Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial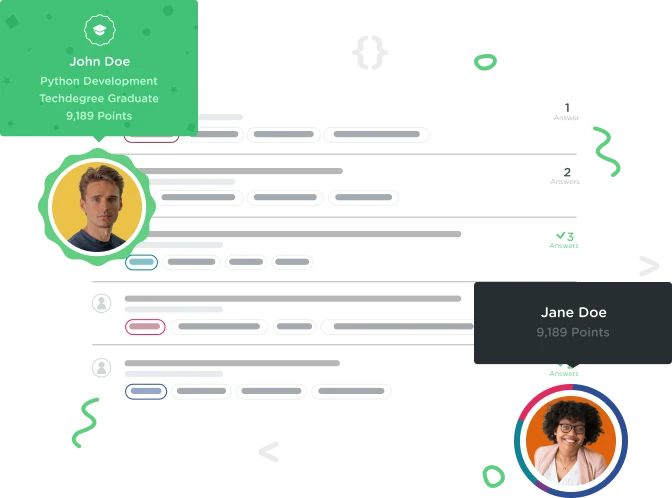

Alex Cawley
9,291 PointsHelp with condensing Javascript/jQuery
Basically i wanted to test my knowledge of the javascript and jQuery that i have been learning here and reading from books elsewhere so I decided to make a very simple "web app", if you can even call it that, that generates a media query to your specifications. When you click on the fields to give it certain breakpoints and types i wanted a box to appear to the side to show a few common examples and the default value for the text area to disappear so the user doesn't have to back space a bunch of time before typing. Then all of this to undo when clicked away. I was able to accomplish all of this, but in a very very cluttered way. Specifically the examples and the disappearing text. I repeated my code very badly and was wondering if someone could help me with suggestions on how to condense it. Here is my code, Thanks!
The html
<input id="type" type="username" value="Type"></input>
<div class="examples example1">
<p>A few common types:</p>
<ul>
<li>Screen</li>
<li>Print</li>
<li>All</li>
<li>Aural</li>
<li>Braille</li>
<li>Embossed</li>
<li>Handheld</li>
<li>Projection</li>
<li>Screen</li>
<li>tty</li>
<li>tv</li>
</ul>
</div>
<input id="parenth" type="username" value="Style Breakpoint"></input>
<div class="examples example2">
<p>A few common breakpoints:</p>
<ul>
<li>Mobile Portrait: 320px</li>
<li>Mobile Landscape: 480px</li>
<li>Small Tablet Portrait: 600px</li>
<li>Small Tablet Landscape: 800px</li>
<li>Tablet Portrait: 768px</li>
<li>Tablet Landscape: 1024px</li>
</ul>
</div>
The javascript
var $input1 = $('#type');
var $input2 = $('#parenth');
var default_value1 = $input1.val();
var default_value2 = $input2.val();
$('.examples').hide();
$input1.focus(function() {
$('.example1').fadeToggle('fast');
if($input1.val() == default_value1) {$input1.val("")};
}).blur(function(){
$('.example1').fadeToggle('fast');
if($input1.val().length == 0) {$input1.val(default_value1)};
});
$input2.focus(function() {
$('.example2').fadeToggle('fast');
if($input2.val() == default_value2) {$input2.val("")};
}).blur(function(){
$('.example2').fadeToggle('fast');
if($input2.val().length == 0) {$input2.val(default_value2)};
});
If you need the rest of the code just ask
1 Answer

Dino Paškvan
Courses Plus Student 44,108 PointsThere are many ways to reduce the amount of code here. You could write a function and just call the function for each input... but in this case, you can reduce the code even further by doing something like this:
- First of all, instead of the
value
attribute, use theplaceholder
attribute. It already acts the way you want it to, so there's no need to do the hiding of the text programatically. - Second, hide the elements with the
.examples
class in CSS, not JavaScript. - And finally, use the jQuery
.next()
method to get the next sibling of an element. Your example divs are siblings to the input fields. So, you can easily get to the next sibling of the focused input field and show/hide it.
The end result is visible in this CodePen.
Alex Cawley
9,291 PointsAlex Cawley
9,291 PointsThanks that's exactly what im looking for!