Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial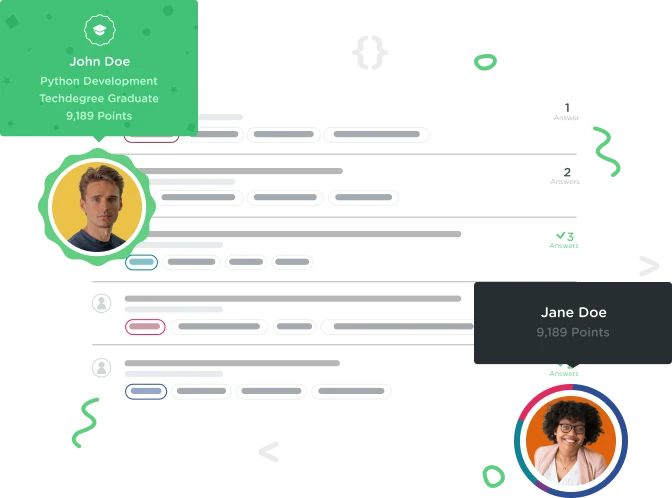
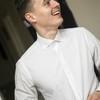
Thomas Hewitt
17,691 PointsHelp with JS and the DOM.
Hi everyone, I'm trying to make a simple to do list using things that I've learned from the JS course so far. I wanted to try to do it with as little help as possible but couldn't get anything to work and when I've gone back through the videos I can see that my code is identical as far as the functions are concerned. The only things I've done differently are things like variable names.
Anyway, I can't seem to get this to work at all. When I click add to list, the list adds an element for a second but that element then disappears.
Would anyone be able to help shed some light on where I'm going wrong? Also, thinking about DRY concepts, would it not make more sense to declare the ul as a global variable or would that not work in this instance?
Thanks
HTML:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>To Do List | JavaScript Practice</title>
<link type="text/css" rel="stylesheet" href="style.css">
</head>
<body>
<div class="container">
<h1>To Do List</h1>
<ul>
<li class="listItem">Add a CSS After property in order to attach some kind of button or image to click on using JavaScript which will give this line the impression that it's done (ie. strikethrough text).</li>
<li class="listItem">Add an input field that allows you to write new things that you want to put in the to do list.</li>
<li class="listItem">Add functionality to the button next to that input field that will then upload that to the dom using the .appendChild(); method.</li>
<li class="listItem">Add functionality to a second button that lets you remove an item from the list depending on what you've typed (for example: ul.removeChild('li:nth-of-type(1)';).</li>
</ul>
<form>
<h3>Add items to the list by typing in the box below and clicking the 'add to list button</h3>
<fieldset>
<input id="input" type="text" placeholder="add to list" name="to do list updater">
<button class="addToList">Add to list</button>
<button class="removeFromList">Remove from list</button>
</fieldset>
</form>
</div>
<script src="script.js"></script>
</body>
</html>
CSS:
body {
background-color: cadetblue;
margin: 0;
padding: 0;
font-family: verdana, sans-serif;
color: aliceblue;
font-size: 1.3em;
}
.container {
width: 80%;
height: 100vh;
margin: 30px auto;
border-left: 10px solid white;
border-right: 10px solid white;
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
border-radius: 10px
}
h1 {
margin: 30px;
font-weight: normal;
}
ul {
width: 80%;
border-top: 10px solid white;
padding-top: 20px;
box-sizing: border-box;
}
form {
width: 80%;
border-top: 10px solid white;
padding-top: 20px;
display: flex;
flex-direction: column;
}
h3 {
font-size: 15px;
font-weight: normal;
text-align: center;
padding: 5px;
margin-bottom: 15px;
}
fieldset {
border: none;
width: 100%;
text-align: center;
}
input {
width: 350px;
height: 35px;
text-align: center;
border-radius: 15px;
border: none;
padding: 10px;
box-sizing: border-box;
}
input:hover{
box-shadow: 1px 1px 2px #888888;
}
input:focus {
outline: none;
border: 3px solid cornflowerblue;
opacity: 1;
}
button {
border: none;
border-radius: 15px;
height: 35px;
margin-left: 30px;
padding: 10px;
box-sizing: border-box;
font-weight: bold;
background-color: aliceblue;
}
button:hover {
background-color: cornflowerblue;
box-shadow: 1px 1px 2px #888888;
}
button:active {
background-color: cornflowerblue;
outline: none;
}
JavaScript:
const input = document.getElementById('input');
const addToList = document.querySelector('button.addToList');
const removeFromList = document.querySelector('button.removeFromList');
addToList.addEventListener('click', () => {
let ul = document.getElementsByTagName('ul')[0];
let li = document.createElement('li');
li.textContent = input.value;
ul.appendChild(li);
input.value = '';
});
removeFromList.addEventListener('click', () => {
let ul = document.getElementsByTagName('ul')[0];
let li = document.querySelector('li:last-child');
ul.removeChild(li);
});
1 Answer
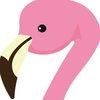
Dave StSomeWhere
19,870 PointsGood move Thomas, learning by doing.
Please check out the Markdown Cheatsheet below for posting your code
Your disappearing issues is happening because you are not preventing the default behavior (read up on the docs).
Try passing the event object to your arrow function (I'm just calling it e
) like below. Also, changed the the second event listener to the remove element variable.
You
addToList.addEventListener('click', (e) => {
e.preventDefault();
let ul = document.getElementsByTagName('ul')[0];
let li = document.createElement('li');
li.textContent = input.value;
ul.appendChild(li);
input.value = '';
});
removeFromList.addEventListener('click', (e) => {
e.preventDefault();
let ul = document.getElementsByTagName('ul')[0];
let li = document.querySelector('li:last-child');
ul.removeChild(li);
});
Thomas Hewitt
17,691 PointsThomas Hewitt
17,691 PointsAmazing, that worked. Thank you so much. I'll need to learn about the preventDefault(); methods then. That wasn't in the section of the course that I was working on and it wasn't included when Guil wrote up his code, but that's okay. I don't mind having more things to learn.
Thanks again and have a Happy New Year
Thomas Hewitt
17,691 PointsThomas Hewitt
17,691 PointsI think I understand now, so the default action of a button is to submit data to the server and because we're using it for something else in this case we need to remove that functionality first in order to leave it as a blank slate so that we can add our new functionality to it.
Thanks again for the help.