Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial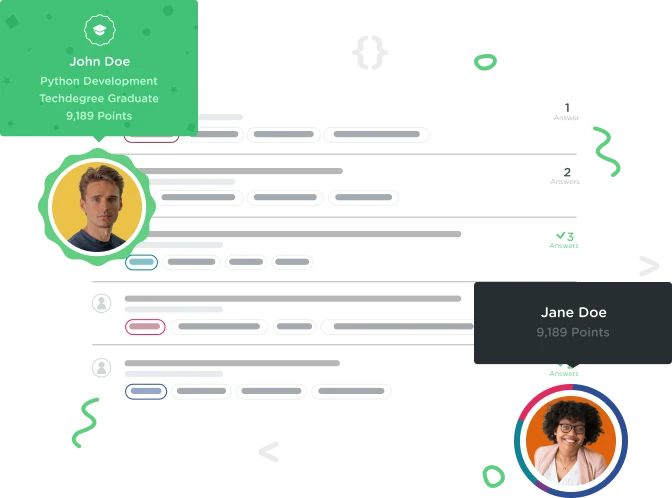

Nikhil Pandey
1,418 PointsHelp with task 1 of 3 in tuples.
I am unable to understand the concept of tuples and complete the exercise tuples.swift. The exercise is to modify the function greeting so that it includes a tuples named greeting and language. Please help
func greeting(person: String, language: String) -> (String, String) {
let language = "English"
let greeting = "Hello \(person)"
return (greeting, language)
}
3 Answers
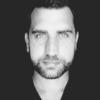
Jhoan Arango
14,575 PointsHello Nikhil Pandey :
challenge says:
Currently our greeting function only returns a single value. Modify it to return both the greeting and the language as a tuple. Make sure to name each item in the tuple: greeting and language. We will print them out in the next task.
let’s break it down
First part says: Modify it to return both the greeting and the language as a tuple.
/*
Here the function says it will return just ONE value of type String
and we need for it to say that it will return TWO values of type String.
*/
func greeting(person: String) -> String {
let language = "English"
let greeting = "Hello \(person)”
/*
We also need to modify our return statement to return two values,
meaning, a tuple.
*/
return greeting
}
Here are the modifications:
func greeting(person: String) -> (String, String) {
let language = "English"
let greeting = "Hello \(person)"
return greeting
}
Then it says: Make sure to name each item in the tuple: greeting and language.
// We give them names
func greeting(person: String) -> (greeting: String, language: String) {
let language = "English"
let greeting = "Hello \(person)"
// And modify it so that it returns both values of the tuple.
return (greeting, language)
}
Hope that clears it out for you a bit.

Nikhil Pandey
1,418 PointsHi Jhoan I am using the following in Xcode Beta 7
func greeting(person person: String, language: String) -> (greeting: String, language: String) { let language = "English" let greeting = "Hello (person)" return (greeting, language) }
greeting(person: "John", language: "Hindi")
This code is working. Also there are few changes in Swift 2, now for named parameters Swift 2 is not accepting # . Can you please help me with a bit clarity on answer you have posted versus the above code I was trying. Also request you to please attach notes at appropriate places to highlight differences in Swift 2.
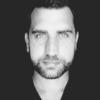
Jhoan Arango
14,575 PointsHello, I am not using swift 2, but I do know that they did eliminate the # to explicitly name parameters, but from your code, you have seem to fix that with “person person: String”.
Now the changes in swift will not affect much on the coding or the concept of tuples. Below is the code you provided me with. As you can see, you created a function called “greeting”, greeting has 2 parameters. One was named explicitly “person”, and the other one was named “language”. When you call your function, it will look something like this:
greeting(person: “Nikhil”, “English”)
// Only one parameter name appears in the calling of the function.
If you had explicitly named BOTH parameters
// Swift 2.0
func greeting(person person: String, language language: String)
// Swift 1.2
func greeting(#person: String, #language: String)
Then the calling of the function would be something like this
greeting(person: “Nikhil”, language: “English”)
// Notice how both parameters names appear in the function calling.
This is your code :
func greeting(person person: String, language: String) -> (greeting: String, language: String) {
let language = "English"
let greeting = "Hello (person)" // Fix it as such “Hello \(person)"
return (greeting, language)
}
/*
You are calling the function here, but you are adding the extra parameter
which you did not explicitly named when creating the function.
*/
greeting(person: "John", language: "Hindi”)
// This is how you should call the function
greeting(person: “John”, “Hindi”)

Nikhil Pandey
1,418 PointsHi, Just saw that in Xcode 7 beta println has been renamed to print in Swift 2. Request you to provide an updates of changelog in Swift 2 versus Swift 1.2