Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial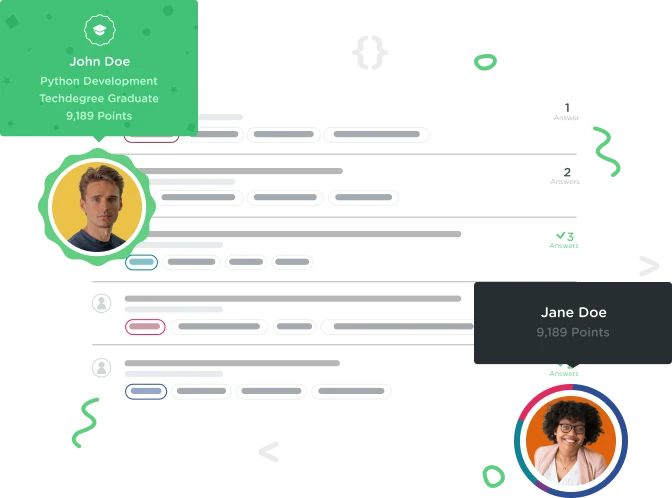

Frank Peer
4,780 PointsHelp with the challenge: Delivering the MVP, Refactoring.
I'm hoping that people that have already taken this challenge can steer me in the right direction. The instructions are to make the code easier to add items to the cart, and to make the existing entries for cart.addItem work. But is this supposed to be carried out through refactoring, using method signatures, writing brand new code, or all of the above? My code is the first section under the comment " // Here's what..." but I think I'm going in the wrong direction.
public class Example {
public static void main(String[] args) {
ShoppingCart cart = new ShoppingCart();
//Here's what I think the answer might look like
String item = console.readLine("Enter an item name: ");
int quantity = console.readLine("Enter an item quantity: ");
cart.addItem(item, quantity);
Product pez = new Product("Cherry PEZ refill (12 pieces)");
cart.addItem(pez, 5);
/* Since a quantity of 1 is such a common argument when adding a product to the cart,
* your fellow developers have asked you to make the following code work, as well as keeping
* the ability to add a product and a quantity.
*/
Product dispenser = new Product("Yoda PEZ dispenser");
cart.addItem(dispenser, 1);
}
}
public class ShoppingCart {
public void addItem(Product item, int quantity) {
System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
/* Other code omitted for clarity */
}
}
public class Product {
/* Other code omitted for clarity, but you could imagine
it would store price, options like size and color
*/
private String mName;
public Product(String name) {
mName = name;
}
public String getName() {
return mName;
}
}
2 Answers
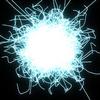
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsHello, I was very confused by this when I first did it as well. What they're asking you to do is create a new method for product to be added in the event that end user does not signify a quanity, since we already have one work addItem method it shouldn't be too difficult, I just ended up over thinking the problem.
Here's a step by step breakdown of what needs to happen to pass the challange.
1) Create a new public void addItem method.
2) make it take only a Product Item like it's original method, but make this one that it does not require a quantity.
3) in the newly constructed method body use the already defined body to add 1 of the items in the cart if the user only passed a value of add item.
4) be sure to uncomment the line cart.addItem(dispenser, 1); in the example class, but it looks like you were already on top of that part.
It should look something like this when you are done.
public class ShoppingCart {
public void addItem(Product item, int quantity) {
System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
/* Other code omitted for clarity */
}
public void addItem(Product item) {
addItem(item, 1);
}
}
Thanks, I hope this helps. Let me know if not and I'll provide any further clarification I can.

Jess Sanders
12,086 PointsHere's my simplest explanation of what's going on in the 'defaulting parameters' code challenge:
Example.java has a line of code that is commented out
cart.addItem(dispenser);
If all you do is uncomment this line and check your work, you get a compiler error. We need to write a second addItem method that only takes one argument, rather than two.
So, in ShoppingCart.java, we can copy and paste the existing addItem method, but remove the second argument. We also need to change what is printed, because we know that we are "Adding 1 of" of the item to the cart.
Frank Peer
4,780 PointsFrank Peer
4,780 PointsSo, with this code in effect, if the user just types in a product name and doesn't specify a quantity, the code will automatically add a quantity of 1 to the cart? Was that the goal?
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsRob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsYes, that is absolutely correct, all that this challenge wanted you to do is make the method more user friendly, so that if someone just wanted to add an item and not enter a quantity instead of throwing an irritating error message it just told the program to assume that the user meant 1.