Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial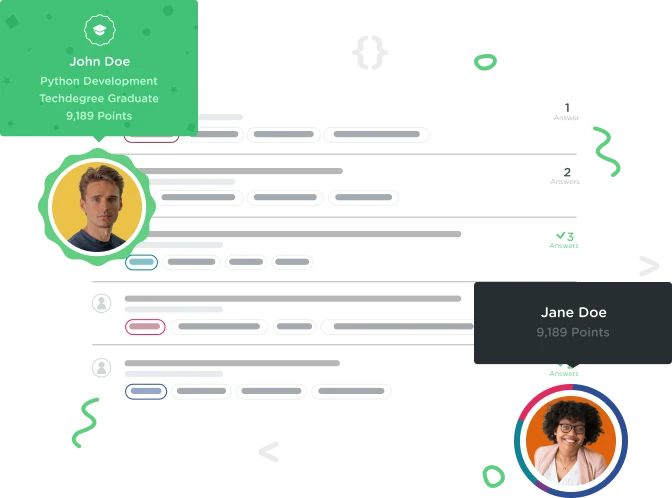
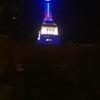
ei2
2,618 PointsHelp with "foreach" loops
In this question (from the quiz), we are given the following:
// $numbers = array(1,2,3,4); $total = count($numbers); $sum = 0; $loop = 0;
foreach($numbers as $number) { $loop = $loop + 1; if ($loop < $total) { $sum = $sum + $number; } }
echo $sum; //
According to the answers, $sum is supposed to be displayed as 6.
How? Why?
What is $loop equal to?
I thought if $loop is assigned as 0, it would be always be equal to 1 in the foreach loop ($loop + 1) and thus less than $total.
What is $total equal to?
I thought if $total is always 4 (the number of items in the $numbers array), it would always be 4 and greater than $loop.
So how do we get $sum to display as 6 from all this? Anyone?
2 Answers
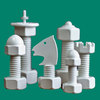
Steven Parker
231,275 PointsYou're right that $total is 4, but on the last iteration, $loop becomes 4 also and so the "if" test is no longer true.
That means the "sum" is only of the numbers less than 4, which gives you the value of 6.
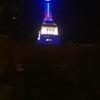
ei2
2,618 PointsAh, I see. The "foreach" loop is just like the "for" loop in that the other variables (in this case $loop and $sum) are always acted upon (in an accumulated fashion) over and over.
The operations stay the same, but the values change as an accumulation in each iteration, and depending on the conditions, the operations either stop or keep going.
I finally get it. Thanks for your help!
ei2
2,618 Pointsei2
2,618 PointsWell, ok. I guess I could see the condition changing that last time, if the variable $loop was the variable we were altering...but it isn't...(is it?).
The heart of the confusion here (at least to me) is // $loop = $loop + 1; //.
I thought $loop was always equal to 0 (and then to 1), because it was declared as 0 in the first place.
Because to me, adding 1 to $loop (within the foreach statement) will always make $loop == 1. And 1 is always less than 4, no matter how many times the code is executed.
So what is it about the foreach loop that messes with the variable $loop? I thought $loop remained unaffected because it was just another variable, unaffected by the foreach statement. It would always be == 1, because you are executing the command separately each time (foreach).
So how and when does $loop change too? Are we just supposed to substitute the array($numbers) values within $loop? If so, why?
And if we are, since when are
$sum == 1 and $sum == 2 and $sum == 3
all added together? Doesn't this take another statement?
I'm sorry if this is getting repetitive (the irony), but it really is something that I just don't understand, at least in the general idea of what a foreach loop is supposed to do. But thanks for your help, even if just reading this post and seeing where I'm coming from.
Steven Parker
231,275 PointsSteven Parker
231,275 PointsYes, $loop was initially assigned with 0 before the loop starts. But this line inside the loop changes it:
$loop = $loop + 1;
This happens during every iteration, so the value continues to increase each time to show which iteration is being performed. So on the 4th iteration, it's value is 4, and no longer smaller than $total.
Maybe it would help to look at all the variables for each iteration: