Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial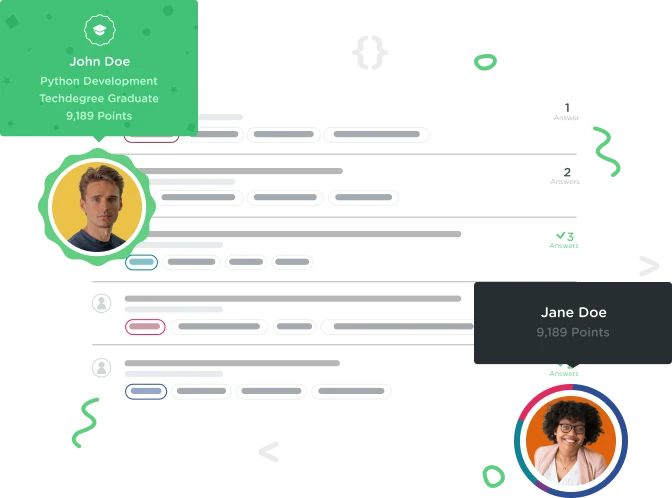

meitav asulin
1,123 Pointshelp with mission
why it is wrong
func fizzBuzz(n: Int) -> String {
// Enter your code between the two comment markers
switch n {
case n % 3 == 0 : return "Fizz"
case n % 5 == 0 : return "Buzz"
case n % 5 == 0 && n % 3 == 0 : return "FizzBuzz"
default : return (n)
}
// End code
return "\(n)"
}
3 Answers
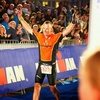
Steve Hunter
57,712 PointsHi there,
Do the double-test first. Otherwise, your FizzBuzz candidates will all get filtered off at the first line as n % 3 == 0
.
Make sense?
Steve.
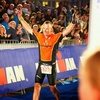
Steve Hunter
57,712 PointsAlso, if you're using a switch
statement, you aren't switch
ing on n
. You are looking for a true
outcome, i.e. you want n % 3 == 0
to evaluate to true
else that value of n
is disregarded. So switch
on true
then put your cases in as you have done - but think about the order, as in my other answer. Do the double test first. Something like:
switch true {
case n % 3 == 0 && n % 5 == 0:
return "FizzBuzz"
The challenge is expecting you to use multiple if
and else if
statements, as below. That may be the easier way forward. But both are valid solutions.
if n % 3 == 0 && n % 5 == 0 {
return "FizzBuzz"
I hope that helps,
Steve.
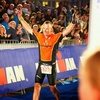
Steve Hunter
57,712 PointsAlternatively, to switch
on n
, use a where
clause like:
switch n {
case _ where n % 5 == 0 && n % 3 == 0:
return "FizzBuzz"

meitav asulin
1,123 Pointsthe value we consider is a name like func name or it is a value that must have some activity (function, true, false...) ?
thanks for help
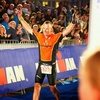
Steve Hunter
57,712 PointsThis way won't work:
switch n {
case n % 3 == 0: // do something
}
because the case
evaluates to a true
value boolean whereas n
is an integer. So, the case will never be met as an integer isn't a boolean.
So you switch on the outcome you want to compare, which is n % 3 == 0
evaluating to true
. So, here:
switch true {
case n % 3 == 0: // do something
}
will pull out all examples where n % 3 == 0
is true
and disregard the rest which is precisely what we want!
I think if you're using a switch
to check for a true value, maybe an if
statement is deemed more appropriate. The outcome is binary, true
or false
, so given that there are only three tests being made, perhaps three if
statements are simpler? That's down to a matter of preference. While I think if
would be the more purist solution, I'd go down the switch
route too just because adding further conditions is easier and lots of if
statements lack clarity.
Make sense?
Steve.
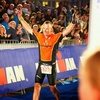
Steve Hunter
57,712 PointsIf you like complicated solutions, you could use tuples. This makes the order irrelevant as the cases are unique so doing the single tests first wouldn't filter out the double-test candidates.
However, this is horrible code - it doesn't read well at all! Complicated switch
conditions aren't helpful. There are clearer ways of doing this, as above but I thought I'd mention it just to demonstrate how badly wrong things can go if you don't keep it simple!
switch (n % 3 == 0, n % 5 == 0) {
case (true, false):
return "Fizz"
case (false, true):
return "Buzz"
case (true, true):
return "FizzBuzz"
default:
return("\(n)")
}