Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial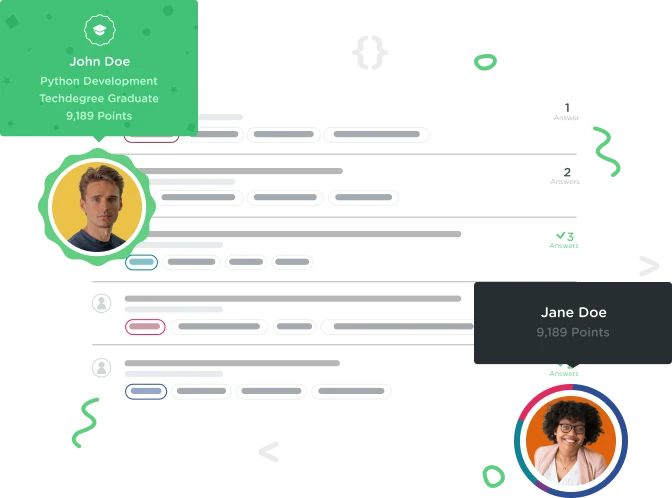
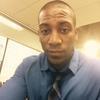
Stephen Gillon
11,996 PointsHelp with JS code. Have no idea what I'm doing wrong.
Working on a quiz that validates the correct answer by color and when dragged to correct category. I also want it to verify when submit is clicked at some point. So far, I got the correct target that I want to change as a test ("AppleTalk") from the initial color to green. However, if I move any button, AppleTalk still turns to green and red doesn't work. Not sure what I'm doing wrong. Thanks! Here's my html/js :
<section id="user-levels">
<h1>Drag and Drop Quiz</h1>
<div id="unassigned" ondrop="dropUser(this, event)" ondragenter="return false" ondragover="return false">
<h2>Choices</h2>
<!--These are all the draggable peices-->
<a draggable="true" class="user" id="NetBEUI" ondragstart="dragUser(this, event)">NetBEUI</a>
<a draggable="true" class="user" id="IP" ondragstart="dragUser(this, event)">IP</a>
<a draggable="true" class="user" id="AppleTalk" ondrop="dropUser(this, event)" ondragstart="dragUser(this, event)">AppleTalk</a>
<a draggable="true" class="user" id="DECnet" ondragstart="dragUser(this, event)">DECnet</a>
<a draggable="true" class="user" id="BGP" ondragstart="dragUser(this, event)">BGP</a>
<a draggable="true" class="user" id="RGIP" ondragstart="dragUser(this, event)">RGIP</a>
<a draggable="true" class="user" id="RIP" ondragstart="dragUser(this, event)">RIP</a>
</div>
<div id="routed" ondrop="dropUser(this, event)" ondragenter="return false" ondragover="return false">
<h2>Layer 3 Routed Protocols</h2>
</div>
<div id="routing" ondrop="dropUser(this, event)" ondragenter="return false" ondragover="return false">
<h2>Layer 3 Routing Protocols</h2>
</div>
<button id="submit" class="sub" onsubmit="mySubmit">Submit</button>
<div class="clear"></div>
</section>
var appleTalk = document.getElementById("AppleTalk");
var routing = document.getElementById("routed");
var routed = document.getElementById("routing");
function dragUser(user, event) {
event.dataTransfer.setData('User', user.id);
}
function dropUser(target, event) {
var user = event.dataTransfer.getData('User');
target.appendChild(document.getElementById(user));
if ( appleTalk === routed ) {
document.getElementById("AppleTalk").style.background = "green";
} else {
document.getElementById("AppleTalk").style.background = "red"
}
}
2 Answers
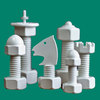
Steven Parker
229,786 Points
Comparing two different elements will never be equal.
But you can compare the id property of the moved element to a constant:
if ( user.id == "AppleTalk" ) {
I made a few other observations:
- the tests could be generalized to work with all the elements
- if you check for unassigned conditions you can also remove the color if the item is "put back"
- black is easier to read on lime than green
I revised dropUser like this:
function dropUser(target, event) {
var protocol = {
NetBEUI: "routed",
IP: "routed",
AppleTalk: "routed",
DECnet: "routed",
BGP: "routing",
RGIP: "routing",
RIP: "routing"
};
var user = event.dataTransfer.getData('User');
var moved = document.getElementById(user);
target.appendChild(moved);
if (target.id == "unassigned") {
moved.style.background = "";
} else if (target.id == protocol[user]) {
moved.style.background = "lime";
} else {
moved.style.background = "red";
}
}
Check the protocol list to be sure I got all the correct answers.
Also, you didn't share your CSS, but I found this nice while testing:
a[draggable] {
cursor: pointer;
margin: 2px;
}
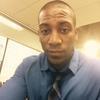
Stephen Gillon
11,996 PointsThanks so much for you input Steven Parker and Erik Nuber !
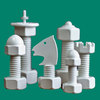
Steven Parker
229,786 PointsHappy to help, and it was fun for me as I had not worked with draggable items before!
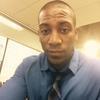
Stephen Gillon
11,996 PointsGot it, thank you again Steven Parker !
Erik Nuber
20,629 PointsErik Nuber
20,629 PointsInteresting project.
I played with it a little in codepen
just a couple of notes.
I am not sure if it is for a reason but you assign routing to routed and routed to routing in your variable declarations.
var routed = document.getElementById("routing");
Also, as you assign appleTalk to AppleTalk ID you can just use
appleTalk.style.backgorund = "red" //or green"
The reason that this is not working the way you want is that your comparison will never be true. You get
var routed = document.getElementById("routed");
This means routed is now equal to
Then when appleTalk is dragged to routed you are comparing if the above is equal to appleTalk. appleTalk is this
<a draggable="true" class="user" id="AppleTalk" ondrop="dropUser(this, event)"
so it will never turn green.
You can check what each holds in the console and, you may need to add a class or something upon dragging that you can check for and then to a test for true/false to assign green and red.
edit: forgot about why it turns red all the time. The other issue is that every time something is being dragged, hyou send it to the function dropUser and within that the only comparison being made is with appleTalk so no matter what you drag, that comparison gets made and since it will always be false it is turning red.