Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial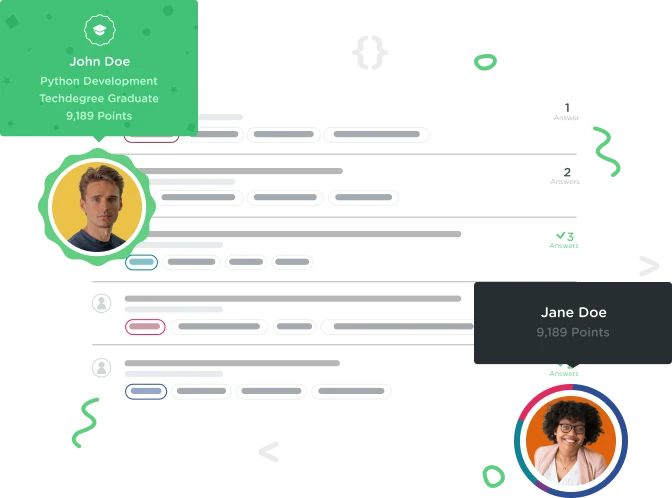

Tobi Ogunnaike
2,242 PointsHelp with Parse Error ?? Thanks
The challenge is to create a function that accepts two numbers, checks which number is larger and then returns that.
I guessed that the ParseInt () function would be needed to convert the values in my parameters 'smaller' and 'larger' into numbers that the browser can do math with (i.e. compare which is bigger). Is this necessary??
Then I wrote a conditional statement in order to compare these two numbers in order to make the function return only the larger one.
But it's not quite working out...
Where have I gone wrong?
Thanks!!
function max('smaller', 'larger') {
if parseInt( (larger > smaller)) {
return larger ;
} }
2 Answers
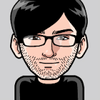
Alexandru Doru Grosu
2,753 PointsYour way of thinking is on the right track, but you have quite a few mistakes, so I will go line by line.
function max('smaller', 'larger')
Here, instead of declaring two arguments (and that's just a fancy name for a variable used in the context of the ()
s of a function) in the signature of the functions, you are actually creating the strings 'smaller' and 'larger'.
So to fix, the declaration would have to be
function(smaller, larger)
Next up
if parseInt( (larger > smaller))
Three mistakes here. First of all, an if
statement is always followed by ()
. This is where your condition goes. It is called a condition because it needs to boil down to either true
or false
(note: in JavaScript there is the concept of truthy and falsy, but that is beyond the scope of your question, and I recommend you look into it yourself - also it does not influence your code in any way).
So you would have to move your parseInt
inside ()
s.
Second of all, parseInt
takes a string
as an argument, while your expression
(larger > smaller)
will always evaluate to a boolean. So instead of passing string
to parseInt
you will always be passing a boolean, which it cannot parse.
Third and last, parseInt
can only parse one string at a time (the second, optional, argument is radix, which is used to define the base in which you want to parse the number in - by default it will be base 10, which is what you and me use to count so you don't have to worry about it for now). So you will need to turn your code into two calls to parseInt
like so (note this is a combination of all 3 suggestions)
if(parseInt(larger) > parseInt(smaller)) {
// ...
}
Lastly, there is one last thing you have to fix, and I will leave that to you, however I will give you a hint. Right now you are returning the larger
number if it indeed has a bigger value than smaller
. But what happens if smaller
has a bigger value than larger
?
e.g. I am calling your function like this:
max(7,10)
where larger
takes the value 7 and smaller
will take the value 10.

Tobi Ogunnaike
2,242 PointsThanks!!
I need to add an 'else' clause to return 'smaller' if smaller has a bigger value than larger?
Like this?
function max(larger, smaller) {
if(parseInt(larger) > parseInt(smaller)){
return parseInt(larger);}
else {return parseInt(smaller)}
}