Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial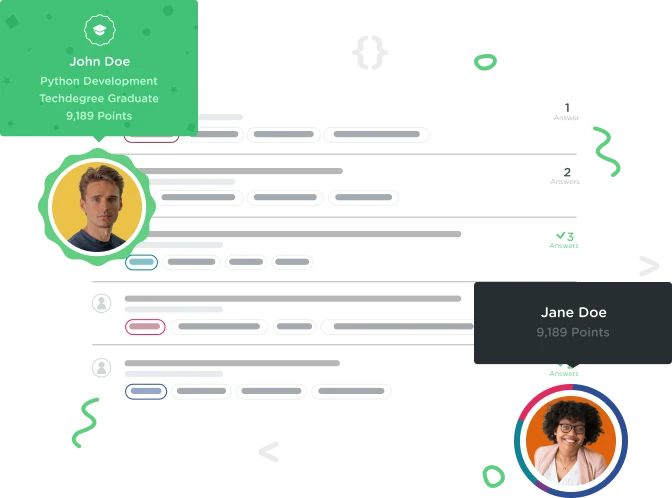
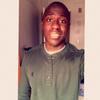
Allan Oloo
8,054 PointsHelp with search function for the students object.
function searchReport() {
var studentName = students[i];
while(true) {
var searchName = prompt('Type a name of a student or press \"quit\" to end the search!');
if (searchName === 'quit'){
break;
}
}
for (var i = 0; i < studentName.length; i++){
if(searchName == studentName.name) {
message += '<h2>Student: ' + studentName.name + '</h2>';
message += '<p>Track: ' + studentName.track + '</p>';
message += '<p>Points: ' + studentName.points + '</p>';
message += '<p>Achievements: ' + studentName.achievements + '</p>';
} else {
alert('Name could not be found on the report')
break;
}
}
}
searchReport();
print(message);
My search function is not printing out the name the user has requested. I do not know if my logic is the issue. Can someone please help me get to the solution. Thank you!
2 Answers

Umesh Ravji
42,386 PointsHey there Allan, looks like you have a bit of work to do with this one :)
var studentName = students[i];
It doesn't make much sense to do this. Your loop should be looping over the entire students list, not trying to loop over the first item.
for (var i = 0; i < studentName.length; i++){
// code removed
}
Your for loop should be inside your while loop. In your code the while loop continuously executes the prompt until it receives 'quit' and then attempts to find a student through the loop.
// For my response, you can assume the loop is over the students array
for (var i = 0; i < studentName.length; i++){
if(searchName == studentName.name) {
message += '<h2>Student: ' + studentName.name + '</h2>';
message += '<p>Track: ' + studentName.track + '</p>';
message += '<p>Points: ' + studentName.points + '</p>';
message += '<p>Achievements: ' + studentName.achievements + '</p>';
} else {
alert('Name could not be found on the report')
break;
}
Your loop isn't quite correct. If the name is found, it will actually keep looping and not break out of it once the name is found. If the name is not found on the first loop, it will break, and not actually keep searching.
message += '<h2>Student: ' + studentName.name + '</h2>';
This actually causes an error because message is not defined. You haven't created it anywhere yet, and the best place to do it would be before the for loop:
var message = '';
for(var i = 0...
print(message);
This method should be called from inside your searchReport function. The print method also seems to not part of the initial code, and is something you have to write yourself.
Here's more of a skeleton of what I would do based on your approach, feel free to try using this. If you need anymore help just ask.
function searchReport() {
while(true) {
var searchName = prompt('Type a name of a student or press \"quit\" to end the search!');
if (searchName === 'quit'){
break;
}
var message = '';
for (var i = 0; i < students.length; i++) {
var student = students[i];
if(searchName == student.name) {
// build message here
break;
}
}
if (message == '') {
alert('Name could not be found on the report')
}
}
}
searchReport();

Umesh Ravji
42,386 PointsRemember to set the message variable before the loop, otherwise the concatenation will cause an error. Lets take a look at how that loop runs.
First, lets say the user enters 'Dave':
1st iteration: first student is Dave, there is a match.
if condition checked, since there is a match, no alert
2nd iteration: secound student is Jody, there is no match
if condition checked, and 'Name not found alerted', then break
You see the loop finds the student correctly, but then it keeps searching because you don't break out of the loop once the user is found.
Next, lets say the user enters 'Trish':
1st iteration: first student is Dave, there is no match, and 'Name not found alerted', then break
Here, the loop never even continue's past the first student.
There's probably a million different ways to handle this problem, but the way I chose made sense to me. It makes sure the entire loop is run, then checks the message variable at the end. If message is an empty string, it knows no name was found and the user can be alerted.
var message = '';
for (var i = 0; i < students.length; i++) {
var student = students[i];
if(searchName == student.name) {
// build message here
break;
}
}
if (message == '') {
alert('Name could not be found on the report')
}
In my example, lets enter 'Jordan':
1st iteration: student is Dave, no match
2nd iteration: student is Jody, no match
3rd iteration: student is Jordan, build message string, then break
Then check message variable
As to becoming proficient with JavaScript, I wouldn't be able to say. I enjoyed programming here and there, but it wasn't my my first choice of study, but then returned to it later on. I was really bad to start with, but sticking to it and practicing with own projects helps a lot.
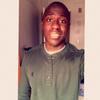
Allan Oloo
8,054 PointsThank you for your help. You have cleared up a lot questions I had. I will continue hacking away. My problem is I understand the concepts and syntax. But when you give me a challenge or algorithm to solve I always struggle with it. I have been focusing on JS since october. I hope this new year by the June I will be able to get somewhat proficient to be able to get junior developer job. Thanks again!
Allan Oloo
8,054 PointsAllan Oloo
8,054 PointsHello Umesh, I was confused with the last if statement. would it better to just add an else statement? If the name doesn't exist go to the else statement alert?
So my else statement is not functioning well. Every time i put a name that doesn't exist it keeps alerting over and over. I had to add the break; because it kept iterating over and over. Also even when I put the right name it still alerts me "name not found". Any suggestions? Also how long did it take you to be become proficient in javascript?