Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial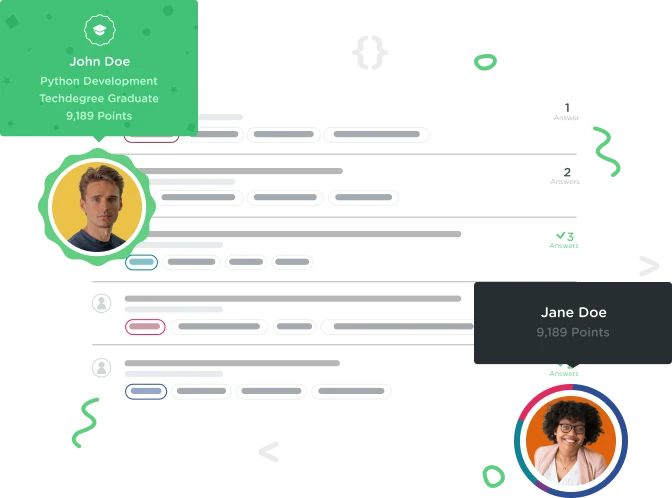

Steven Wheeler
2,330 PointsHelp with sorting dictionaries?
I am stuck on the Python challenge: "Create a function named stats that takes a dictionary of teachers and returns a list of lists in the format: ["name", "number of classes"]
My existing code is here, any advice on how to start thinking about it will be appreciated.. I honestly can't think how to go about doing it:
teachers = {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
'Kenneth Love': ['Python Basics', 'Python Collections']}
def most_classes(teachers):
max_count = 0
max_teacher = ''
for key in teachers:
class_count = len(teachers[key])
if class_count > max_count:
max_teacher = key
max_count = class_count
return max_teacher
def num_teachers(teachers):
teacherNumber = 0;
for teacher in teachers:
teacherNumber += 1
return teacherNumber
def stats(teachers):
2 Answers

Ron Fisher
5,752 PointsSteven,
Since the results will be a list you will need an empty list to append the results to.
myList = []
Since you will be checking all teachers and their classes you need a loop:
for teacher in passed_in_dictionary:
You also need to count all the classes for each teacher and append the results to the list:
myList.append([teacher,len(passed_in_dictionary[teacher])])
And then return the results. Does that help?
Ron

Ron Fisher
5,752 PointsSteven,
len() is a function and should be len(teachers[item])
Ron

Steven Wheeler
2,330 PointsI swear this challenge was designed by Satan (sorry Kenneth).
The final task is: "Write a function named courses that takes the dictionary of teachers. It should return a list of all of the courses offered by all of the teachers."
Easy right?... So I did:
teachers = {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
'Kenneth Love': ['Python Basics', 'Python Collections']
def courses(teachers):
for item in teachers.values():
print item
Other code omitted for ease of reading.
And nope, it gives me this error: You returned 4 courses, you should have returned 18.
I can list it nicely with each teacher's name and showing all their courses or whatever but apparently I'm meant to be returning "18" courses.
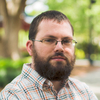
Kenneth Love
Treehouse Guest TeacherAll Hail Ken-- I mean, wow, sorry about that.
Yes, these are challenging challenges, but they're also fairly real world challenges. You'll work with dictionaries all the time in Python and you really need to know how to manipulate them to whatever ends you want.
Remember, too, that we're always going to want to return
in functions instead of print()
, at least in code challenges (and 99.9% of the code you write anyway). You'll probably want to use .extend()
for that last challenge.

Steven Wheeler
2,330 PointsKenneth- It was more my fault for not completely understanding the wording. I extended it into its own list (rather than just returning every item) and returned that.. task done -___-. I think I need to find some projects to work on using dictionaries more.
Thanks for the help all
Steven Wheeler
2,330 PointsSteven Wheeler
2,330 PointsHi Ron,
It helps but I still haven't quite got it working. I think for every task in "dictionaries" I've asked for help on these forums! -_-
I'm getting the error "builtin_function_or_method' object has no attribute 'teachers'".
I've made a list then tried to make a "list of lists". In my eyes it should add the "item" (teacher name) followed by the length of the value (the number of classes). I'm not sure where I have gone wrong :o.