Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial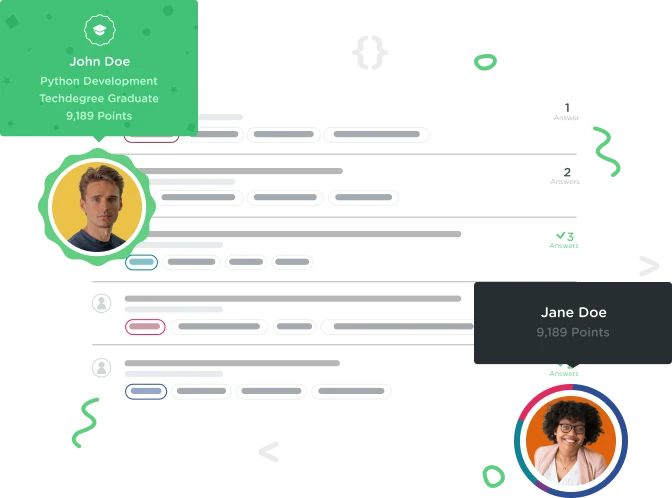
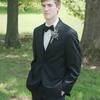
Jamison Imhoff
12,460 PointsHelp with Dict Code Challenge Python
Need to return number of teachers in the dict, keep getting error expected 5 got 16. "Create a function named num_teachers that takes a dictionary of teachers and returns the number of teachers."
# The dictionary will be something like:
# {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Often, it's a good idea to hold onto a max_count variable.
# Update it when you find a teacher with more classes than
# the current count. Better hold onto the teacher name somewhere
# too!
#
# Your code goes below here.
# Your code goes below here.
teacher_dict = {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
'Kenneth Love': ['Python Basics', 'Python Collections']}
def most_classes(test):
max_count = 0
busy_teacher = ''
for teacher1,teacher2 in test.items():
if len(teacher2) > max_count:
max_count = len(teacher2)
busy_teacher = teacher1
return busy_teacher
def num_teachers(test2):
teach_count = 0
for teacher1,teacher2 in test2.items():
if len(teacher1) > teach_count:
teach_count = len(teacher1)
return teach_count
4 Answers
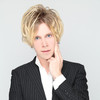
Mikael Enarsson
7,056 PointsThis is a semantic error (the worst kind of error, since you can run your code without error, but you don't get the answers you expect!). I noticed that you already managed to get past this problem, but if you are interested, I wanted to go through what your code actually did in a bit of detail. It may be a bit long, but I think it would be worth reading, just so you get an idea of how to analyze the code and discover these kind of errors. If you are just interested in why len(test2) worked, I'll explain it briefly at the bottom.
def num_teachers(test2): #Define the function "num_teachers",
#taking a dictionary of teachers and classes
#(really, it would be good practice to name
#your argument "teachers_dict" or "some_teachers" so that
#it's easy to understand what to input, but that's fine
teach_count = 0 #create a counter for teachers, initialize to 0
#here it can get a bit snary, but hang with me!
for teacher1,teacher2 in test2.items(): #.items() returns a list of tuple pairs. If you don't know what
#a tuple is, don't worry, it's in the course, but for now,
#just consider them some, in this case a pair, of values
#allowing you to make multiple assignments simultaneously.
#In this case, it assigns the name of the teacher to teacher1 and
#the list of that teachers classes to teacher two.
#It does this for EVERY teacher in the dict
if len(teacher1) > teach_count: #here, you check if the length (i.e, how many items are in) teacher1.
#teacher1 is the name of the teacher in the current iteration of the
#for loop. Note that this will be true for the first pass through the loop
#(since teach_count is initialized to 0), and after that ONLY if the
#current teachers name string is longer than the teacher currently in
#teacher_count
teach_count = len(teacher1) #if the new teachers name string is longer then the currently held one, the
#lenght of the new teachers name replaces the old one, and so on throughout
#the list
return teach_count #returns the value of teacher count, which is now the lenght of the longest
#teacher name string
Now, in regards as to why len(test2) worked, len() returns the length of, or the number of items in, certain kinds of objects (or maybe all kinds, reading the documentation made me a bit unsure), among others, lists and dicts. That's pretty much all there is to it.
I hope that this is of some help/interest, and that others who are better informed help to correct me if I made any mistakes!
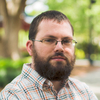
Kenneth Love
Treehouse Guest Teacherlen()
works on any type that has .__len__()
defined...which is way farther into the internals of Python than we need to go just yet.
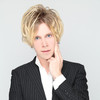
Mikael Enarsson
7,056 PointsOk, fair enough. Sounds very interesting though~~
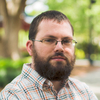
Kenneth Love
Treehouse Guest TeacherWhen you loop through a dictionary, you only loop through the keys. Since len()
effectively loops through and counts all of the members of something, it only counts the keys.
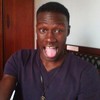
Paul Vitalis
2,858 PointsKenneth, I got the correct answer by doing this but I think there is a more logical way. Please help me out
def num_teachers(dct): for name in dct: teacher= dct[name] return len(teacher) + 1
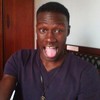
Paul Vitalis
2,858 Pointsdef num_teachers(dct): for name in dct: teacher= dct[name] return len(teacher) + 1
Why do I get the correct anser when I do this ? and would someone please help me with their code so that i may compare
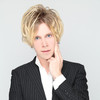
Mikael Enarsson
7,056 PointsQuite frankly, it's probably just chance, in this case. It's difficult to know in this case, but I'm going to assume that your code looks something like this:
def num_teachers(dct):
for name in dct:
teacher= dct[name]
return len(teacher) + 1
This code takes a dictinory (dct
), takes the name (name
) of every teacher in the dictionary in turn , and assigns the list of that name
to teacher
. Then, it returns the lenght of teacher
+ 1. It's simply chance that the last list is one less than the expected answer.
Now, what you want to do is check the number of items in dct
, or simply:
def num_teachers(dct):
return len(dct)

Eldor Ibragimov
1,733 Pointsdef num_teachers(dictionary): count = 0 for key in dictionary.keys(): count += 1 return count
Jamison Imhoff
12,460 PointsJamison Imhoff
12,460 PointsUPDATE
I just ventured out with a simple solution and it worked:
Now can someone please explain why this works. I understand that it is simply returning the length of 'test2', but how does the code know that test2 is referencing the x in the dict??? (ie dict = {x: y}) ??
I am brand new to coding so little things like this I need to understand.