Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial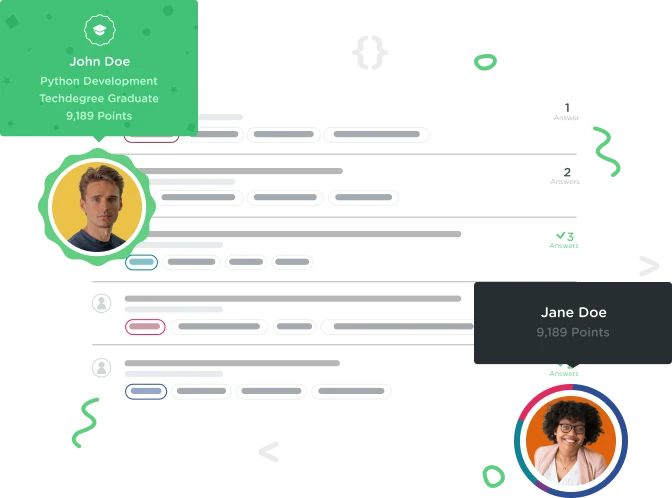

Antonis Tsagaris
17,511 PointsHelp with getter and setter challenge
Hi,
What is wrong with the code attached?
Any help will be appreciated! :)
class Temperature {
var celsius: Float
var fahrenheit: Float {
get {
return self.celsius * 1.8 + 32
}
set {
self.celsius = (newValue-32)/1.8
}
}
init(celsius: Float) {
self.celsius = celsius
}
}
5 Answers
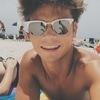
garyguermantokman
21,097 PointsYou had the answer, just omit the init() you add at the end of the class.
class Temperature {
var celsius: Float = 0.0
var fahrenheit: Float {
get {
return (celsius * 1.8) + 32.0
}
set {
celsius = (newValue - 32) / 1.8
}
}
}
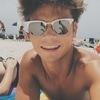
garyguermantokman
21,097 PointsYou are setting the Fahrenheit property (calls the setter) and pass the new value you are setting. You may be just over thinking it. Paste this into a playground and try it out your self.
class Temperature {
var celsius: Float = 0.0
var fahrenheit: Float {
get {
return (celsius * 1.8) + 32.0
}
set {
print(newValue) // ------> Prints 50.0
celsius = (newValue - 32) / 1.8
}
}
}
let temp = Temperature()
temp.fahrenheit = 50 // ---------> You are setting the value off Fahrenheit

Antonis Tsagaris
17,511 PointsIndeed! That was the problem!
Thanks Gary!
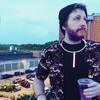
Robert Kaltenbach
10,285 PointsHi there!
I've completed this challenge as above listed, but I was having trouble as I was trying to do the following:
class Temperature {
var celsius: Float = 0.0
var fahrenheit: Float {
set {
return (celsius * 1.8) + 32.0
}
get {
celsius = (fahrenheit - 32) / 1.8
}
}
}
I'm having a hard time understanding why I cannot just directly assign celsius using the fahrenheit variable. Can someone kindly explain why I must use newValue and how the code knows the newValue I refer to means the setter above?
Thanks
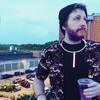
Robert Kaltenbach
10,285 PointsAh! Your print lines helped immensely! Thanks!