Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial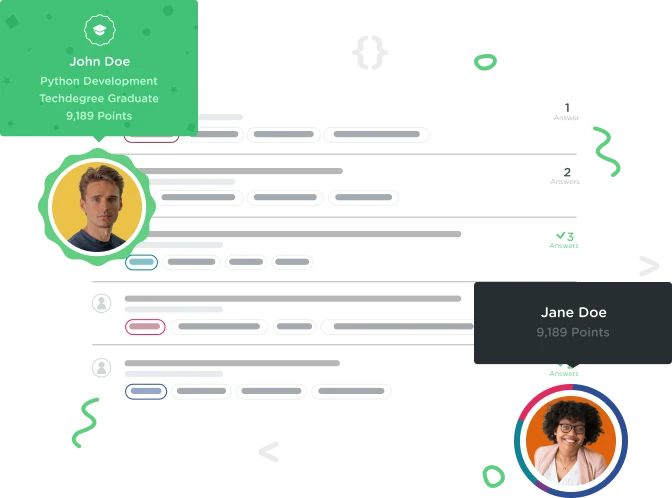
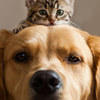
Devin Scheu
66,191 PointsHelp With Swift
Question: Create an instance of the class RoundButton and name the instance rounded.
My Code:
class Button {
var width: Double
var height: Double
init(width:Double, height:Double){
self.width = width
self.height = height
}
}
class RoundButton: Button {
var cornerRadius = 5.0
}
var rounded = RoundButton
3 Answers

Stone Preston
42,016 Pointsto create instances of classes, we use what are called initializers:
initializers are called to create a new instance of a particular type.
you need to call the init method of the RoundButton class. It doesnt override the superclass init, it just inherits it. Because of this, the init method for RoundButton is the same as Button
generally, a call to an initializer looks something like:
var newObject = ClassName()
However, the Button init method takes 2 arguments, a width and a height. Remember that initializer parameters are external by default, so you must provide the parameter name when making a call to the method
var rounded = RoundButton(width: 10, height: 10)
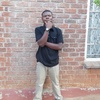
MUZ140316 Milton Chitsike
7,344 PointsThe code should be somthing like this:class Button { var width: Double var height: Double
init(width:Double, height:Double){ self.width = width self.height = height }
func incrementBy(points: Double){ width += points height += points } }
class RoundButton: Button { var cornerRadius: Double = 5.0 } var rounded = RoundButton(width: 10, height: 10)

Judith Straetemans
1,807 PointsI don't get it. Why does the subclass needs to be outside the base class brackets ?
class Button {
var width: Double
var height: Double
init(width:Double, height:Double){
self.width = width
self.height = height
class RoundButton: Button {
var cornerRadius = 5.0
}
}
}
var rounded = RoundButton(width: 10, height: 10)
Error:
swift_lint.swift:17:15: error: use of unresolved identifier 'RoundButton'
var rounded = RoundButton(width: 10, height: 10)
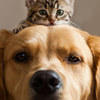
Devin Scheu
66,191 PointsRoundedButton is a subclass because it inherits the Button class, RoundedButton class being inside of the Button Class brackets doesn't necessarily mean that it's a subclass of the Button class. Move the RoundedButton class out of the Button class and beneath it and your code should pass, it should look something like this:
class Button {
var width: Double
var height: Double
init(width:Double, height:Double){
self.width = width
self.height = height
}
}
class RoundButton: Button {
var cornerRadius = 5.0
}
var rounded = RoundButton(width: 10, height: 10)
hamza shaikh
5,875 Pointshamza shaikh
5,875 Pointsi was stuck on the same problem, but i was confused because they didn't tell in a task to add a value and without it it was giving an error.