Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial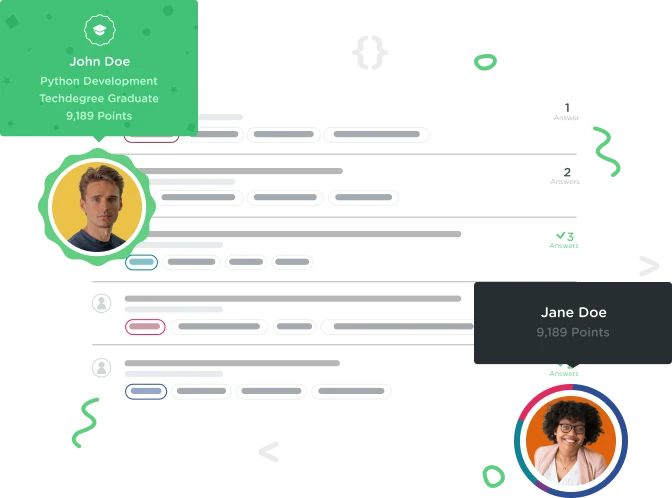
Peter Fuhrey
2,146 PointsHelp with enum methods in Swift
Hello, so I've been playing around with code a little bit today and I wanted to write out a program in my playground that whenever you passed it one of the four main compass directions it would tell you which state you were heading towards so for example: North - New York, East - Carolina, South - Florida, West - California (I know theres two Carolinas lol) I believe I'm on the right track however whenever I pass a direction different than the one I have set in my initializer I still get the value from the initializer. Here's my code:
enum Direction {
case North
case East
case South
case West
init() {
self = .North
}
func directionBound(directionHeading: Direction) -> String {
switch self {
case .North:
return "You're heading towards New York"
case .East:
return "You're heading towards the Carolinas"
case .South:
return "You're heading towards Florida"
case .West:
return "You're heading towards California"
}
}
}
var roadTrip = Direction()
roadTrip.directionBound(.East)
I understand theres probably aspects to this code which are unorthodox or just simply wrong and I would appreciate any type of constructive criticism you have for me! Thank you!
2 Answers

Chris Shaw
26,676 PointsHi Peter,
The reason you're getting the same value over and over is because your switch
statement is referring to self
which is the current enum
instance value instead of the directionHeading
parameter which you've defined within your directionBound
method.
Also it's bad practise to not include a default
case when using switches as if nothing matches your code may fail silently and can cause you hours of pain looking for a reason why, the other change is I've made your return type an optional that way we can ensure that a true direction is been returned otherwise we assume an unknown direction.
See the below.
enum Direction {
case North, East, South, West
init() {
self = .North
}
func directionBound(directionHeading: Direction) -> String? {
switch directionHeading {
case .North:
return "You're heading towards New York"
case .East:
return "You're heading towards the Carolinas"
case .South:
return "You're heading towards Florida"
case .West:
return "You're heading towards California"
default:
return nil
}
}
}
var roadTrip = Direction()
if let direction = roadTrip.directionBound(.East) {
println(direction)
} else {
println("Unknown direction")
}
Hope that helps.

Ian Bowers
3,665 PointsNeed to assign a default, not use the Swift default in the enum. Hope this works!
enum Compass: Int {
case North = 1
case South = 2
case East = 3
case West = 4
}
let direction = Compass(rawValue: 2)
Peter Fuhrey
2,146 PointsPeter Fuhrey
2,146 PointsSorry for the code being weirdly placed. I don't know how to fit it all into that gray box, or even that one existed haha.