Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial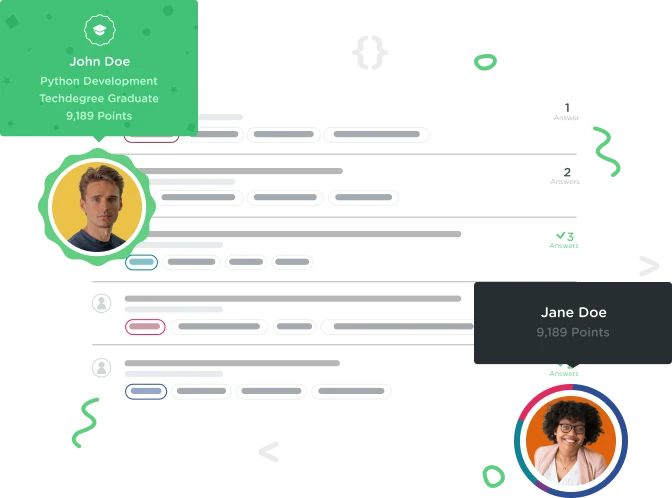
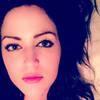
Nikki Bearman
3,389 PointsHelp with task challenge for Object-Oriented Swift 2.0 - Classes and initializer methods
Hi,
I'm having real difficulty with this challenge and was wondering if anyone could help. The task is as follows:
*In the editor you've been provided with a struct named Location that models a coordinate point using longitude and latitude values.
We want to create a class named Business. The class contains two constant stored properties: name of type String and location of type Location.
In the initializer method pass in a name and an instance of Location to set up the instance of Business. Using this initializer, create an instance and assign it to a constant named someBusiness.*
The initial code provided in the editor is as follows:
struct Location {
let latitude: Double
let longitude: Double
}
To be honest a lot of this struct and class section has gone completely over my head and I just can't seem to get it - I was hoping to get to the end and then study further elsewhere, perhaps in addition to going over the course all over again, but at least wanted to have some vague understanding and ability before completing the course. The reason I mention this is because I have no idea if what I've done so far is way off the mark or not! Here is where I've got to including the above initial code:
struct Location {
let latitude: Double
let longitude: Double
init(latitude: Double, longitude: Double) {
self.latitude = latitude
self.longitude = longitude
}
}
class Business {
let name: String
let location: Location
init(name: String, location: Location) {
self.name = name
self.location = Location(latitude: 123.4, longitude: 567.8)
}
}
let someBusiness = Business(name: "NB", location: self.location)
I'm embarrassed to say I really have no real understanding of what I'm meant to do or how right now, when up until this point it's been pretty plain sailing. I'm hoping someone could shed some light on how far off I am with what I've started with, and/or direction on what I should be doing. Any help is greatly appreciated!
Many thanks,
Nikki

Anjali Pasupathy
28,883 PointsAaron is mostly right. The only thing is, in the corrected code given, "Location" shouldn't be capitalized.
self.location = location
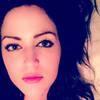
Nikki Bearman
3,389 PointsThanks so much both Aaron Bilenky & Anjali Pasupathy - relieved to hear I wasn't too far off! I guess maybe I understood a little more than I had realised.
Thanks again.

Anjali Pasupathy
28,883 PointsYou're welcome!
1 Answer

Derek Scollon
8,422 PointsYou're actually very close to the solution, it just looks like you've muddled a couple of points.
First, your Location struct all looks good. Next, your Business class init method takes two parameters, a name of type String, which you've handled correctly and a location of type Location, which should be assigned in the same way...
self.location = location
Finally, after your class, it's probably easier to understand if you create a location separately before passing it in to create someBusiness, like this...
let someLocation = Location(latitude: 123.4, longitude: 567.8)
let someBusiness = Business(name: "NB", location: someLocation)
My entire code looks like this...
struct Location {
let latitude: Double
let longitude: Double
init(latitude: Double, longitude: Double) {
self.latitude = latitude
self.longitude = longitude
}
}
class Business {
let name: String
let location: Location
init(name: String, location: Location) {
self.name = name
self.location = location
}
}
let someLocation = Location(latitude: 123.4, longitude: 567.8)
let someBusiness = Business(name: "NB", location: someLocation)
I hope that helps to clarify it for you.
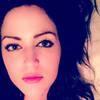
Nikki Bearman
3,389 PointsThanks ever so much for your quick help Derek Scollon - much appreciated and I've been able to complete the challenge with a little more understanding now.
All the best,
Nikki
Aaron Bilenky
13,294 PointsAaron Bilenky
13,294 PointsYou are really close. You don't want to pass in values for latitude and longitude when you are defining the init method, otherwise every Business would have the same coordinates (123.4, 567.8). If you change the second line of your init method to match the first like this
self.location = location
then you pass in the location with specific coordinates for someBusiness
let someBusiness = Business(name: "NB", location: Location(latitude: 123.4, longitude: 567.8))