Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial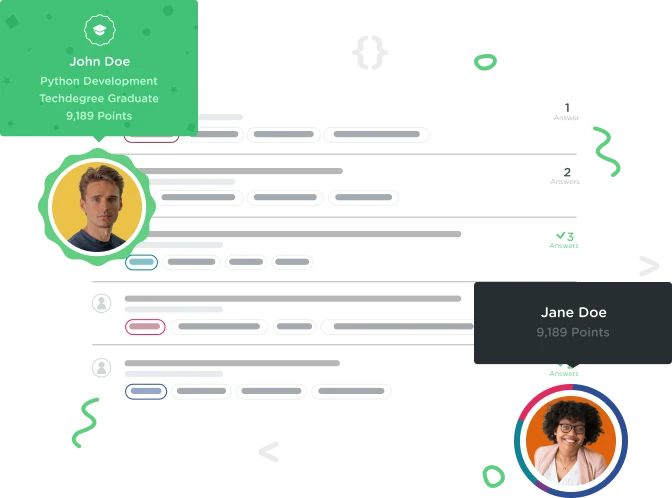

Christine Devore
Courses Plus Student 1,175 PointsHelp with Task - Set the 'robotImages' to the animation images property of 'imageView'
I am completely lost as to what they are asking for here, based on the video which changed many things in different files... I'm not sure how to adjust this code to: Set the 'robotImages' to the animation images property of 'imageView'??? :(
UIImage *image = [UIImage imageNamed:@"robot.png"];
UIImageView *imageView = [[UIImageView alloc] initWithImage:image];
/* Write your code below this line */
NSArray *robotImages = [[NSArray alloc] initWithObjects:[UIImage imageNamed:@"Robot1"],
[UIImage imageNamed:@"Robot2"],
[UIImage imageNamed:@"Robot3"],
[UIImage imageNamed:@"Robot4"],nil];
12 Answers
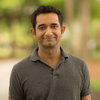
Amit Bijlani
Treehouse Guest Teacher@sven Christine got it right by declaring the robotImages
instance variable. The next step after that is to simply assign the robotImages
to the animationImages
property as you did without self
. You would use self
only if imageView
was a property of a class which in this case it is not. In the video imageView
is a property of the view controller that is why we use self
.
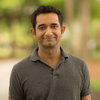
Amit Bijlani
Treehouse Guest TeacherYou are almost there. You need to allocate and initialize a new array and set it to robotImages
. Then set the robotImages
to imageView.animationImages
.

Chris Mitchell-Clare
1,270 PointsStill not solved, I am caught up on the same issue.
Set the animation images property of 'imageView' to 'robotImages'. Important: The code you write in each task should be added to the code written in the previous task. Recheck Work Bummer! Make sure you create an NSArray named 'robotImages' with instances of UIImage objects.
ViewController.m
1 UIImage *image = [UIImage imageNamed:@"robot.png"]; 2 UIImageView *imageView = [[UIImageView alloc] initWithImage:image]; 3
4 /* Write your code below this line */ 5 imageView.robotImages = [[NSArray alloc] initWithObjects:[UIImage imageNamed:@"Robot1"], 6 [UIImage imageNamed:@"Robot2"], 7 [UIImage imageNamed:@"Robot3"], 8 [UIImage imageNamed:@"Robot4"], nil]; 9
10 self.imageView.animationImages = robotImages;
What am I doing wrong?????? There is no Tips along the way if you make errors, and I am stuck, cannot progress any further, this one Code Challenge is really putting me off as nothing seems to be accepted and the video isn't very insightful when the Code Challenge Question doesn't even specifically match the code I am looking at in xCode.
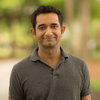
Amit Bijlani
Treehouse Guest TeacherSorry you have issues but that's the goal of the code challenge to help you think above and beyond what was taught in the video. Here are some issues with your code:
robotImages
is not a property ofimageView
. It should be declared as an instance variable. Which means that you would be declaring something likeNSArray *robotImages = ....
Once you create a
robotImages
instance variable then you can set that to theanimationImages
property ofimageView
.You cannot not use
self
because there are no properties declared using@property
. They are all instance variables.

Chris Mitchell-Clare
1,270 PointsHaha. Oh, thanks. Got it.

Ryan Carson
23,287 PointsHey Christine,
Thanks for posting this question. I'll ping Amit and ask him to chime in. We appreciate you being a Treehouse Student! :)
Ryan
Founder/CEO Treehouse
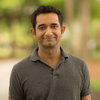
Amit Bijlani
Treehouse Guest Teacher@Christine UIImageView
has a property called animationImages
. In this case, you would refer to it as imageView.animationImages
. You can review the video at 5:50 mark where we set the array to the animationImages
property.

sven tillack
610 PointsI'm quite hanging there, too.
imageView.robotImages = [[NSArray alloc] initWithObjects:[UIImage
imageNamed:@"Robot1"],
[UIImage imageNamed:@"Robot2"],
[UIImage imageNamed:@"Robot3"],
[UIImage imageNamed:@"Robot4"], nil];
I understand that robotImages is incorrect at that point because animationImages is kind of a fixed statement there, but I dont know where to put it :(
Searching the web I thought
self.imageView.animationImages = robotImages;
might help, but it doesnt.
Its always quite a trial and error for me, but your courses are great fun, though!

sven tillack
610 PointsGreat, it worked for me! Thanks! Hopefully Christine got through, too!

Christine Devore
Courses Plus Student 1,175 PointsThank you so much ~ Got it!!! Glad you did too Sven!!!

David Jacobus
2,833 PointsI am stuck on this step. Amit, I do not understand when you say,"The next step after that is to simply assign the robotImages to the animationImages property". I am confused to where I need to put the imageView.animationImages property.
Here is my code:
imageView.animationImages *robotImages = [[NSArray alloc] initWithObjects: [UIImage imageNamed:@"Robot1"],[UIImage imageNamed:@"Robot2"], [UIImage imageNamed:@"Robot3"], [UIImage imageNamed:@"Robot4"],nil];
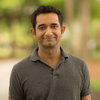
Amit Bijlani
Treehouse Guest TeacherDavid Jacobs your code is right except that you first need to declare *robotImages
and then set it to imageView.animationImages
.
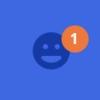
x124441249481294124
2,492 PointsHello Amit I'm stuck on the same spot and do not really understand what you mean by "declare *robotImages", I've tried everything I could think of and now hope you can help me.
Thank you very much.
Best regards, Fabio
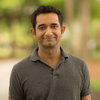
Amit Bijlani
Treehouse Guest TeacherI mean create an instance variable named robotImages
of the class NSArray
.
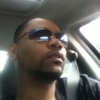
DeShawn Pellebon
Courses Plus Student 1,350 PointsI'm stuck at this point and would really appreciate any feed back on what i am doing wrong thanks.
NSArray*robotImages; imageView.animationImages*robotImages =[[NSArray alloc ]initWithObjects: [UIImage imageNamed:@"Robot1"],[UIImage imageNamed: @"Robot2"], [UIImage imageNamed: @"Robot3"],[UIImage imageNamed: @"Robot4"], nil];

Adam Peterka
9,626 PointsI am having difficulties with this code challenge as well. Here is what i have:
NSArray *robotImages = imageView.animationImages;
imageView *robotImages = [[NSArray alloc] initWithObjects:[UIImage imageNamed:@"Robot1"],[UIImage imageNamed:@"Robot2"],[UIImage imageNamed:@"Robot3"],[UIImage imageNamed:@"Robot4"],nil];
i think my first statement is wrong and second one is correct. Not sure what i need to change.
Thanks.
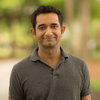
Amit Bijlani
Treehouse Guest TeacherUnfortunately, your statements are mixed up. When you are declaring an array the left and right side of your statements must equate to allocating and initializing a new array, which would be the left side of the first line and right side of your second line.
NSArray *robotImages = [[NSArray alloc] initWithObjects:[UIImage imageNamed:@"Robot1"],[UIImage imageNamed:@"Robot2"],[UIImage imageNamed:@"Robot3"],[UIImage imageNamed:@"Robot4"],nil];
Once you have created that array (as shown above) then it should be assigned to the animationImages
property of imageView
, which means that imageView.animationImages
is on the left side and robotImages
is on the right.

Adam Peterka
9,626 PointsThanks Amit. I understand now!
Joseph Finkenbinder
5,340 PointsJoseph Finkenbinder
5,340 PointsBut where do you put the
imageView.animationImages
line of code? I've read all the posts here a few times and it's not sinking in where to put this stuff. I'm really struggling with the terminology and nomenclature of Obj-C.NSArray *robotImages = [[NSArray alloc] initWithObjects:[UIImage:@"Robot1"], [UIImage:@"Robot2"], [UIImage:@"Robot3"], [UIImage:@"Robot4"], nil];
What do I need to add to this code to get through the challenge? Specifically, 'where' do I add it?
Amit Bijlani
Treehouse Guest TeacherAmit Bijlani
Treehouse Guest TeacherYou've got the two statements now all you have to do is assign
robotImages
toimageView.animationImages
.Joseph Finkenbinder
5,340 PointsJoseph Finkenbinder
5,340 PointsWhere do I put that into the code? I tried to put it in the beginning but that didn't work.
/* Write your code below this line */ imageView.animationImages *robotImages = [[NSArray alloc] initWithObjects:[UIImage:@"Robot1"], [UIImage:@"Robot2"], [UIImage:@"Robot3"], [UIImage:@"Robot4"], nil];
Amit Bijlani
Treehouse Guest TeacherAmit Bijlani
Treehouse Guest TeacherIn the first line you define the array:
Next line you assign the
robotImages
instance variable toanimationImages
imageView.animationImages = robotImages;
Joseph Finkenbinder
5,340 PointsJoseph Finkenbinder
5,340 PointsThanks. I picked up a Big Nerd Ranch objec C book to help out a bit.
Mike Mitchell
Courses Plus Student 27,026 PointsMike Mitchell
Courses Plus Student 27,026 PointsWow. Brain slowly turning to soup...
So in our project, we flipped it from what we see in the challenge. We created and synthesized the imageView array and then assigned the animationImages to imageView using all of the crystal ball pics.
In the challenge, we need to define an array and then assign it an instance variable?
I couldn't even begin to tell you if I think I'm making sense.
... sleep(5);
Amit Bijlani
Treehouse Guest TeacherAmit Bijlani
Treehouse Guest TeacherMike Mitchell You got it. Spot on!