Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial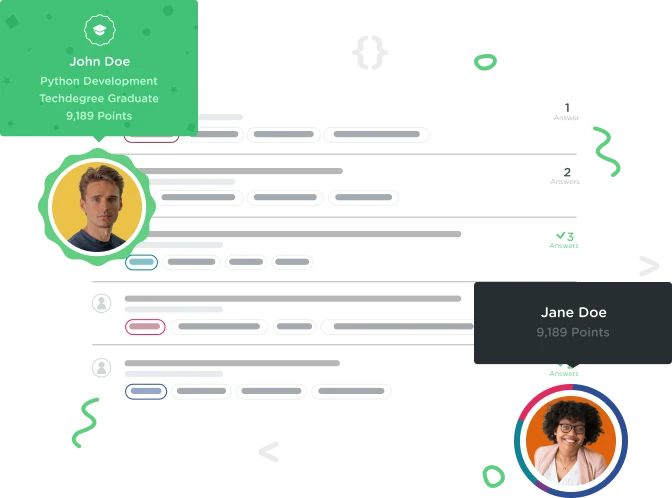

Tony Brackins
28,766 PointsHelp with code
Anyone feel like breaking down what this code means exactly?
!function(root){
"use strict";
function Dice(sides) {
this.sides = sides;
}
Dice.prototype.roll = function () {
var randomNumber = Math.floor(Math.random() * this.sides) + 1;
return randomNumber;
}
if (typeof module !== 'undefined' && module.exports) {
module.exports = Dice;
} else {
root.Dice = Dice;
}
}(this);
Particularly !function(root){}(this)
and the if statement with module and module.exports etc.
Thanks in advance.
1 Answer
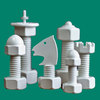
Steven Parker
229,744 PointsHere's a breakdown of the lines in question:
!function(root){...}(this)
This is an immediately invoked function expression (IIFE, pronounced "iffy"). The exclamation point (!) is a way to insure that the JavaScript engine recognizes it as a complete expression and not just a a function definition (another way would be to enclose the whole thing in parentheses). The function is invoked passing the current context ("this") as the argument, which is then assigned to the parameter variable "root".
if (typeof module !== 'undefined' && module.exports)
The first part of this test determines that "module" exists (is not undefined), and the second part determines that it has a property named "exports". Based on that test, the following lines either assign the Dice function to that property, or to a "Dice" property which is added to the current context ("root").
Tony Brackins
28,766 PointsTony Brackins
28,766 Pointsgotcha. Thx Steven. The checking for module piece is still a little cloudy for me though I read that it's checking for NodeJS but I need to do some further learning perhaps more object oriented programming.