Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial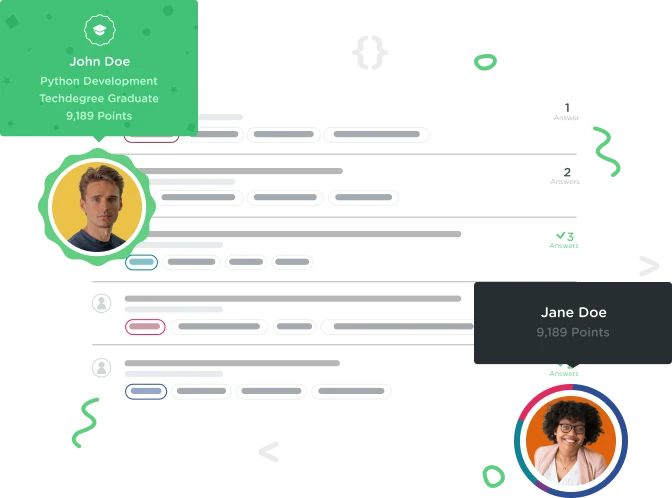
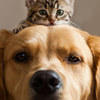
Devin Scheu
66,191 PointsHelp With JavaScript
Here's The Question: Set the 'greeting' variable, on line 29, by using the 'genericGreet' function in the context of 'andrew' with the array of arguments, 'args1'.
Here's my code:
var genericGreet = function(name, mood) {
name = name || "you";
mood = mood || "good";
return "Hello " + name + ", my name is " + this.name +
" and I am in a " + mood + " mood!";
}
var andrew = {
name: "Andrew"
}
var args1 = ["Michael", "awesome", ":)"];
var greeting = genericGreet(jim, awesome);
4 Answers

Danie Clawson
4,216 PointsOkay, I think you're confusing two different ways of doing this. You can write
function assembleGreeting(NAME, MOOD) {
return "Hello my name is " + (NAME || "you") + " and I am in a " + (MOOD || "good") + " mood!";
}
var greeting = assembleGreeting("jim","awesome"); //Don't forget quotes!
That will get you the output you expected.
However, you can also do it this way, which it looks like you were getting mixed up at:
var GenericGreet = function(name, mood) {
this.name = name || "you";
this.mood = mood || "good";
this.speak = function() {
return "Hello my name is " + this.name + " and I am in a " + this.mood + " mood!";
};
};
var greeter = new GenericGreet("jim", "awesome");
Let's break down what's going on here. This is called a Constructor function. Instead of using this function, our greeter variable could have been constructed like this:
var literalConstructed = {
name: "jim",
mood: "awesome",
speak: function() {
return "Hello my name is " + this.name + " and I am in a " + this.mood + " mood!";
};
};
With a constructor though, you can do way more to your input data, reuse the structure, have local variables, etc. Anyways now you can say alert(greeter.speak()) or you can say alert(greeter.mood), and you can edit them directly like greeter.mood = "happy";
Anyways, that's what it seems like you were being mixed up with, and why people were telling you to use apply. You can use .apply, with this method too. For example, assuming the Constructor is defined as above
var changeMe = {
existingProperty: "Golden!"
};
GenericGreet.apply(changeMe, ["jim","awesome"]);
//or you can use
GenericGreet.call(changeMe, "jim", "awesome");
What's going on here, is that you are basically running the Constructor over an existing object, and the first argument you give to .apply or .call is the variable to be used as "this" in that process.

Shawn Anderton
24,124 Pointsneed to use the apply method here is some info https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Function/apply
genericGreet.apply(andrew,args1);

Duane Moody
18,163 PointsAs Shawn stated, you need to use the apply() method when setting the var greeting.
The apply() method calls a function with a given this value and arguments provided as an array.
So your line 29 should look like:
var greeting = genericGreet.apply(andrew,args1);
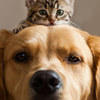
Devin Scheu
66,191 PointsI don't understand