Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial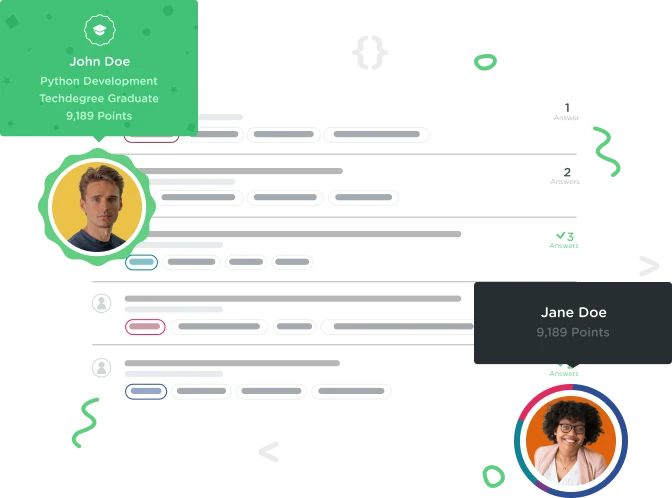

Theodor Fahami
1,034 PointsHelp with java treehouse challenge
I am being tasked this:
Okay, so let's throw an IllegalArgumentException from the drive method if the requested number of laps cannot be completed on the current battery level. Make the exception message "Not enough battery remains".
So i added this part to my code:
public class GoKart { public static final int MAX_BARS = 8; private String mColor; private int mBarsCount;
public GoKart(String color) { mColor = color; mBarsCount = 0; }
public String getColor() { return mColor; }
public void drive() { drive(1); }
public void drive(int laps) { // Other driving code omitted for clarity purposes mBarsCount -= laps;
if (laps > mBarsCount) {
throw new IllegalArgumentException("Not enough battery remains");
}
}
public void charge() { while (!isFullyCharged()) { mBarsCount++; } }
public boolean isBatteryEmpty() { return mBarsCount == 0; }
public boolean isFullyCharged() { return mBarsCount == MAX_BARS; }
}
it does not give me a error it just says: Bummer! Make sure you throw the exception before change the mBarsCount field.
please help my understand why this does not work
1 Answer

Benjamin Barslev Nielsen
18,958 PointsThe statement mBarsCount -= laps changes the mBarsCount field and the message you get: "Bummer! Make sure you throw the exception before change the mBarsCount field." means that you should not execute this statement before throwing the exception. This means the drive method should be:
public void drive(int laps) {
if (laps > mBarsCount) {
throw new IllegalArgumentException("Not enough battery remains");
}
// Other driving code omitted for clarity purposes
mBarsCount -= laps;
}