Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial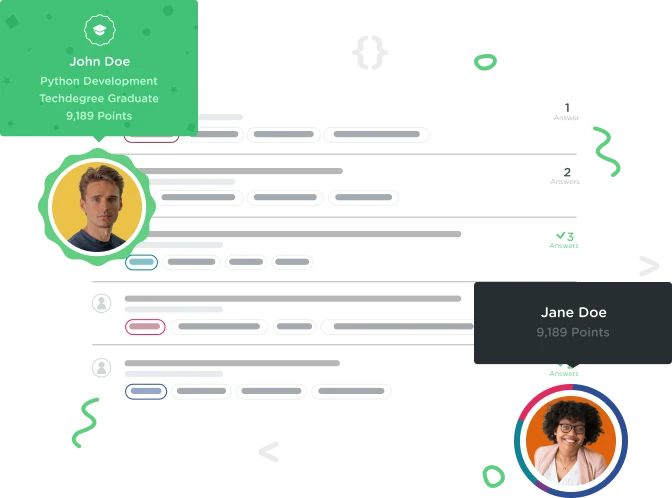

Martins Rokis
6,995 Pointshelp with exercise needed
hi, my solution is not working,as i understood i need to convert string to char array, loop through and count how many char are there and return int value what solution looks like? thanks
public class ScrabblePlayer {
private String mHand;
public ScrabblePlayer() {
mHand = "";
}
public String getHand() {
return mHand;
}
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
}
public boolean hasTile(char tile) {
return mHand.indexOf(tile) > -1;
}
public int getTileCount(){
int count=0;
for(char i:mHand.toCharArray()){
count+=1;
}
return count;
}
}
1 Answer

andren
28,558 PointsYou have misunderstood the task slightly, if the task was what you thought then your code would actually be correct. Though it would also be quite overkill, as you can just use the length method found on strings to figure out how many chars are in them.
Anyway what the task actually wants you to do is create a method that receives a char as a parameter, and then compares that char to the chars in the mHand string. It should then return a count of how many of the chars in mHand matches the char that was passed in to the method.
Doing so only requires you to slightly modify your code like this:
public class ScrabblePlayer {
private String mHand;
public ScrabblePlayer() {
mHand = "";
}
public String getHand() {
return mHand;
}
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
}
public boolean hasTile(char tile) {
return mHand.indexOf(tile) > -1;
}
public int getTileCount(char passedInChar){ // get a char as a parameter
int count=0;
for(char i:mHand.toCharArray()){
if (passedInChar == i) { // Compare the passed in char to the chars in mHand
count++;
}
}
return count;
}
}
I added comments to the code I added, with those additions you will be able to pass the challenge.
Martins Rokis
6,995 PointsMartins Rokis
6,995 Pointsthank you very much, it worked