Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial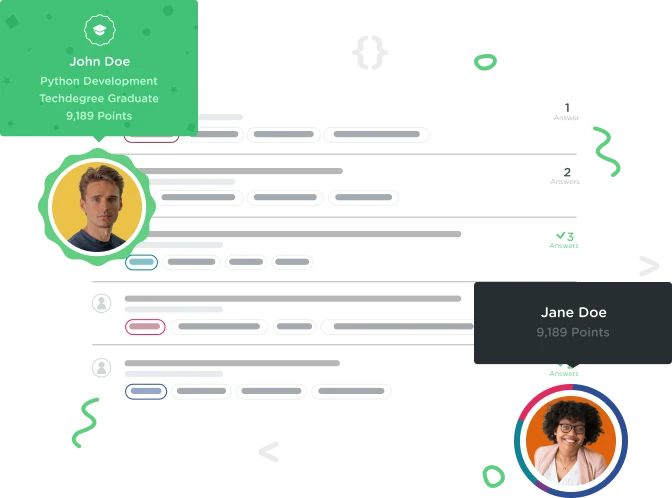

Abid Rather
4,719 PointsHelp with Morse.py. Appreciate the help
Problem is to convert, for instance, "dash-dot" string to ["_" , "."] by defining a class method from_String. My static method does not seem to work. Appreciate your help in pointing out whats wrong with my code
class Letter:
def __init__(self, pattern=None):
self.pattern = pattern
def __iter__(self):
yield from self.pattern
def __str__(self):
output = []
for blip in self:
if blip == '.':
output.append('dot')
else:
output.append('dash')
return '-'.join(output)
@staticmethod
def from_string(cls, string_arg):
output = []
for word in string_arg.split('-'):
if word == "dash":
output.append('_')
else:
output.append('.')
return cls(','.join(output))
class S(Letter):
def __init__(self):
pattern = ['.', '.', '.']
super().__init__(pattern)
1 Answer

andren
28,558 PointsThere are two issues in your code:
- For this method you should use the
@classmethod
annotation, not @staticmethod. The difference between them is that with a class method Python passes the class as the first argument automatically, with a static method the class is not sent automatically. - The
Letter
constructor takes an array as its argument, but you are passing it a string since you usejoin
on the array you create. There is no need to convert the array before passing it to the constructor.
If you fix those two issues like this:
class Letter:
def __init__(self, pattern=None):
self.pattern = pattern
def __iter__(self):
yield from self.pattern
def __str__(self):
output = []
for blip in self:
if blip == '.':
output.append('dot')
else:
output.append('dash')
return '-'.join(output)
@classmethod # @staticmethod changed to @classmethod
def from_string(cls, string_arg):
output = []
for word in string_arg.split('-'):
if word == "dash":
output.append('_')
else:
output.append('.')
return cls(output) # Don't turn the array into a string
class S(Letter):
def __init__(self):
pattern = ['.', '.', '.']
super().__init__(pattern)
Then your code will work.
Abid Rather
4,719 PointsAbid Rather
4,719 PointsAbsolutely. Thanks for your help Andren!