Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial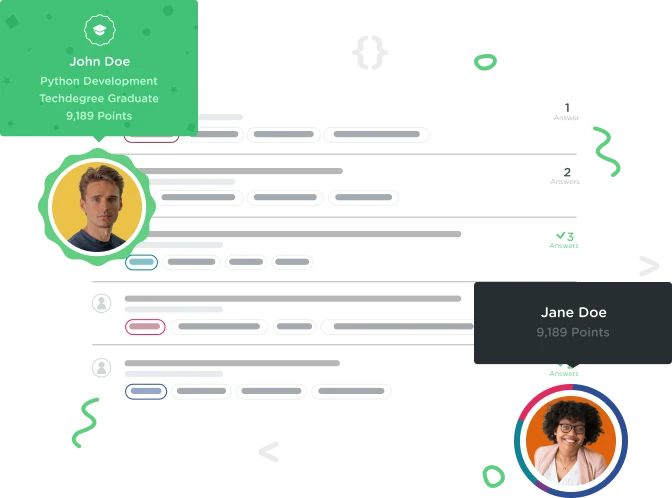

martin li
1,026 Pointshelp with lab
- (The Stock class) Design a class named Stock that contains:
A string data field named symbol for the stock's symbol.
A string data field named name for the stock's name.
A double data field named previousClosingPrice that stores the stock price for the previous day.
A double data field named currentPrice that stores the stock price for the current time.
A constructor that creates a stock with specified symbol and name.
The accessor methods for all data fields.
The mutator methods for previousClosingPrice and currentPrice.
A method named changePercent() that returns the percentage changed from
previousClosingPrice to currentPrice.
Draw the UML diagram for the class. Implement the class. Write a test program that creates a Stock object with the stock symbol NYSE:IBM, the name International Business Machines Corporation, and the previous closing price of 100. Set a new current price to 90 and display the price-change percentaage
1 Answer
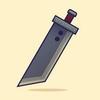
Allan Clark
10,810 Points"Data field" is the same as "member variables" in TreeHouse lingo. An example from the videos would be the character for the Pez dispenser. Not sure what the exact name was but it was something like "mCharacter."
The constructor method has the same name as the class and has no return tag. For your example it would be something like:
public Stock(String symbol, String name) {
mSymbol = symbol;
mName = name;
}
Accessors just a fancy name for getters. The getName() method should return the String mName. Similar with mutators, just means a method that changes the value of the member variable. In this example setSymbol(String foo) method should return nothing but set mSymbol to foo. Here is the oracle documentation on Class structure: https://docs.oracle.com/javase/tutorial/java/javaOO/classes.html
Unified Modeling Language is out of scope of this course but is really easy to pick up. It is visual representation of classes and how they interact. This has to be done with special software, I personally use Dia: http://sourceforge.net/projects/dia-installer/
Also here is a quick tutorial on UML: https://www.youtube.com/watch?v=OkC7HKtiZC0
Don't let the jargon discourage you, programmers like to use fancy names for things, some prefer certain fanciness over others.
Hope this helps!
Nicolas Hampton
44,638 PointsNicolas Hampton
44,638 PointsWhere did you find this project or class?