Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial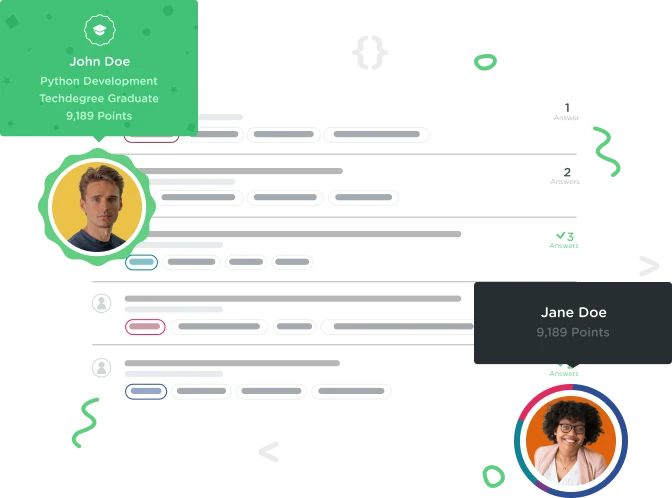
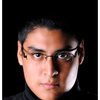
Alejandro Villalobos
4,276 PointsHelp with java please, what a I doing wrong?
Hi, I am taking a test on Build an android app and can't figure out the correct way to write this java...
String[] sports = { "Basketball", "Baseball", "Tennis" };
String bestSport = "";
int numberOfSports = sports.length;
bestSport = sports[numberOfSports];
4 Answers

Stone Preston
42,016 Pointsyour code for task 1 is incorrect, task 1 states: Declare a String variable named bestSport and initialize it to the first element of the sports array.
you just need to index the sports array. you can index arrays like so:
arrayName[index]
where arrayName is an array and index is an integer between 0 and the length of the array - 1. array indexes start at 0, so that means the first index is 0, the second is 1, etc etc.
so if I had an array named animals and wanted to assign a variable to the 3rd element of the array I would use
String[] animals = { "Cat, "Dog", "Pig", "Cow" };
String thirdAnimal = animals[2]; // 2nd index is the 3rd element since indexes start at 0
to assign a string named best sport to the first element in the sports array you would use:
String[] sports = { "Basketball", "Baseball", "Tennis" };
String bestSport = sports[0];
for the other tasks you are almost right. you neeed to name the variable lastSport, not best sport and you need to subtract 1 from the length since indexes start at 0. if you dont subtract 1, you try and access the element at index 3 which does not exist since the only indexes are 0, 1, and 2. thats what known as going out of bounds of the array
String[] sports = { "Basketball", "Baseball", "Tennis" };
String bestSport = sports[0];
int numberOfSports = sports.length
String lastSport = sports[numberOfSports - 1]; //subtract 1 from the number of sports to avoid going out of bounds. the last element is index 2, your numberOfSports is 3 so subtract 1.

Nicholas Vandenbroeck
4,599 PointsArrays in Java are zero-based. Which means that if you count the items in your array is 3. While the index of the last item in the array is 2. This gives you an exception: java.lang.ArrayIndexOutOfBoundsException.
You should do this: int numberOfSports = sports.length - 1;
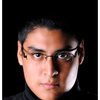
Alejandro Villalobos
4,276 PointsI am actually stuck on the 2nd question.... :o But thanks a lot :D

Stone Preston
42,016 Pointsah ok. edited answer then. your code for task 1 is incorrect so you probably need to fix that since it might prevent you from moving forward. see my updated answer for more information
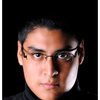
Alejandro Villalobos
4,276 PointsThanks a lot, I got it now :D
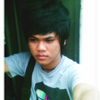
Topan Lutfi
539 PointsWhere is the answer?? Challenge task 2 of 3 Declare an int variable named numberOfSports and set it to the number of elements in the array using the array's length property.
zlatko divjakovski
874 Pointszlatko divjakovski
874 PointsThank you for your question and answers ^_^ I was stuck on the second one too, I putted int number second instead of third, and it didn't came out. Thanks to you, I've resolved it. Thanks!