Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial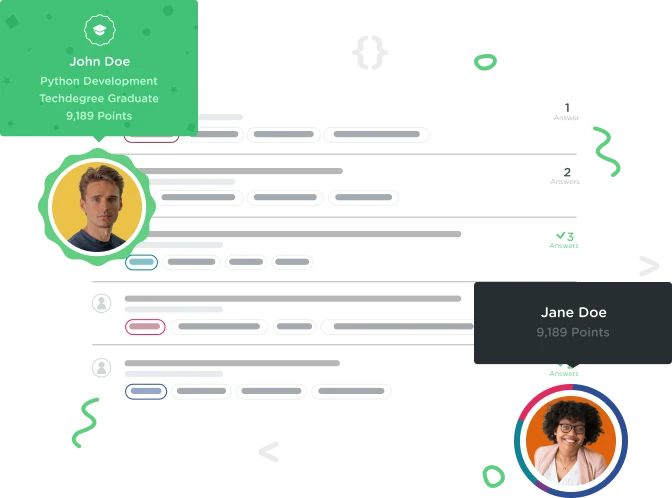

Daniel Villanueva
12,329 PointsHelp with my code!
Alright, I need you make a new method named feedback. It should take an argument named grade. Methods take arguments just like functions do. You'll still need self in there, though.
If grade is above 50, return the result of the praise method. If it's 50 or below, return the reassurance method's result.
class Student:
name = "Your Name"
grade = 0
def praise(self):
return "You inspire me, {}".format(self.name)
def reassurance(self):
return "Chin up, {}. You'll get it next time!".format(self.name)
def feedback(grade, self):
if self.grade > 50:
return praise(self.name)
else:
return reassurance(self.name)
3 Answers
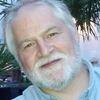
Jeff Muday
Treehouse Moderator 28,720 PointsPretty close-- but you need some minor fixes.
On the feedback, you need self as the first parameter rather than grade.
When you test the grade, you should not be referencing "self.grade" but just the "grade" parameter
When a class calls/executes its own function, you need to add self. See below:
class Student:
name = "Your Name"
def praise(self):
return "You inspire me, {}".format(self.name)
def reassurance(self):
return "Chin up, {}. You'll get it next time!".format(self.name)
def feedback(self, grade):
if grade > 50:
# call the self.praise method
return self.praise()
else:
# calling reassurance method
return self.reassurance()
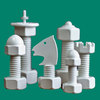
Steven Parker
231,269 PointsYou have the right idea, but a few implementation issues:
- the "self" parameter should be the first thing in the parameter list
- since "grade" is a parameter, it won't need a "self." prefix
- but the methods you are calling will need a "self." prefix
- neither method takes an argument

ERDAL DINCER
1,635 PointsCAn`t we assign after name as variable such as grade=random(1,100) ?
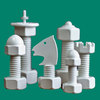
Steven Parker
231,269 PointsAssigning a grade isn't part of this challenge, only using one that has already been set to create a response.

ERDAL DINCER
1,635 PointsWhat is wrong with this code ?Can't I define grade after name with random function?
import random class Student:
name = "Your Name"
grade=random(1,100)
def praise(self):
return "You inspire me, {}".format(self.name)
def reassurance(self):
return "Chin up, {}. You'll get it next time!".format(self.name)
def feedback(self, grade):
if grade > 50:
# call the self.praise method
return self.praise()
else:
# calling reassurance method
return self.reassurance()
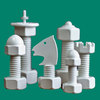
Steven Parker
231,269 PointsThat's not what the challenge is asking you to do.