Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial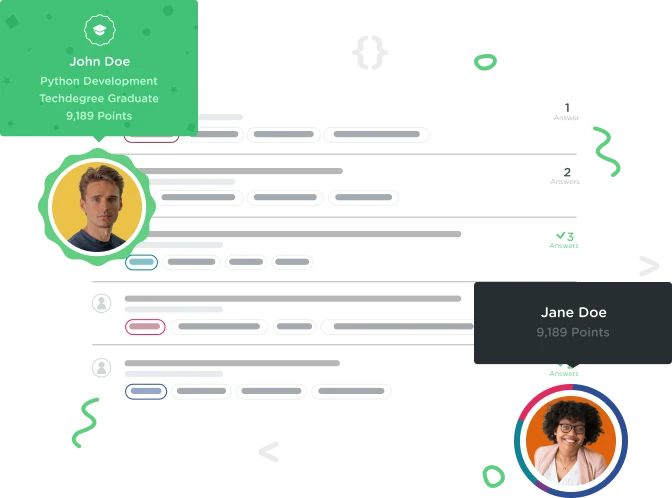

Oliver Primus
2,233 PointsHelp with JavaScript mini-game
Hey everyone!
So I started JavaScript two weeks ago, going through the Full Stack course on here. I feel like I need to go back and start again as I think I'm only getting about 50% retention right now, but that's ok. I wanna get my foundations down properly.
But anyway... I'm building a game for my wife that we usually play in the car. The setup is simple:
Each div looks like this:
<div id="cat" onclick="myCat()" class="col-md-3 col-xs-4 card"> <img src="./resources/images/cat.png"></img> </div>
There's a bunch of those, with each div representing a different value. When a user clicks on it, it updates the total.
I also have another div, which should either double the value of whatever comes next, or double the value of whatever came before it (it doesn't matter either way) and add to the total.
My very beginner JavaScript has this:
var total = 0;
function myCat() { total += 7; document.getElementById("idiot").innerHTML = total; }
My first problem is that I have a different function for every div, so there's a lot of repeating code. If anyone could explain how I cut that down, that would be incredible.
My second problem is that I can't figure out the double value aspect. Again, any help would be amazing!
Thank you
2 Answers
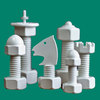
Steven Parker
230,274 PointsHere's a few ideas:
You might modify your function to take an argument:
function addToTotal(val) {
total += val;
document.getElementById("idiot").innerHTML = total;
}
Then, every div
could call the same function but with a different value (7 in this case):
<div id="cat" onclick="addToTotal(7)" ...
Also, note that
img
tags are "self-closing", so there is no such thing as a closing "</img>
" tag.
Then, for doubling the next choice, you could have a boolean (I'll call it "bonus") that is normally false. Then, the div used with it would set it to true:
<div id="doubler" onclick="bonus=true" ...
And then modifying the original function again:
function addToTotal(val) {
total += val;
if (bonus) {
total += val; // add again for double score
bonus = false;
}
document.getElementById("idiot").innerHTML = total;
}
If you also want to be able to double the previous choice, you could add another variable to store the last point value in so you could add that previous value again.
For future postings, remember to use the instructions for code formatting in the Markdown Cheatsheet pop-up below the "Add an Answer" area.
Or watch this video on code formatting.
And if you're using the workspaces, to share the entire project you can make a snapshot of your workspace and post the link to it here.

Oliver Primus
2,233 PointsThis is perfect - thanks so much for your help!