Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial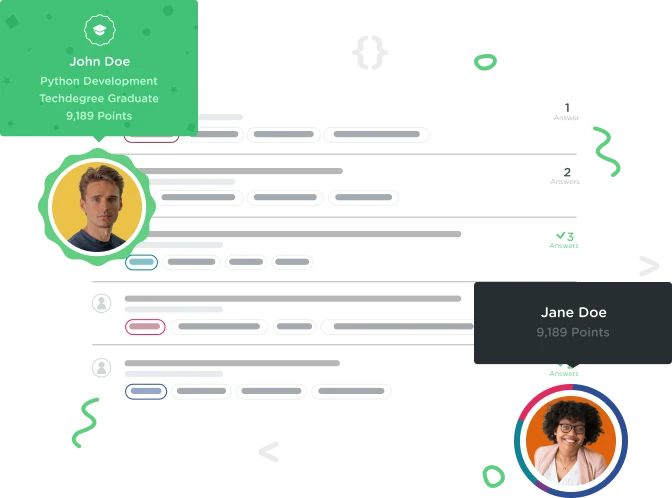
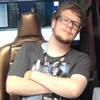
Felipe Belinassi
1,567 PointsHelp with my solutions
This my two solutions to the challenge (one user input and two user inputs).
One input:
var userNumber = prompt("Hey! Type a number");
userNumber = parseInt(userNumber);
var number = Math.floor(Math.random() * userNumber) + 1;
alert("You picked " + userNumber + ". Your random number from 1 to " + userNumber + " is " + number);
Two inputs:
var minNumber = parseInt(prompt("Type a number!"));
var maxNumber = parseInt(prompt("Now type a higher number!"));
var random = Math.floor(Math.random() * maxNumber) + minNumber;
alert("You picked " + minNumber + " and " + maxNumber + ". Your random number from " + minNumber + " to " + maxNumber + " is " + random);
I don't know if my solution to one input is right. But i'm sure my solution for two inputs is incorrect (sometimes the random number is bigger than the user maxNumber input). Could someone help me?
1 Answer
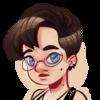
Alexandra Wakefield
1,866 PointsThe mishap in your Two Input is with the line
var random = Math.floor(Math.random() * maxNumber) + minNumber;
Instead of using the minNumber as a base, which could be anything, you are using it as an additive to whatever random number is created.
Example: Let's say you entered 2 as your minNumber, 6 as your maxNumber and Math.random generates 0.9. This creates the equation:
(0.9 * 6) + 2 = 7.4
Math.floor rounds this down to 7. As you can see, this is happening because there is no actual base and you're instead using the minNumber as a replacement for the +1, which is there to raise the (Math.random() * maxNumber) to 1 if Math.random decides to equal 0.
Well then, what can you do? Doug gives a great solution, I just moved the + 1 into the maxNumber and minNumber's parenthesis to ensure that if the number generated by Math.random is 0 the minNumber added to it will not be +1 higher than the our wanted minimum:
var random = Math.floor(Math.random() * (maxNumber - minNumber + 1)) + minNumber;
What This Solution Does
- has minNumber act as a base
- + 1 is there in case Math.random equals 0 as well as to help reach the maxNumber
- the + minNumber ensures that the maxNumber can be reached while a number under minNumber cannot
Example: Let's use the same numbers as before.
(0.9 * (6 - 2 + 1)) + 2 = 6.9 = 6
If our lowest is 0:
(0 * (6 - 0 + 1)) + 0 = 0
Doug Hantke
10,018 PointsDoug Hantke
10,018 Pointsvar random = Math.floor(Math.random() * (maxNumber - minNumber) +1 ) + minNumber;
I am obviously not a teacher, but that is the part that is creating the higher random number. Edited out a terrible explanation of how this works