Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial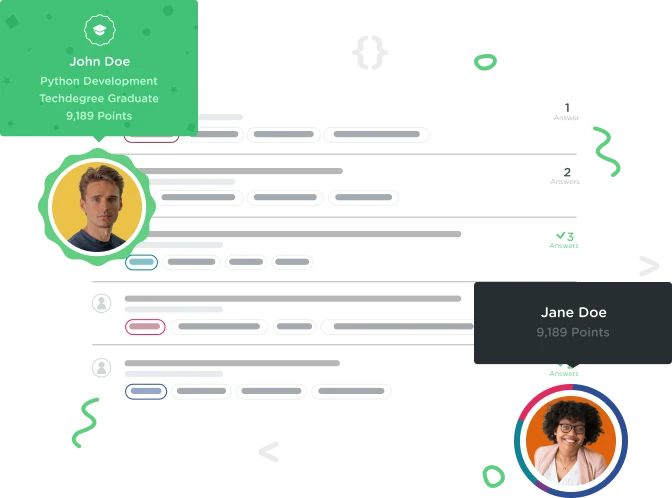

Eric C
7,998 PointsHelp with JavaScript challenge
I can't seem to get this to pass, what am I missing? Thanks
var isAdmin = false;
var isStudent = true;
if ( isAdmin = true) {
alert('Welcome administrator')
} if else ( isStudent = true ) {
alert('Welcome Student')
}
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JavaScript Basics</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
2 Answers

Douglas Counts
10,126 PointsHey Eric,
You have a couple of problems here. Firstly you are doing assignments to the variables instead of comparing their value to true
. Use three equal signs isStudent === true
to do a comparison. like so:
} else if ( isStudent === true ) {
Your else-if statement was out of order too. Notice about that the comparison comes after the if keyword. Think of it as an else-if statement, that way you don't get the order of those two keywords wrong.
Secondly, instead of comparing your values to true, you can simply check the boolean value directly like so:
if ( isAdmin ) {
which is easier to read and involves one less logic step than
if (isAdmin === true) {
Good luck....

Neil Docherty
10,418 PointsCouple of things to note.
You need to use 'else if' and not 'if else'
The variables "isAdmin" and "isStudent" already have boolean values assigned to them. There is no need to check if they are true using (isStudent = true).
The code challenge is picky and doesn't like the capitalisation of 'Student' in your alert message. (This is not a coding error)
Here is the working solution:
var isAdmin = false;
var isStudent = true;
if ( isAdmin ) {
alert('Welcome administrator')
} else if ( isStudent ) {
alert('Welcome student')
}

Douglas Counts
10,126 PointsLOL, you got your answer submitted before mine. Noticed though that you didn't mention that using a single equal sign is actually making an assignment to the variable instead of doing a comparision. Always use 3x equal signs to do a comparison. Making assignments instead of comparisons is one of the top JavaScript errors.

Neil Docherty
10,418 PointsNo need to use the identity operator.
The variables of isAdmin and isStudent are assigned a boolean ( true / false ).
The if statement checks if the condition is true. isAdmin returns false whereas isStudent returns true.
It would be different if the code was set up like this:
var isAdmin = "false";
var isStudent = "true";
if ( isAdmin ) {
alert('Welcome administrator')
} else if ( isStudent ) {
alert('Welcome student')
}
In this situation the variables of isAdmin and isStudent are assigned a string of ( "true" / "false" ). This means that the conditional statement if ( isAdmin ) returns true because isAdmin has a 'real' value (i.e. it's not empty and it's not 0). The alert('Welcome administrator') will execute in this case.
This is where you're quite right in saying that we should use the === identity operator.
if ( isAdmin === "true" ) {
alert('Welcome administrator')
} else if ( isStudent === "true") {
alert('Welcome student')
}
Eric C
7,998 PointsEric C
7,998 Pointsthank you