Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial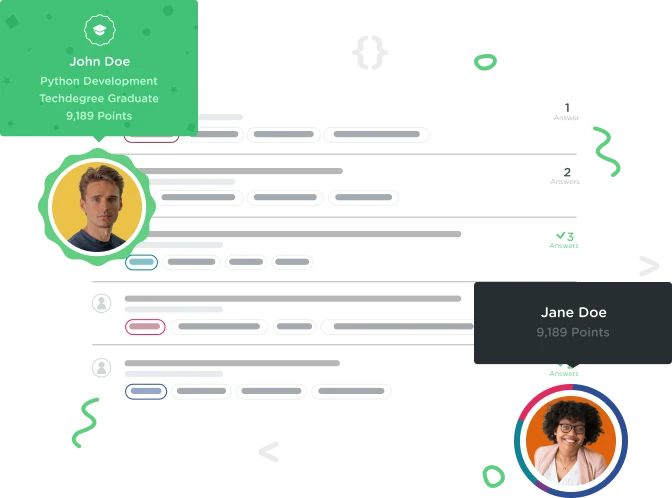
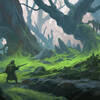
Caleb Newell
12,562 PointsHelp with my JS Promise code
My code, when the button is pushed, is supposed to retruve back all of the current astronoughts in space, but I keep getting the error, "Uncaught ReferenceError: response is not defined at HTMLButtonElement.<anonymous> (promises.js:37)" and I can't figure out what it means. Can anyone help me?
here is my code,
const astrosUrl = 'http://api.open-notify.org/astros.json';
const wikiUrl = 'https://en.wikipedia.org/api/rest_v1/page/summary/';
const peopleList = document.getElementById('people');
const btn = document.querySelector('button');
function getProfiles(json) {
const profiles = json.people.map( person => {
const craft = person.craft;
return fetch(wikiUrl + person.name)
.then( response = response.json() )
.then (profile => {
return {...profile, craft};
})
.catch( err => console.log('Error fetching Wiki', err) )
});
return Promise.all(profiles);
}
function generateHTML(data) {
data.forEach( person => {
const section = document.createElement('section');
peopleList.appendChild(section);
section.innerHTML = `
<img src=${data.thumbnail.source}>
<span>${person.craft}</span>
<h2>${person.title}</h2>
<p>${person.description}</p>
<p>${person.extract}</p>
`;
});
}
btn.addEventListener('click', (event) => {
event.target.textContent = "Loading...";
fetch(astrosUrl)
.then( response = response.json() )
.then(getProfiles)
.then(generateHTML)
.catch( err => {
peopleList.innerHTML = '<h3>Something went wrong!</h3>';
console.log(err)
})
.finally( () => event.target.remove() )
});
1 Answer
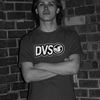
Martin Sole
82,199 PointsHi
Where you response = response.json(), this should be an arrow function response => response.json()