Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial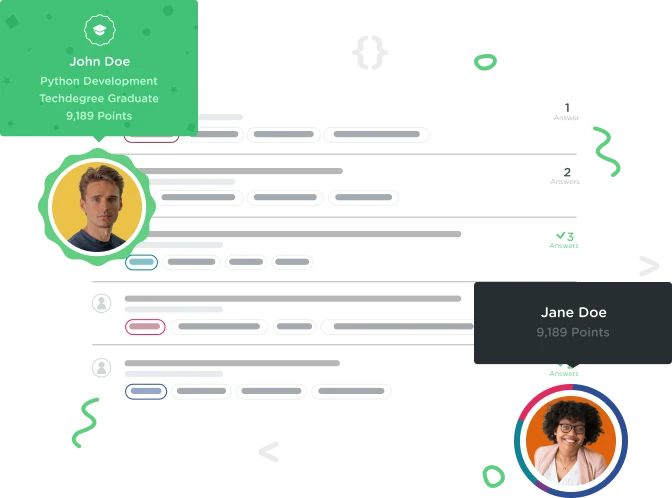
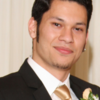
Michael Kempf
1,800 PointsHelp with return values challenge
http://teamtreehouse.com/library/return-values
Can't seem to get my code to work, this is my current code:
function arrayCounter (array){
if (typeof array === 'string', 'number', 'undefined'){
return 0;
} else {
return array.length;
}
}
5 Answers

Giovanni CELESTE
20,961 Points(erf, my previous answer disapeared, wrong manipulation). Your if comparison is the same as writing if ("undefined") {} because the comma in Javascript return the last operand (in your case, "undefined"). You should try another structure (like if...else if...else).

Knut Ringheim Lunde
38,811 PointsIf you have to check for multiple conditions you have to use the or-operator ( || ) to achieve your desired result. For example:
var n = 10;
if( n < 9 || n * n > 99 ) {
// Do something..
}
I tried it in the code engine, but it said I hadn't defined a function called arrayCounter, so that didn't work, but it should (I think)..
EDIT: I had written “array typeof === "string" (etc ..)” instead of “typeof array === "string" (etc...)”, it worked after I corrected it. And, you could also use a single | to represent the or-operator (see Giovanni's post). Thanks for the enlightenment, Giovanni! :)
But, another way, and maybe a better way too, is to check if the argument is an instance of Array (note capital “a”). Like this:
function arrayCounter(array) {
if(array instanceof Array) {
return array.length;
} else {
return 0;
}
}

Giovanni CELESTE
20,961 PointsHi Knut, in some other languages you use ||, in javascript you use the single | (then it will work).
function counterArray(array) {
if (typeof array ==="string" | typeof array ==="number" | typeof array==="undefined") {
return 0 ;
} else {
return array.length ;
}
}
Another option would be the if...else if...else...
function counterArray(array) {
if (typeof array ==="string") {
return 0 ;
} else if (typeof array ==="number") {
return 0 ;
} else if (typeof array ==="undefined") {
return 0 ;
}
else {
return array.length ;
}

Giovanni CELESTE
20,961 PointsWell, I don't know how to format my code like you all, if you could tell me ^^, my answers would be clearer.
EDIT: ok got it :), now it's much clearer.
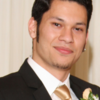
Michael Kempf
1,800 PointsThank you both so much for your help!! It is extremely frustrating being stuck and having no idear what went wrong for hours on end. Knut Ringheim Lunde the "instanceof Array" method worked but I'm not positive on how it does work. Also I tried the "||" and "|" operator out but neither worked until I changed the format I had to look like Giovanni's code if (typeof array ==="string" | typeof array ==="number" | typeof array==="undefined") Giovanni CELESTE is that the shortest way possible to write that line of code? It seems to me that its a little repetitive, regardless your method worked best for me because I can understand it fully. Again thank you both much

Giovanni CELESTE
20,961 PointsI may be wrong, but yes, I think it's the shortest you can do. The "shortest" means that you write only one condition (+ and/or) for the same result, but your condition can be quite long, if you make it complex with multi-layer conditions.

Knut Ringheim Lunde
38,811 PointsCheck out the following code snippet:
var arr_1 = [1, 2, 3, 4];
var name = "John Doe";
if( arr_1 instanceof Array ) {
console.log("This will be written since arr_1 is an instance of the object prototype Array");
}
if( name instanceof Array) {
console.log("This will not be written since the variable name is not an instance of the object prototpye Array");
}
Since arr_1 is an instance of the Array-object, the instanceof-operator returns true. The name variable, on the other hand, is not an instance of the Array-object, thus the instanceof-operator returns false.
Check out more examples for instanceof here: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/instanceof
Michael Kempf
1,800 PointsMichael Kempf
1,800 PointsI am sorry but I am still unable to understand. Maybe it's because I am still brand new at this but could you please show me the correct way to write my code and explain why that would work vs what I have already written. I'v been stuck on this for a couple of hours now and "googling" isnt helping my case much...