Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial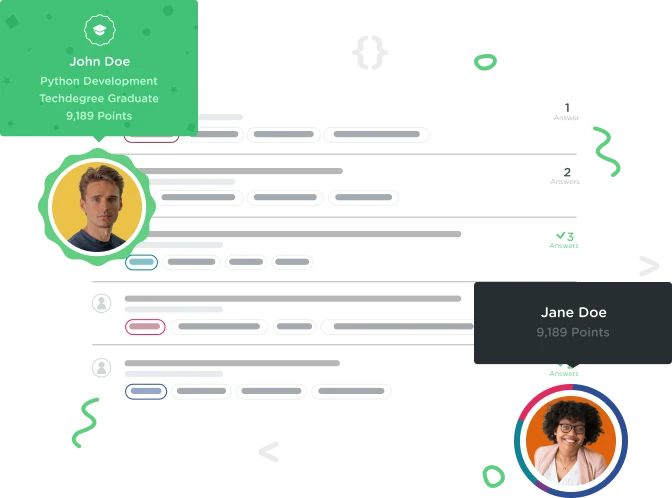

Michael Doyle
2,418 PointsHelp With Extra Credit Project Passing Dictionary to Function
I have successfully completed all the extra credit assignments, so far in the tutorials, but this one has me confused.
Here is my code:
// Playground - ExtraCredit4
import UIKit
var Song1 = ["Title" : "Freebird", "Artist" : "Lynyrd Skynyrd", "Album" : "Thyrty"]
var Song2 = ["Title" : "Money", "Artist" : "Pink Floye", "Album" : "Dark Side Of The Moon"]
var Song3 = ["Title" : "Another Brick In The Wall", "Artist" : "Pink Floyd", "Album" : "Another Brick In The Wall"]
func myMusic([title: String, artist: String, album: String]) -> (title: String, artist: String, album: String) {
return ("Title: \(title)\n","Artist: \(artist)\n", "Album: \(album)\n")
}
myMusic(Song1)
4 Answers

Stone Preston
42,016 Pointscan you post what the extra credit assignment is? does your code work? is there an error?

Michael Doyle
2,418 PointsThe assignment is: Write a function that accepts a Dictionary as parameter and returns a named tuple. The dictionary should contain the following keys: title, artist and album.
I have tried this too:
// Playground - ExtraCredit4
import UIKit
var Song1 = ["Title" : "Freebird", "Artist" : "Lynyrd Skynyrd", "Album" : "Thyrty"] var Song2 = ["Title" : "Money", "Artist" : "Pink Floye", "Album" : "Dark Side Of The Moon"] var Song3 = ["Title" : "Another Brick In The Wall", "Artist" : "Pink Floyd", "Album" : "Another Brick In The Wall"]
func myMusic(song: Dictionary) -> (title: String, artist: String, album: String) { return ("Title: (title)\n","Artist: (artist)\n", "Album: (album)\n")
} println(myMusic(Song1))
I get an error that reference to generic type dictionary requires an argument

Stone Preston
42,016 Pointsalright the problem is in your dictionary type for your parameter. the swift eBook states:
You can also write the type of a dictionary in shorthand form as [Key: Value]
so knowing that functions have parameters in the form of
func someFunc(parameterName: Type) {}
you can create your function that takes a dictionary as a parameter and returns a named tuple like so
func myMusic(myDict: [String: String]) -> (title: String, artist: String, album: String) {
}
then using the myDict parameter we can access the values for the keys of the dict and return them in a tuple. the eBook states:
You can also use subscript syntax to retrieve a value from the dictionary for a particular key. Because it is possible to request a key for which no value exists, a dictionary’s subscript returns an optional value of the dictionary’s value type. If the dictionary contains a value for the requested key, the subscript returns an optional value containing the existing value for that key. Otherwise, the subscript returns nil:
so you can force unwrap the values for the keys or use optional binding which would be safer. I used forced unwrapping below for simplicity
func myMusic(myDict: [String: String]) -> (title: String, artist: String, album: String) {
return (myDict["title"]!, myDict["artist"]!, myDict["album"]!)
}

Michael Doyle
2,418 PointsWe're getting closer:
// Playground - ExtraCredit4
import UIKit
var Song1 = ["Title" : "Freebird", "Artist" : "Lynyrd Skynyrd", "Album" : "Thyrty"] var Song2 = ["Title" : "Money", "Artist" : "Pink Floye", "Album" : "Dark Side Of The Moon"] var Song3 = ["Title" : "Another Brick In The Wall", "Artist" : "Pink Floyd", "Album" : "Another Brick In The Wall"]
func myMusic(songs: [String: String]) -> (title: String, artist: String, album: String) { return (songs["title"]!,songs["artist"]!,songs["album"]!)
} println(myMusic(Song1))
error on return line:
Execution was interrupted, reason: EXC_BAD_INSTRUCTION (code=EXC_1386_INVOP,subcode=0x0).

Donnie Wang
1,842 PointsYour error looks like it's coming from your capitalization of "Title" vs. "title", "Artist" vs. "artist" etc.
let song1 = ["title": "My Way", "artist": "Usher", "album": "17"]
let song2 = ["title": "Give it Away", "artist": "Red Hot Chili Peppers", "album": "Blood Sugar Sex Magic"]
func playlist (songs: [String: String]) -> (title: String, artist: String, album: String) {
return (songs["title"]!, songs["artist"]!, songs["album"]!)
}
println(playlist(song1))