Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial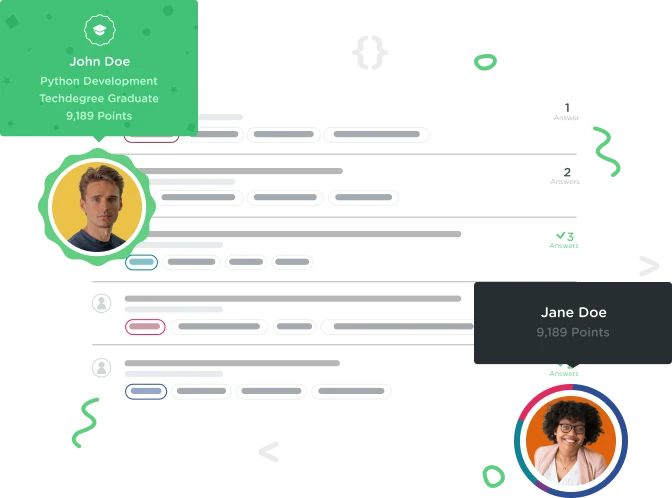
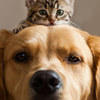
Devin Scheu
66,191 PointsHelp With Ruby
Question: Fill in the find_index method to return the index of a todo item in the @todo_items array given the name. The method should return the index of the item if the item is found and nil if it is not found.
My Code:
class TodoList
attr_reader :name, :todo_items
def initialize(name)
@name = name
@todo_items = []
end
def add_item(name)
todo_items.push(TodoItem.new(name))
end
def find_index(name)
index = 0
found = false
todo_items[name]
end
end
6 Answers

Jason Seifer
Treehouse Guest TeacherStone Preston Devin Scheu The code looks like it would work but it's a bit deceiving! If we were going for an index of the String
"name" in the todo_items
array, then the index
method would work. However, when we add an item to the todo_items
array, in the add_item
method, we're creating a new TodoItem
with that information. The index
method looks for equality in an array and a string instance is not going to be equal to a TodoItem
instance. Therefore, we need to figure out how to find TodoItem
in the todo_items
array.
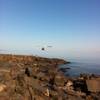
ttaleg
9,830 Pointsdid anyone figure this out? I cant either
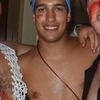
Peter Zurkuhlen
6,277 PointsI answered with the following code and it worked (basically the same as what was done in the preceding video lessons):
def find_index(name)
index = 0
found = false
todo_items.each do |todo_item|
if todo_item.name == name
found = true
end
if found
break
else
index += 1
end
end
if found
return index
else
return nil
end
end
Took me a few tries to actually get it right - with this challenge, there are a lot of small moving parts, so it's important to do a thorough check and de-bug. I noticed that mine wasn't passing for a few tried simply because I had an extra "end" at the bottom, which was throwing the whole thing off.
I think Treehouse should at least give the option for some sort of answer key to the challenge problems, so that getting stuck on something like this doesn't completely derail progress. It can be very discouraging, and waiting days, weeks, or longer for an answer definitely ain't good for getting into a coding groove. Just a thought - love the program/teachers, and the content and delivery is great!!
Thanks and good luck! -Peter *loyal fan and user
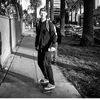
Joshua Paulson
37,085 PointsLove when people copy paste code that doesn't work when they say it worked for them. Really helpful.

Stone Preston
42,016 Pointslooking at the ruby array documentation there is a method called index you can use.
Returns the index of the first object in self such that the object is == to obj. If a block is given instead of an argument, returns index of first object for which block is true. Returns nil if no match is found
so you should be able to use that easily
def find_index(name)
todo_items.index(name)
end
However, the challenge does not accept that for some reason. Jason Seifer any idea why that does not work?
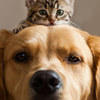
Devin Scheu
66,191 PointsOkay so I changed the code to and if statement, and used the concept you showed me. But im still getting it wrong, I often get confused between the syntax's of different languages. So what am I doing wrong,.
def find_index(name)
index = 0
found = false
if (index > 0)
todo_items.find_index(name)
else
nil
end
end

Alex Ignat
8,538 PointsCan anyone else help with this question please? I cannot figure it out either.

Vlad Filiucov
10,665 Pointsi don't get this part
if todo_item.name == name
found = true
Where does this ".name" method come from?
Devin Scheu
66,191 PointsDevin Scheu
66,191 PointsYes, thanks for clearing it up for me Jason. :)