log.e(TAG, e.getMessage());
AlertDialog.Builder builder = new AlertDialog.Builder(RecipientsActivity.this);
builder.setMessage(e.getMessage())
.setTitle(R.string.error_title)
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
}
});
}
public int getCheckedItemCount() {
ListView listView = mRecipientListView;
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.HONEYCOMB) {
return listView.getCheckedItemCount();
}
SparseBooleanArray checkedItems = listView.getCheckedItemPositions();
int count = 0;
for (int i = 0, size = checkedItems.size(); i < size; ++i) {
if (checkedItems.valueAt(i)) {
count++;
}
}
return count;
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.menu_recipients, menu);
mSendMenuItem = menu.getItem(0);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
switch (item.getItemId()) {
case android.R.id.home:
NavUtils.navigateUpFromSameTask(this);
return true;
case R.id.action_send:
return true;
}
return super.onOptionsItemSelected(item);
}
}
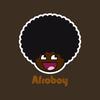
adsdev
15,583 Pointsfor the newer people that encounter problems with deprecated ActionBarActivity, replace it with AppCompatActivity
public class RecipientsActivity extends AppCompatActivity{
/**
*Rest of the code from Nathan's code works. Its working for me right now :)
* Do carefully observe his code, there may be some parts you didn't correctly implement
*
*/
Nathan Pickard If it is not too much to ask and if you're ok with it, can you add some comments on the parts you had to " repair" to make it work ? I dont get the getCheckItemCount() Method for a 100%.
miguelcastro2
Courses Plus Student 6,573 Pointsmiguelcastro2
Courses Plus Student 6,573 PointsWhat is the error you are getting?