Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial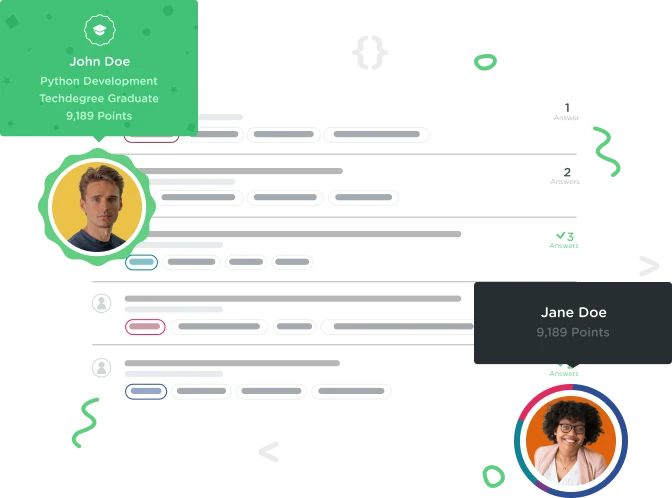

Jake Adam
9,226 PointsHelp with JS code challenge
How do I sort this: var saying2 = ["The", "quick", "brown", "fox", "jumped", "over", "the", "lazy", "dog's", "back"]; by the length of the strings from shortest to largest? It wasn't in the video and I don't know where to start.
2 Answers
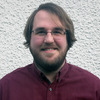
Alex Robertson-Brown
Courses Plus Student 8,956 PointsYeah this one caught me out as well. The answer is a slight modification to one of the examples in the video:
var saying2 = ["The", "quick", "brown", "fox", "jumped", "over", "the", "lazy", "dog's", "back"];
saying2.sort(function(a,b){
return a.length >= b.length;
})
Hope This helps.

Jason Anello
Courses Plus Student 94,610 PointsHi Jake,
The example given in the previous video was to sort numbers.
my_array.sort(function(a, b) {
return a - b;
});
By subtracting the 2 numbers that are passed in you'll be returning either a positive, negative, or zero value which tells the sort method the order of those 2 items. I believe Jim talks about this in the video. You can also read more about the compare function here: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/sort
In this code challenge you want to sort by string length. So two strings from the array will be passed in for a
and b
and you would want to subtract their lengths.
saying2.sort(function(a, b) {
return a.length - b.length;
});

Jake Adam
9,226 PointsThat link really helped me understand the sort function thank you for your help!
Jake Adam
9,226 PointsJake Adam
9,226 PointsThank you it does.
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 PointsHi Alex,
You should always return a positive, negative, or zero value from the compare function. You will get yourself into trouble with returning a boolean value. It may pass this challenge for this very specific array but it's not correct to do this and it will not always work.
For this to work it relies on the sort method being stable. Not all of the browsers implement a stable sort.
One experiment that you can do is run your code above as is in the chrome console and I think you'll find that it does sort correctly although it does not maintain original order of equal length strings.
Then try this code:
Here I've added one more element to make 11 total. It does not sort this correctly. This is because chrome implements a stable sort for 10 elements or less and switches to a unstable sort beyond 10 elements.
The sort method is expecting a positive, negative, or zero value to be returned so you don't want to be returning
true
orfalse
.