Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial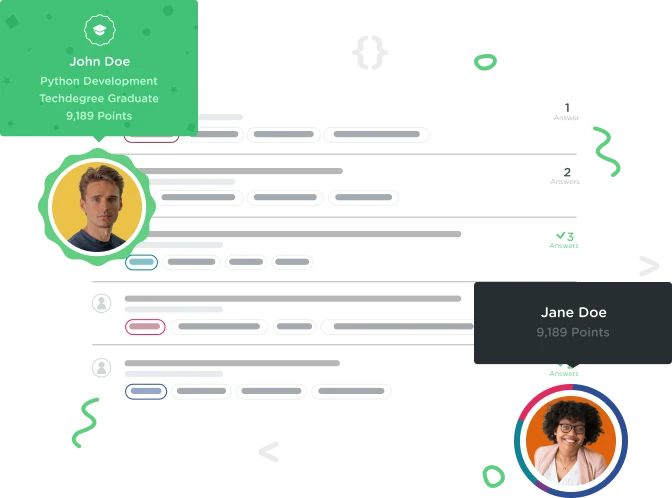

Pedro Gonzalez
2,097 PointsHelp with Task 2 of 2 in C# Dictionary
I can't find any errors wrong with my code. The error it keeps throwing out at me is: LexicalAnalysis.cs(24,12): error CS1525: Unexpected symbol `public' Compilation failed: 1 error(s), 0 warnings
using System.Collections.Generic;
namespace Treehouse.CodeChallenges
{
public class LexicalAnalysis
{
public Dictionary<string, int> WordCount = new Dictionary<string, int>();
public void AddWord(string word)
{
int count = 0;
if(WordCount.ContainsKey(word))
{
WordCount[word] = WordCount[word] + 1;
}
else if(!(WordCount.ContainsKey(word)))
{
WordCount.Add(word,count+1);
}
}
public Dictionary<string,int> WordsWithCountGreaterThan(int input) //This is the method I made for this task
{
public Dictionary<string, int> WordsGreaterThan = new Dictionary<string, int>(); ///The error is supposed to be here
foreach(KeyValuePair<string,int> word1 in WordCount)
{
if(WordCount[word1] < input)
{
WordsGreaterThan.Add(word1 , WordCount[word1]);
}
}
return WordsGreaterThan;
}
}
}
1 Answer

andren
28,558 PointsThe cause of that error is that access modifiers like public
and private
can only be used on variables when they are field variables. Declaring an access modifier for a variable defined within a method is invalid. So you can clear up that error simply by removing the public
keyword from the variable declaration.
That won't allow you to pass the challenge though as there is still a few other errors in your code. Mainly in your for loop. word1
is an object that contains a Key
property and a Value
property. You have to use those properties to pull out the key and value of the dictionary entry, you can't just pass the KeyValuePair object into the dictionary in order to pull out the value like you seem to be trying to do.
Pedro Gonzalez
2,097 PointsPedro Gonzalez
2,097 PointsThank you for your help.