Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial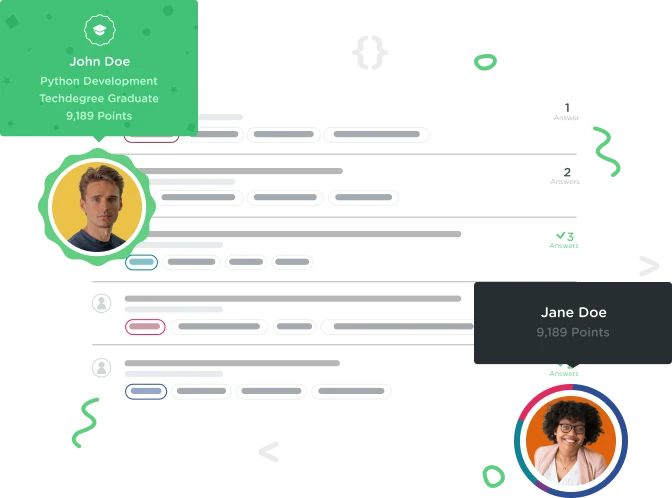

Timothy Van Cauwenberge
8,958 PointsHelp with discount program
I'm writing a program that takes an input of what the user spent and gives back the amount they saved. Every time I put in an input of 10 or greater I get back "java.util.IllegalFormatConversionException: null (in java.util.Formatter$FormatSpecifier) and it highlights line 28 (System.out.printf("You win a discount coupon of $%d. (8% of your purchase)", discount);) I copied and pasted the code in here so it might come out very disorganized. Please help if you can.
import java.util.*;
public class Coupon { public static void main(String [] args) { Scanner input = new Scanner(System.in);
double purchase = 0;
double discount = 0;
System.out.println("Please enter the cost of your groceries: ");
purchase = input.nextDouble();
if (purchase < 10) {
System.out.println("You did not spend enough to get a discount coupon.");
} else if (purchase >= 10 || purchase <= 60) {
discount = 8 / purchase;
System.out.printf("You win a discount coupon of $%d. (8% of your purchase)", discount);
} else if (purchase > 60 || purchase <= 150) {
discount = 10 / purchase;
System.out.printf("You win a discount coupon of $%d. (10% of your purchase)", discount);
} else if (purchase >= 150 || purchase <= 210) {
discount = 12 / purchase;
System.out.printf("You win a discount coupon of $%d. (12% of your purchase)", discount);
} else if (purchase > 210) {
discount = 14 / purchase;
System.out.printf("You win a discount coupon of $%d. (14% of your purchase)", discount);
}
else {
}
}
}
4 Answers

Irving Amador
7,488 PointsI found the problem:
First of all you do have to use %f instead of %d for printing double numbers. Second, you have to escape the % character, you do that by putting %%
Here is an example of one line printing the message:
java> System.out.printf("You win a discount coupon of $%f. (8%% of your purchase)", discount);
You win a discount coupon of $78.054600. (8% of your purchase)
Hope it helps.

Timothy Van Cauwenberge
8,958 PointsThanks so much.

Timothy Van Cauwenberge
8,958 PointsAlso, for some reason any input no matter what the value of the number is the program only finds 8 percent of the number instead. For example if I input 70 I should get $7 back but I get back 8 percent of 70 instead of 10.

Irving Amador
7,488 PointsMmmmmm maybe you should use && instead of ||

Irving Amador
7,488 PointsMmmmmm maybe you should use && instead of ||

Irving Amador
7,488 Points%c char Character
%d int Signed decimal integer.
%e, %E float Real number, scientific notation (lowercase or uppercase exponent marker)
%f float Real number, standard notation.
Try printing the number with %f instead of %d

Timothy Van Cauwenberge
8,958 PointsI changed it to %f and is giving me the same error message.

Irving Amador
7,488 PointsThe error is at the same line?

Timothy Van Cauwenberge
8,958 PointsYes and it also doesn't matter what value I put in, even if the value is not included in the else if statement.
Irving Amador
7,488 PointsIrving Amador
7,488 PointsIt seems its a problem when you are trying to print the number in that line. Check this post http://stackoverflow.com/questions/4455951/java-printf-stack-error